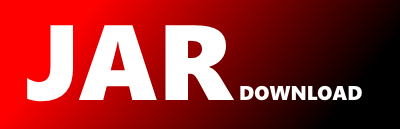
com.pulumi.awsnative.wisdom.kotlin.KnowledgeBaseArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-native-kotlin Show documentation
Show all versions of pulumi-aws-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.wisdom.kotlin
import com.pulumi.awsnative.kotlin.inputs.CreateOnlyTagArgs
import com.pulumi.awsnative.kotlin.inputs.CreateOnlyTagArgsBuilder
import com.pulumi.awsnative.wisdom.KnowledgeBaseArgs.builder
import com.pulumi.awsnative.wisdom.kotlin.enums.KnowledgeBaseType
import com.pulumi.awsnative.wisdom.kotlin.inputs.KnowledgeBaseRenderingConfigurationArgs
import com.pulumi.awsnative.wisdom.kotlin.inputs.KnowledgeBaseRenderingConfigurationArgsBuilder
import com.pulumi.awsnative.wisdom.kotlin.inputs.KnowledgeBaseServerSideEncryptionConfigurationArgs
import com.pulumi.awsnative.wisdom.kotlin.inputs.KnowledgeBaseServerSideEncryptionConfigurationArgsBuilder
import com.pulumi.awsnative.wisdom.kotlin.inputs.KnowledgeBaseSourceConfigurationArgs
import com.pulumi.awsnative.wisdom.kotlin.inputs.KnowledgeBaseSourceConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Definition of AWS::Wisdom::KnowledgeBase Resource Type
* @property description The description.
* @property knowledgeBaseType The type of knowledge base. Only CUSTOM knowledge bases allow you to upload your own content. EXTERNAL knowledge bases support integrations with third-party systems whose content is synchronized automatically.
* @property name The name of the knowledge base.
* @property renderingConfiguration Information about how to render the content.
* @property serverSideEncryptionConfiguration This customer managed key must have a policy that allows `kms:CreateGrant` and `kms:DescribeKey` permissions to the IAM identity using the key to invoke Wisdom. For more information about setting up a customer managed key for Wisdom, see [Enable Amazon Connect Wisdom for your instance](https://docs.aws.amazon.com/connect/latest/adminguide/enable-wisdom.html) . For information about valid ID values, see [Key identifiers (KeyId)](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#key-id) in the *AWS Key Management Service Developer Guide* .
* @property sourceConfiguration The source of the knowledge base content. Only set this argument for EXTERNAL knowledge bases.
* @property tags The tags used to organize, track, or control access for this resource.
*/
public data class KnowledgeBaseArgs(
public val description: Output? = null,
public val knowledgeBaseType: Output? = null,
public val name: Output? = null,
public val renderingConfiguration: Output? = null,
public val serverSideEncryptionConfiguration: Output? = null,
public val sourceConfiguration: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.wisdom.KnowledgeBaseArgs =
com.pulumi.awsnative.wisdom.KnowledgeBaseArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.knowledgeBaseType(knowledgeBaseType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 }))
.renderingConfiguration(
renderingConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.serverSideEncryptionConfiguration(
serverSideEncryptionConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.sourceConfiguration(
sourceConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [KnowledgeBaseArgs].
*/
@PulumiTagMarker
public class KnowledgeBaseArgsBuilder internal constructor() {
private var description: Output? = null
private var knowledgeBaseType: Output? = null
private var name: Output? = null
private var renderingConfiguration: Output? = null
private var serverSideEncryptionConfiguration:
Output? = null
private var sourceConfiguration: Output? = null
private var tags: Output>? = null
/**
* @param value The description.
*/
@JvmName("rnkujdocxpsmgbyv")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The type of knowledge base. Only CUSTOM knowledge bases allow you to upload your own content. EXTERNAL knowledge bases support integrations with third-party systems whose content is synchronized automatically.
*/
@JvmName("lfjmfbpttayoxrcb")
public suspend fun knowledgeBaseType(`value`: Output) {
this.knowledgeBaseType = value
}
/**
* @param value The name of the knowledge base.
*/
@JvmName("blbyyroecxdtpnxw")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Information about how to render the content.
*/
@JvmName("giqevupsgblphenu")
public suspend fun renderingConfiguration(`value`: Output) {
this.renderingConfiguration = value
}
/**
* @param value This customer managed key must have a policy that allows `kms:CreateGrant` and `kms:DescribeKey` permissions to the IAM identity using the key to invoke Wisdom. For more information about setting up a customer managed key for Wisdom, see [Enable Amazon Connect Wisdom for your instance](https://docs.aws.amazon.com/connect/latest/adminguide/enable-wisdom.html) . For information about valid ID values, see [Key identifiers (KeyId)](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#key-id) in the *AWS Key Management Service Developer Guide* .
*/
@JvmName("cgkmagrkaokmdgma")
public suspend fun serverSideEncryptionConfiguration(`value`: Output) {
this.serverSideEncryptionConfiguration = value
}
/**
* @param value The source of the knowledge base content. Only set this argument for EXTERNAL knowledge bases.
*/
@JvmName("axjthgashhvcalgv")
public suspend fun sourceConfiguration(`value`: Output) {
this.sourceConfiguration = value
}
/**
* @param value The tags used to organize, track, or control access for this resource.
*/
@JvmName("fasguabawmbjudif")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("cpmygaerdlqrctys")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values The tags used to organize, track, or control access for this resource.
*/
@JvmName("effnoyiepqxcivdh")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy