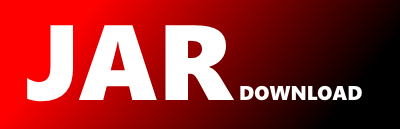
com.pulumi.awsnative.acmpca.kotlin.CertificateArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.acmpca.kotlin
import com.pulumi.awsnative.acmpca.CertificateArgs.builder
import com.pulumi.awsnative.acmpca.kotlin.inputs.CertificateApiPassthroughArgs
import com.pulumi.awsnative.acmpca.kotlin.inputs.CertificateApiPassthroughArgsBuilder
import com.pulumi.awsnative.acmpca.kotlin.inputs.CertificateValidityArgs
import com.pulumi.awsnative.acmpca.kotlin.inputs.CertificateValidityArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The ``AWS::ACMPCA::Certificate`` resource is used to issue a certificate using your private certificate authority. For more information, see the [IssueCertificate](https://docs.aws.amazon.com/privateca/latest/APIReference/API_IssueCertificate.html) action.
* @property apiPassthrough Specifies X.509 certificate information to be included in the issued certificate. An ``APIPassthrough`` or ``APICSRPassthrough`` template variant must be selected, or else this parameter is ignored.
* @property certificateAuthorityArn The Amazon Resource Name (ARN) for the private CA issues the certificate.
* @property certificateSigningRequest The certificate signing request (CSR) for the certificate.
* @property signingAlgorithm The name of the algorithm that will be used to sign the certificate to be issued.
* This parameter should not be confused with the ``SigningAlgorithm`` parameter used to sign a CSR in the ``CreateCertificateAuthority`` action.
* The specified signing algorithm family (RSA or ECDSA) must match the algorithm family of the CA's secret key.
* @property templateArn Specifies a custom configuration template to use when issuing a certificate. If this parameter is not provided, PCAshort defaults to the ``EndEntityCertificate/V1`` template. For more information about PCAshort templates, see [Using Templates](https://docs.aws.amazon.com/privateca/latest/userguide/UsingTemplates.html).
* @property validity The period of time during which the certificate will be valid.
* @property validityNotBefore Information describing the start of the validity period of the certificate. This parameter sets the "Not Before" date for the certificate.
* By default, when issuing a certificate, PCAshort sets the "Not Before" date to the issuance time minus 60 minutes. This compensates for clock inconsistencies across computer systems. The ``ValidityNotBefore`` parameter can be used to customize the "Not Before" value.
* Unlike the ``Validity`` parameter, the ``ValidityNotBefore`` parameter is optional.
* The ``ValidityNotBefore`` value is expressed as an explicit date and time, using the ``Validity`` type value ``ABSOLUTE``.
*/
public data class CertificateArgs(
public val apiPassthrough: Output? = null,
public val certificateAuthorityArn: Output? = null,
public val certificateSigningRequest: Output? = null,
public val signingAlgorithm: Output? = null,
public val templateArn: Output? = null,
public val validity: Output? = null,
public val validityNotBefore: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.acmpca.CertificateArgs =
com.pulumi.awsnative.acmpca.CertificateArgs.builder()
.apiPassthrough(apiPassthrough?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.certificateAuthorityArn(certificateAuthorityArn?.applyValue({ args0 -> args0 }))
.certificateSigningRequest(certificateSigningRequest?.applyValue({ args0 -> args0 }))
.signingAlgorithm(signingAlgorithm?.applyValue({ args0 -> args0 }))
.templateArn(templateArn?.applyValue({ args0 -> args0 }))
.validity(validity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.validityNotBefore(
validityNotBefore?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [CertificateArgs].
*/
@PulumiTagMarker
public class CertificateArgsBuilder internal constructor() {
private var apiPassthrough: Output? = null
private var certificateAuthorityArn: Output? = null
private var certificateSigningRequest: Output? = null
private var signingAlgorithm: Output? = null
private var templateArn: Output? = null
private var validity: Output? = null
private var validityNotBefore: Output? = null
/**
* @param value Specifies X.509 certificate information to be included in the issued certificate. An ``APIPassthrough`` or ``APICSRPassthrough`` template variant must be selected, or else this parameter is ignored.
*/
@JvmName("pqtodspbhmtiiugf")
public suspend fun apiPassthrough(`value`: Output) {
this.apiPassthrough = value
}
/**
* @param value The Amazon Resource Name (ARN) for the private CA issues the certificate.
*/
@JvmName("knkilugmvwiryttq")
public suspend fun certificateAuthorityArn(`value`: Output) {
this.certificateAuthorityArn = value
}
/**
* @param value The certificate signing request (CSR) for the certificate.
*/
@JvmName("reakmuabilqajdtr")
public suspend fun certificateSigningRequest(`value`: Output) {
this.certificateSigningRequest = value
}
/**
* @param value The name of the algorithm that will be used to sign the certificate to be issued.
* This parameter should not be confused with the ``SigningAlgorithm`` parameter used to sign a CSR in the ``CreateCertificateAuthority`` action.
* The specified signing algorithm family (RSA or ECDSA) must match the algorithm family of the CA's secret key.
*/
@JvmName("ypkhidlvwntfvkkc")
public suspend fun signingAlgorithm(`value`: Output) {
this.signingAlgorithm = value
}
/**
* @param value Specifies a custom configuration template to use when issuing a certificate. If this parameter is not provided, PCAshort defaults to the ``EndEntityCertificate/V1`` template. For more information about PCAshort templates, see [Using Templates](https://docs.aws.amazon.com/privateca/latest/userguide/UsingTemplates.html).
*/
@JvmName("nwckrelfadnjqabt")
public suspend fun templateArn(`value`: Output) {
this.templateArn = value
}
/**
* @param value The period of time during which the certificate will be valid.
*/
@JvmName("njjqwpsxjkfulssf")
public suspend fun validity(`value`: Output) {
this.validity = value
}
/**
* @param value Information describing the start of the validity period of the certificate. This parameter sets the "Not Before" date for the certificate.
* By default, when issuing a certificate, PCAshort sets the "Not Before" date to the issuance time minus 60 minutes. This compensates for clock inconsistencies across computer systems. The ``ValidityNotBefore`` parameter can be used to customize the "Not Before" value.
* Unlike the ``Validity`` parameter, the ``ValidityNotBefore`` parameter is optional.
* The ``ValidityNotBefore`` value is expressed as an explicit date and time, using the ``Validity`` type value ``ABSOLUTE``.
*/
@JvmName("snobdtpkawdhiyfq")
public suspend fun validityNotBefore(`value`: Output) {
this.validityNotBefore = value
}
/**
* @param value Specifies X.509 certificate information to be included in the issued certificate. An ``APIPassthrough`` or ``APICSRPassthrough`` template variant must be selected, or else this parameter is ignored.
*/
@JvmName("pckqavslaqnfsjtm")
public suspend fun apiPassthrough(`value`: CertificateApiPassthroughArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiPassthrough = mapped
}
/**
* @param argument Specifies X.509 certificate information to be included in the issued certificate. An ``APIPassthrough`` or ``APICSRPassthrough`` template variant must be selected, or else this parameter is ignored.
*/
@JvmName("gkgnckhtsqperknx")
public suspend fun apiPassthrough(argument: suspend CertificateApiPassthroughArgsBuilder.() -> Unit) {
val toBeMapped = CertificateApiPassthroughArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.apiPassthrough = mapped
}
/**
* @param value The Amazon Resource Name (ARN) for the private CA issues the certificate.
*/
@JvmName("nxbbmatbqckoxsfr")
public suspend fun certificateAuthorityArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certificateAuthorityArn = mapped
}
/**
* @param value The certificate signing request (CSR) for the certificate.
*/
@JvmName("llfppaldwkcmmuux")
public suspend fun certificateSigningRequest(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certificateSigningRequest = mapped
}
/**
* @param value The name of the algorithm that will be used to sign the certificate to be issued.
* This parameter should not be confused with the ``SigningAlgorithm`` parameter used to sign a CSR in the ``CreateCertificateAuthority`` action.
* The specified signing algorithm family (RSA or ECDSA) must match the algorithm family of the CA's secret key.
*/
@JvmName("geptskwndqcctaqh")
public suspend fun signingAlgorithm(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.signingAlgorithm = mapped
}
/**
* @param value Specifies a custom configuration template to use when issuing a certificate. If this parameter is not provided, PCAshort defaults to the ``EndEntityCertificate/V1`` template. For more information about PCAshort templates, see [Using Templates](https://docs.aws.amazon.com/privateca/latest/userguide/UsingTemplates.html).
*/
@JvmName("shiklmfyxrggcbbq")
public suspend fun templateArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.templateArn = mapped
}
/**
* @param value The period of time during which the certificate will be valid.
*/
@JvmName("rtchrchcfmayqtma")
public suspend fun validity(`value`: CertificateValidityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validity = mapped
}
/**
* @param argument The period of time during which the certificate will be valid.
*/
@JvmName("yxxdtedotdlcosjj")
public suspend fun validity(argument: suspend CertificateValidityArgsBuilder.() -> Unit) {
val toBeMapped = CertificateValidityArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.validity = mapped
}
/**
* @param value Information describing the start of the validity period of the certificate. This parameter sets the "Not Before" date for the certificate.
* By default, when issuing a certificate, PCAshort sets the "Not Before" date to the issuance time minus 60 minutes. This compensates for clock inconsistencies across computer systems. The ``ValidityNotBefore`` parameter can be used to customize the "Not Before" value.
* Unlike the ``Validity`` parameter, the ``ValidityNotBefore`` parameter is optional.
* The ``ValidityNotBefore`` value is expressed as an explicit date and time, using the ``Validity`` type value ``ABSOLUTE``.
*/
@JvmName("uxucovjycaiayhla")
public suspend fun validityNotBefore(`value`: CertificateValidityArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validityNotBefore = mapped
}
/**
* @param argument Information describing the start of the validity period of the certificate. This parameter sets the "Not Before" date for the certificate.
* By default, when issuing a certificate, PCAshort sets the "Not Before" date to the issuance time minus 60 minutes. This compensates for clock inconsistencies across computer systems. The ``ValidityNotBefore`` parameter can be used to customize the "Not Before" value.
* Unlike the ``Validity`` parameter, the ``ValidityNotBefore`` parameter is optional.
* The ``ValidityNotBefore`` value is expressed as an explicit date and time, using the ``Validity`` type value ``ABSOLUTE``.
*/
@JvmName("aignnsjxaymmtfar")
public suspend fun validityNotBefore(argument: suspend CertificateValidityArgsBuilder.() -> Unit) {
val toBeMapped = CertificateValidityArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.validityNotBefore = mapped
}
internal fun build(): CertificateArgs = CertificateArgs(
apiPassthrough = apiPassthrough,
certificateAuthorityArn = certificateAuthorityArn,
certificateSigningRequest = certificateSigningRequest,
signingAlgorithm = signingAlgorithm,
templateArn = templateArn,
validity = validity,
validityNotBefore = validityNotBefore,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy