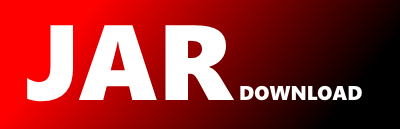
com.pulumi.awsnative.transfer.kotlin.inputs.As2ConfigPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.transfer.kotlin.inputs
import com.pulumi.awsnative.transfer.inputs.As2ConfigPropertiesArgs.builder
import com.pulumi.awsnative.transfer.kotlin.enums.ConnectorAs2ConfigPropertiesCompression
import com.pulumi.awsnative.transfer.kotlin.enums.ConnectorAs2ConfigPropertiesEncryptionAlgorithm
import com.pulumi.awsnative.transfer.kotlin.enums.ConnectorAs2ConfigPropertiesMdnResponse
import com.pulumi.awsnative.transfer.kotlin.enums.ConnectorAs2ConfigPropertiesMdnSigningAlgorithm
import com.pulumi.awsnative.transfer.kotlin.enums.ConnectorAs2ConfigPropertiesSigningAlgorithm
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Configuration for an AS2 connector.
* @property basicAuthSecretId ARN or name of the secret in AWS Secrets Manager which contains the credentials for Basic authentication. If empty, Basic authentication is disabled for the AS2 connector
* @property compression Compression setting for this AS2 connector configuration.
* @property encryptionAlgorithm Encryption algorithm for this AS2 connector configuration.
* @property localProfileId A unique identifier for the local profile.
* @property mdnResponse MDN Response setting for this AS2 connector configuration.
* @property mdnSigningAlgorithm MDN Signing algorithm for this AS2 connector configuration.
* @property messageSubject The message subject for this AS2 connector configuration.
* @property partnerProfileId A unique identifier for the partner profile.
* @property signingAlgorithm Signing algorithm for this AS2 connector configuration.
*/
public data class As2ConfigPropertiesArgs(
public val basicAuthSecretId: Output? = null,
public val compression: Output? = null,
public val encryptionAlgorithm: Output? = null,
public val localProfileId: Output? = null,
public val mdnResponse: Output? = null,
public val mdnSigningAlgorithm: Output? = null,
public val messageSubject: Output? = null,
public val partnerProfileId: Output? = null,
public val signingAlgorithm: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.transfer.inputs.As2ConfigPropertiesArgs =
com.pulumi.awsnative.transfer.inputs.As2ConfigPropertiesArgs.builder()
.basicAuthSecretId(basicAuthSecretId?.applyValue({ args0 -> args0 }))
.compression(compression?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.encryptionAlgorithm(
encryptionAlgorithm?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.localProfileId(localProfileId?.applyValue({ args0 -> args0 }))
.mdnResponse(mdnResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.mdnSigningAlgorithm(
mdnSigningAlgorithm?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.messageSubject(messageSubject?.applyValue({ args0 -> args0 }))
.partnerProfileId(partnerProfileId?.applyValue({ args0 -> args0 }))
.signingAlgorithm(
signingAlgorithm?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [As2ConfigPropertiesArgs].
*/
@PulumiTagMarker
public class As2ConfigPropertiesArgsBuilder internal constructor() {
private var basicAuthSecretId: Output? = null
private var compression: Output? = null
private var encryptionAlgorithm: Output? = null
private var localProfileId: Output? = null
private var mdnResponse: Output? = null
private var mdnSigningAlgorithm: Output? = null
private var messageSubject: Output? = null
private var partnerProfileId: Output? = null
private var signingAlgorithm: Output? = null
/**
* @param value ARN or name of the secret in AWS Secrets Manager which contains the credentials for Basic authentication. If empty, Basic authentication is disabled for the AS2 connector
*/
@JvmName("mngtuxlkxwgjqylo")
public suspend fun basicAuthSecretId(`value`: Output) {
this.basicAuthSecretId = value
}
/**
* @param value Compression setting for this AS2 connector configuration.
*/
@JvmName("mfmjgxukhtudcxiq")
public suspend fun compression(`value`: Output) {
this.compression = value
}
/**
* @param value Encryption algorithm for this AS2 connector configuration.
*/
@JvmName("fllxucnxufplswdr")
public suspend fun encryptionAlgorithm(`value`: Output) {
this.encryptionAlgorithm = value
}
/**
* @param value A unique identifier for the local profile.
*/
@JvmName("qtkuifsmbiksrpqm")
public suspend fun localProfileId(`value`: Output) {
this.localProfileId = value
}
/**
* @param value MDN Response setting for this AS2 connector configuration.
*/
@JvmName("tdbjggppbovoxtfx")
public suspend fun mdnResponse(`value`: Output) {
this.mdnResponse = value
}
/**
* @param value MDN Signing algorithm for this AS2 connector configuration.
*/
@JvmName("fbpqgddglushlyqm")
public suspend fun mdnSigningAlgorithm(`value`: Output) {
this.mdnSigningAlgorithm = value
}
/**
* @param value The message subject for this AS2 connector configuration.
*/
@JvmName("ibumuomyynpobbnd")
public suspend fun messageSubject(`value`: Output) {
this.messageSubject = value
}
/**
* @param value A unique identifier for the partner profile.
*/
@JvmName("blmekwmrnkwekfba")
public suspend fun partnerProfileId(`value`: Output) {
this.partnerProfileId = value
}
/**
* @param value Signing algorithm for this AS2 connector configuration.
*/
@JvmName("ybkfgkflopqhgtmi")
public suspend fun signingAlgorithm(`value`: Output) {
this.signingAlgorithm = value
}
/**
* @param value ARN or name of the secret in AWS Secrets Manager which contains the credentials for Basic authentication. If empty, Basic authentication is disabled for the AS2 connector
*/
@JvmName("qqhbjejqlwfucwmj")
public suspend fun basicAuthSecretId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.basicAuthSecretId = mapped
}
/**
* @param value Compression setting for this AS2 connector configuration.
*/
@JvmName("mjbiykhahxfptees")
public suspend fun compression(`value`: ConnectorAs2ConfigPropertiesCompression?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.compression = mapped
}
/**
* @param value Encryption algorithm for this AS2 connector configuration.
*/
@JvmName("llrtvtnsnwoabwap")
public suspend fun encryptionAlgorithm(`value`: ConnectorAs2ConfigPropertiesEncryptionAlgorithm?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionAlgorithm = mapped
}
/**
* @param value A unique identifier for the local profile.
*/
@JvmName("jgjjcarvahsnhull")
public suspend fun localProfileId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.localProfileId = mapped
}
/**
* @param value MDN Response setting for this AS2 connector configuration.
*/
@JvmName("ytioocgjvksamofs")
public suspend fun mdnResponse(`value`: ConnectorAs2ConfigPropertiesMdnResponse?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mdnResponse = mapped
}
/**
* @param value MDN Signing algorithm for this AS2 connector configuration.
*/
@JvmName("mukgygyygcfccunq")
public suspend fun mdnSigningAlgorithm(`value`: ConnectorAs2ConfigPropertiesMdnSigningAlgorithm?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mdnSigningAlgorithm = mapped
}
/**
* @param value The message subject for this AS2 connector configuration.
*/
@JvmName("ssfukalkfrxwiogh")
public suspend fun messageSubject(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.messageSubject = mapped
}
/**
* @param value A unique identifier for the partner profile.
*/
@JvmName("aloutctyggkgrryo")
public suspend fun partnerProfileId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.partnerProfileId = mapped
}
/**
* @param value Signing algorithm for this AS2 connector configuration.
*/
@JvmName("pcxhhgnxccgkmmxq")
public suspend fun signingAlgorithm(`value`: ConnectorAs2ConfigPropertiesSigningAlgorithm?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.signingAlgorithm = mapped
}
internal fun build(): As2ConfigPropertiesArgs = As2ConfigPropertiesArgs(
basicAuthSecretId = basicAuthSecretId,
compression = compression,
encryptionAlgorithm = encryptionAlgorithm,
localProfileId = localProfileId,
mdnResponse = mdnResponse,
mdnSigningAlgorithm = mdnSigningAlgorithm,
messageSubject = messageSubject,
partnerProfileId = partnerProfileId,
signingAlgorithm = signingAlgorithm,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy