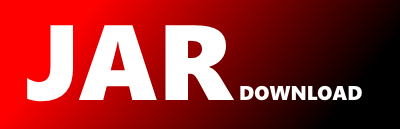
com.pulumi.awsnative.ses.kotlin.ConfigurationSet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ses.kotlin
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetDeliveryOptions
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetReputationOptions
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetSendingOptions
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetSuppressionOptions
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetTrackingOptions
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetVdmOptions
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetDeliveryOptions.Companion.toKotlin as configurationSetDeliveryOptionsToKotlin
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetReputationOptions.Companion.toKotlin as configurationSetReputationOptionsToKotlin
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetSendingOptions.Companion.toKotlin as configurationSetSendingOptionsToKotlin
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetSuppressionOptions.Companion.toKotlin as configurationSetSuppressionOptionsToKotlin
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetTrackingOptions.Companion.toKotlin as configurationSetTrackingOptionsToKotlin
import com.pulumi.awsnative.ses.kotlin.outputs.ConfigurationSetVdmOptions.Companion.toKotlin as configurationSetVdmOptionsToKotlin
/**
* Builder for [ConfigurationSet].
*/
@PulumiTagMarker
public class ConfigurationSetResourceBuilder internal constructor() {
public var name: String? = null
public var args: ConfigurationSetArgs = ConfigurationSetArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ConfigurationSetArgsBuilder.() -> Unit) {
val builder = ConfigurationSetArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ConfigurationSet {
val builtJavaResource = com.pulumi.awsnative.ses.ConfigurationSet(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ConfigurationSet(builtJavaResource)
}
}
/**
* Resource schema for AWS::SES::ConfigurationSet.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
*/
public class ConfigurationSet internal constructor(
override val javaResource: com.pulumi.awsnative.ses.ConfigurationSet,
) : KotlinCustomResource(javaResource, ConfigurationSetMapper) {
/**
* Specifies the name of the dedicated IP pool to associate with the configuration set and whether messages that use the configuration set are required to use Transport Layer Security (TLS).
*/
public val deliveryOptions: Output?
get() = javaResource.deliveryOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> configurationSetDeliveryOptionsToKotlin(args0) })
}).orElse(null)
})
/**
* The name of the configuration set.
*/
public val name: Output?
get() = javaResource.name().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* An object that defines whether or not Amazon SES collects reputation metrics for the emails that you send that use the configuration set.
*/
public val reputationOptions: Output?
get() = javaResource.reputationOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> configurationSetReputationOptionsToKotlin(args0) })
}).orElse(null)
})
/**
* An object that defines whether or not Amazon SES can send email that you send using the configuration set.
*/
public val sendingOptions: Output?
get() = javaResource.sendingOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> configurationSetSendingOptionsToKotlin(args0) })
}).orElse(null)
})
/**
* An object that contains information about the suppression list preferences for your account.
*/
public val suppressionOptions: Output?
get() = javaResource.suppressionOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> configurationSetSuppressionOptionsToKotlin(args0) })
}).orElse(null)
})
/**
* An object that defines the open and click tracking options for emails that you send using the configuration set.
*/
public val trackingOptions: Output?
get() = javaResource.trackingOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> configurationSetTrackingOptionsToKotlin(args0) })
}).orElse(null)
})
/**
* The Virtual Deliverability Manager (VDM) options that apply to the configuration set.
*/
public val vdmOptions: Output?
get() = javaResource.vdmOptions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
configurationSetVdmOptionsToKotlin(args0)
})
}).orElse(null)
})
}
public object ConfigurationSetMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.ses.ConfigurationSet::class == javaResource::class
override fun map(javaResource: Resource): ConfigurationSet = ConfigurationSet(
javaResource as
com.pulumi.awsnative.ses.ConfigurationSet,
)
}
/**
* @see [ConfigurationSet].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ConfigurationSet].
*/
public suspend fun configurationSet(
name: String,
block: suspend ConfigurationSetResourceBuilder.() -> Unit,
): ConfigurationSet {
val builder = ConfigurationSetResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ConfigurationSet].
* @param name The _unique_ name of the resulting resource.
*/
public fun configurationSet(name: String): ConfigurationSet {
val builder = ConfigurationSetResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy