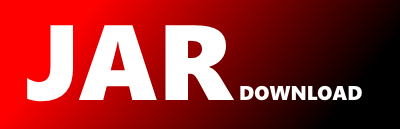
com.pulumi.awsnative.shield.kotlin.ShieldFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.shield.kotlin
import com.pulumi.awsnative.shield.ShieldFunctions.getDrtAccessPlain
import com.pulumi.awsnative.shield.ShieldFunctions.getProactiveEngagementPlain
import com.pulumi.awsnative.shield.ShieldFunctions.getProtectionGroupPlain
import com.pulumi.awsnative.shield.ShieldFunctions.getProtectionPlain
import com.pulumi.awsnative.shield.kotlin.inputs.GetDrtAccessPlainArgs
import com.pulumi.awsnative.shield.kotlin.inputs.GetDrtAccessPlainArgsBuilder
import com.pulumi.awsnative.shield.kotlin.inputs.GetProactiveEngagementPlainArgs
import com.pulumi.awsnative.shield.kotlin.inputs.GetProactiveEngagementPlainArgsBuilder
import com.pulumi.awsnative.shield.kotlin.inputs.GetProtectionGroupPlainArgs
import com.pulumi.awsnative.shield.kotlin.inputs.GetProtectionGroupPlainArgsBuilder
import com.pulumi.awsnative.shield.kotlin.inputs.GetProtectionPlainArgs
import com.pulumi.awsnative.shield.kotlin.inputs.GetProtectionPlainArgsBuilder
import com.pulumi.awsnative.shield.kotlin.outputs.GetDrtAccessResult
import com.pulumi.awsnative.shield.kotlin.outputs.GetProactiveEngagementResult
import com.pulumi.awsnative.shield.kotlin.outputs.GetProtectionGroupResult
import com.pulumi.awsnative.shield.kotlin.outputs.GetProtectionResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.shield.kotlin.outputs.GetDrtAccessResult.Companion.toKotlin as getDrtAccessResultToKotlin
import com.pulumi.awsnative.shield.kotlin.outputs.GetProactiveEngagementResult.Companion.toKotlin as getProactiveEngagementResultToKotlin
import com.pulumi.awsnative.shield.kotlin.outputs.GetProtectionGroupResult.Companion.toKotlin as getProtectionGroupResultToKotlin
import com.pulumi.awsnative.shield.kotlin.outputs.GetProtectionResult.Companion.toKotlin as getProtectionResultToKotlin
public object ShieldFunctions {
/**
* Config the role and list of Amazon S3 log buckets used by the Shield Response Team (SRT) to access your AWS account while assisting with attack mitigation.
* @param argument null
* @return null
*/
public suspend fun getDrtAccess(argument: GetDrtAccessPlainArgs): GetDrtAccessResult =
getDrtAccessResultToKotlin(getDrtAccessPlain(argument.toJava()).await())
/**
* @see [getDrtAccess].
* @param accountId The ID of the account that submitted the template.
* @return null
*/
public suspend fun getDrtAccess(accountId: String): GetDrtAccessResult {
val argument = GetDrtAccessPlainArgs(
accountId = accountId,
)
return getDrtAccessResultToKotlin(getDrtAccessPlain(argument.toJava()).await())
}
/**
* @see [getDrtAccess].
* @param argument Builder for [com.pulumi.awsnative.shield.kotlin.inputs.GetDrtAccessPlainArgs].
* @return null
*/
public suspend fun getDrtAccess(argument: suspend GetDrtAccessPlainArgsBuilder.() -> Unit): GetDrtAccessResult {
val builder = GetDrtAccessPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDrtAccessResultToKotlin(getDrtAccessPlain(builtArgument.toJava()).await())
}
/**
* Authorizes the Shield Response Team (SRT) to use email and phone to notify contacts about escalations to the SRT and to initiate proactive customer support.
* @param argument null
* @return null
*/
public suspend fun getProactiveEngagement(argument: GetProactiveEngagementPlainArgs): GetProactiveEngagementResult =
getProactiveEngagementResultToKotlin(getProactiveEngagementPlain(argument.toJava()).await())
/**
* @see [getProactiveEngagement].
* @param accountId The ID of the account that submitted the template.
* @return null
*/
public suspend fun getProactiveEngagement(accountId: String): GetProactiveEngagementResult {
val argument = GetProactiveEngagementPlainArgs(
accountId = accountId,
)
return getProactiveEngagementResultToKotlin(getProactiveEngagementPlain(argument.toJava()).await())
}
/**
* @see [getProactiveEngagement].
* @param argument Builder for [com.pulumi.awsnative.shield.kotlin.inputs.GetProactiveEngagementPlainArgs].
* @return null
*/
public suspend fun getProactiveEngagement(argument: suspend GetProactiveEngagementPlainArgsBuilder.() -> Unit): GetProactiveEngagementResult {
val builder = GetProactiveEngagementPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProactiveEngagementResultToKotlin(getProactiveEngagementPlain(builtArgument.toJava()).await())
}
/**
* Enables AWS Shield Advanced for a specific AWS resource. The resource can be an Amazon CloudFront distribution, Amazon Route 53 hosted zone, AWS Global Accelerator standard accelerator, Elastic IP Address, Application Load Balancer, or a Classic Load Balancer. You can protect Amazon EC2 instances and Network Load Balancers by association with protected Amazon EC2 Elastic IP addresses.
* @param argument null
* @return null
*/
public suspend fun getProtection(argument: GetProtectionPlainArgs): GetProtectionResult =
getProtectionResultToKotlin(getProtectionPlain(argument.toJava()).await())
/**
* @see [getProtection].
* @param protectionArn The ARN (Amazon Resource Name) of the protection.
* @return null
*/
public suspend fun getProtection(protectionArn: String): GetProtectionResult {
val argument = GetProtectionPlainArgs(
protectionArn = protectionArn,
)
return getProtectionResultToKotlin(getProtectionPlain(argument.toJava()).await())
}
/**
* @see [getProtection].
* @param argument Builder for [com.pulumi.awsnative.shield.kotlin.inputs.GetProtectionPlainArgs].
* @return null
*/
public suspend fun getProtection(argument: suspend GetProtectionPlainArgsBuilder.() -> Unit): GetProtectionResult {
val builder = GetProtectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProtectionResultToKotlin(getProtectionPlain(builtArgument.toJava()).await())
}
/**
* A grouping of protected resources so they can be handled as a collective. This resource grouping improves the accuracy of detection and reduces false positives.
* @param argument null
* @return null
*/
public suspend fun getProtectionGroup(argument: GetProtectionGroupPlainArgs): GetProtectionGroupResult =
getProtectionGroupResultToKotlin(getProtectionGroupPlain(argument.toJava()).await())
/**
* @see [getProtectionGroup].
* @param protectionGroupArn The ARN (Amazon Resource Name) of the protection group.
* @return null
*/
public suspend fun getProtectionGroup(protectionGroupArn: String): GetProtectionGroupResult {
val argument = GetProtectionGroupPlainArgs(
protectionGroupArn = protectionGroupArn,
)
return getProtectionGroupResultToKotlin(getProtectionGroupPlain(argument.toJava()).await())
}
/**
* @see [getProtectionGroup].
* @param argument Builder for [com.pulumi.awsnative.shield.kotlin.inputs.GetProtectionGroupPlainArgs].
* @return null
*/
public suspend fun getProtectionGroup(argument: suspend GetProtectionGroupPlainArgsBuilder.() -> Unit): GetProtectionGroupResult {
val builder = GetProtectionGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProtectionGroupResultToKotlin(getProtectionGroupPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy