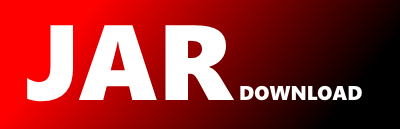
com.pulumi.awsnative.securityhub.kotlin.PolicyAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.securityhub.kotlin
import com.pulumi.awsnative.securityhub.PolicyAssociationArgs.builder
import com.pulumi.awsnative.securityhub.kotlin.enums.PolicyAssociationTargetType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The AWS::SecurityHub::PolicyAssociation resource represents the AWS Security Hub Central Configuration Policy associations in your Target. Only the AWS Security Hub delegated administrator can create the resouce from the home region.
* @property configurationPolicyId The universally unique identifier (UUID) of the configuration policy or a value of SELF_MANAGED_SECURITY_HUB for a self-managed configuration
* @property targetId The identifier of the target account, organizational unit, or the root
* @property targetType Indicates whether the target is an AWS account, organizational unit, or the organization root
*/
public data class PolicyAssociationArgs(
public val configurationPolicyId: Output? = null,
public val targetId: Output? = null,
public val targetType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.securityhub.PolicyAssociationArgs =
com.pulumi.awsnative.securityhub.PolicyAssociationArgs.builder()
.configurationPolicyId(configurationPolicyId?.applyValue({ args0 -> args0 }))
.targetId(targetId?.applyValue({ args0 -> args0 }))
.targetType(targetType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PolicyAssociationArgs].
*/
@PulumiTagMarker
public class PolicyAssociationArgsBuilder internal constructor() {
private var configurationPolicyId: Output? = null
private var targetId: Output? = null
private var targetType: Output? = null
/**
* @param value The universally unique identifier (UUID) of the configuration policy or a value of SELF_MANAGED_SECURITY_HUB for a self-managed configuration
*/
@JvmName("mysuvgyafuxmsjvx")
public suspend fun configurationPolicyId(`value`: Output) {
this.configurationPolicyId = value
}
/**
* @param value The identifier of the target account, organizational unit, or the root
*/
@JvmName("bnjdipcnvfbnakjx")
public suspend fun targetId(`value`: Output) {
this.targetId = value
}
/**
* @param value Indicates whether the target is an AWS account, organizational unit, or the organization root
*/
@JvmName("hyqxijwubeulpytv")
public suspend fun targetType(`value`: Output) {
this.targetType = value
}
/**
* @param value The universally unique identifier (UUID) of the configuration policy or a value of SELF_MANAGED_SECURITY_HUB for a self-managed configuration
*/
@JvmName("xmnxijlxfwgxxafw")
public suspend fun configurationPolicyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configurationPolicyId = mapped
}
/**
* @param value The identifier of the target account, organizational unit, or the root
*/
@JvmName("gvvypgjngheesoab")
public suspend fun targetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetId = mapped
}
/**
* @param value Indicates whether the target is an AWS account, organizational unit, or the organization root
*/
@JvmName("bkmqkuogekqigwwc")
public suspend fun targetType(`value`: PolicyAssociationTargetType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetType = mapped
}
internal fun build(): PolicyAssociationArgs = PolicyAssociationArgs(
configurationPolicyId = configurationPolicyId,
targetId = targetId,
targetType = targetType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy