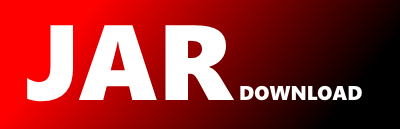
com.pulumi.awsnative.wafv2.kotlin.inputs.WebAclResponseInspectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.wafv2.kotlin.inputs
import com.pulumi.awsnative.wafv2.inputs.WebAclResponseInspectionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Configures the inspection of login responses
* @property bodyContains Configures inspection of the response body for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response body.
* @property header Configures inspection of the response header for success and failure indicators.
* @property json Configures inspection of the response JSON for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response JSON.
* @property statusCode Configures inspection of the response status code for success and failure indicators.
*/
public data class WebAclResponseInspectionArgs(
public val bodyContains: Output? = null,
public val `header`: Output? = null,
public val json: Output? = null,
public val statusCode: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.wafv2.inputs.WebAclResponseInspectionArgs =
com.pulumi.awsnative.wafv2.inputs.WebAclResponseInspectionArgs.builder()
.bodyContains(bodyContains?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.`header`(`header`?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.json(json?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.statusCode(statusCode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [WebAclResponseInspectionArgs].
*/
@PulumiTagMarker
public class WebAclResponseInspectionArgsBuilder internal constructor() {
private var bodyContains: Output? = null
private var `header`: Output? = null
private var json: Output? = null
private var statusCode: Output? = null
/**
* @param value Configures inspection of the response body for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response body.
*/
@JvmName("acbgnexqeyijdwtt")
public suspend fun bodyContains(`value`: Output) {
this.bodyContains = value
}
/**
* @param value Configures inspection of the response header for success and failure indicators.
*/
@JvmName("xfudjrwetxhdchlb")
public suspend fun `header`(`value`: Output) {
this.`header` = value
}
/**
* @param value Configures inspection of the response JSON for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response JSON.
*/
@JvmName("usogeeynlrhnpbup")
public suspend fun json(`value`: Output) {
this.json = value
}
/**
* @param value Configures inspection of the response status code for success and failure indicators.
*/
@JvmName("aelhbjyhuwaqmwbm")
public suspend fun statusCode(`value`: Output) {
this.statusCode = value
}
/**
* @param value Configures inspection of the response body for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response body.
*/
@JvmName("dmpcvtyqkgalrghc")
public suspend fun bodyContains(`value`: WebAclResponseInspectionBodyContainsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bodyContains = mapped
}
/**
* @param argument Configures inspection of the response body for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response body.
*/
@JvmName("whmgfbfryevdrdcf")
public suspend fun bodyContains(argument: suspend WebAclResponseInspectionBodyContainsArgsBuilder.() -> Unit) {
val toBeMapped = WebAclResponseInspectionBodyContainsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.bodyContains = mapped
}
/**
* @param value Configures inspection of the response header for success and failure indicators.
*/
@JvmName("usyjyirqicavdqns")
public suspend fun `header`(`value`: WebAclResponseInspectionHeaderArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`header` = mapped
}
/**
* @param argument Configures inspection of the response header for success and failure indicators.
*/
@JvmName("surotnxmjntonjio")
public suspend fun `header`(argument: suspend WebAclResponseInspectionHeaderArgsBuilder.() -> Unit) {
val toBeMapped = WebAclResponseInspectionHeaderArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.`header` = mapped
}
/**
* @param value Configures inspection of the response JSON for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response JSON.
*/
@JvmName("eamqmjwiddgidhra")
public suspend fun json(`value`: WebAclResponseInspectionJsonArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.json = mapped
}
/**
* @param argument Configures inspection of the response JSON for success and failure indicators. AWS WAF can inspect the first 65,536 bytes (64 KB) of the response JSON.
*/
@JvmName("cyebcccvmfbqnwrh")
public suspend fun json(argument: suspend WebAclResponseInspectionJsonArgsBuilder.() -> Unit) {
val toBeMapped = WebAclResponseInspectionJsonArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.json = mapped
}
/**
* @param value Configures inspection of the response status code for success and failure indicators.
*/
@JvmName("nxgguqjqxwdpgcis")
public suspend fun statusCode(`value`: WebAclResponseInspectionStatusCodeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.statusCode = mapped
}
/**
* @param argument Configures inspection of the response status code for success and failure indicators.
*/
@JvmName("rkoewkxhyihtjekl")
public suspend fun statusCode(argument: suspend WebAclResponseInspectionStatusCodeArgsBuilder.() -> Unit) {
val toBeMapped = WebAclResponseInspectionStatusCodeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.statusCode = mapped
}
internal fun build(): WebAclResponseInspectionArgs = WebAclResponseInspectionArgs(
bodyContains = bodyContains,
`header` = `header`,
json = json,
statusCode = statusCode,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy