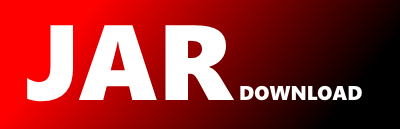
com.pulumi.awsnative.securityhub.kotlin.AutomationRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.securityhub.kotlin
import com.pulumi.awsnative.securityhub.AutomationRuleArgs.builder
import com.pulumi.awsnative.securityhub.kotlin.enums.AutomationRuleRuleStatus
import com.pulumi.awsnative.securityhub.kotlin.inputs.AutomationRulesActionArgs
import com.pulumi.awsnative.securityhub.kotlin.inputs.AutomationRulesActionArgsBuilder
import com.pulumi.awsnative.securityhub.kotlin.inputs.AutomationRulesFindingFiltersArgs
import com.pulumi.awsnative.securityhub.kotlin.inputs.AutomationRulesFindingFiltersArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The ``AWS::SecurityHub::AutomationRule`` resource specifies an automation rule based on input parameters. For more information, see [Automation rules](https://docs.aws.amazon.com/securityhub/latest/userguide/automation-rules.html) in the *User Guide*.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* @property actions One or more actions to update finding fields if a finding matches the conditions specified in `Criteria` .
* @property criteria A set of [Security Finding Format (ASFF)](https://docs.aws.amazon.com/securityhub/latest/userguide/securityhub-findings-format.html) finding field attributes and corresponding expected values that ASH uses to filter findings. If a rule is enabled and a finding matches the criteria specified in this parameter, ASH applies the rule action to the finding.
* @property description A description of the rule.
* @property isTerminal Specifies whether a rule is the last to be applied with respect to a finding that matches the rule criteria. This is useful when a finding matches the criteria for multiple rules, and each rule has different actions. If a rule is terminal, Security Hub applies the rule action to a finding that matches the rule criteria and doesn't evaluate other rules for the finding. By default, a rule isn't terminal.
* @property ruleName The name of the rule.
* @property ruleOrder An integer ranging from 1 to 1000 that represents the order in which the rule action is applied to findings. Security Hub applies rules with lower values for this parameter first.
* @property ruleStatus Whether the rule is active after it is created. If this parameter is equal to ``ENABLED``, ASH applies the rule to findings and finding updates after the rule is created.
* @property tags User-defined tags associated with an automation rule.
*/
public data class AutomationRuleArgs(
public val actions: Output>? = null,
public val criteria: Output? = null,
public val description: Output? = null,
public val isTerminal: Output? = null,
public val ruleName: Output? = null,
public val ruleOrder: Output? = null,
public val ruleStatus: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy