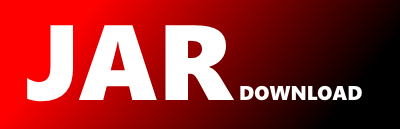
com.pulumi.awsnative.acmpca.kotlin.outputs.CertificateSubject.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.acmpca.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* Contains information about the certificate subject. The ``Subject`` field in the certificate identifies the entity that owns or controls the public key in the certificate. The entity can be a user, computer, device, or service. The ``Subject``must contain an X.500 distinguished name (DN). A DN is a sequence of relative distinguished names (RDNs). The RDNs are separated by commas in the certificate.
* @property commonName For CA and end-entity certificates in a private PKI, the common name (CN) can be any string within the length limit.
* Note: In publicly trusted certificates, the common name must be a fully qualified domain name (FQDN) associated with the certificate subject.
* @property country Two-digit code that specifies the country in which the certificate subject located.
* @property customAttributes Contains a sequence of one or more X.500 relative distinguished names (RDNs), each of which consists of an object identifier (OID) and a value. For more information, see NIST’s definition of [Object Identifier (OID)](https://docs.aws.amazon.com/https://csrc.nist.gov/glossary/term/Object_Identifier).
* Custom attributes cannot be used in combination with standard attributes.
* @property distinguishedNameQualifier Disambiguating information for the certificate subject.
* @property generationQualifier Typically a qualifier appended to the name of an individual. Examples include Jr. for junior, Sr. for senior, and III for third.
* @property givenName First name.
* @property initials Concatenation that typically contains the first letter of the *GivenName*, the first letter of the middle name if one exists, and the first letter of the *Surname*.
* @property locality The locality (such as a city or town) in which the certificate subject is located.
* @property organization Legal name of the organization with which the certificate subject is affiliated.
* @property organizationalUnit A subdivision or unit of the organization (such as sales or finance) with which the certificate subject is affiliated.
* @property pseudonym Typically a shortened version of a longer *GivenName*. For example, Jonathan is often shortened to John. Elizabeth is often shortened to Beth, Liz, or Eliza.
* @property serialNumber The certificate serial number.
* @property state State in which the subject of the certificate is located.
* @property surname Family name. In the US and the UK, for example, the surname of an individual is ordered last. In Asian cultures the surname is typically ordered first.
* @property title A title such as Mr. or Ms., which is pre-pended to the name to refer formally to the certificate subject.
*/
public data class CertificateSubject(
public val commonName: String? = null,
public val country: String? = null,
public val customAttributes: List? = null,
public val distinguishedNameQualifier: String? = null,
public val generationQualifier: String? = null,
public val givenName: String? = null,
public val initials: String? = null,
public val locality: String? = null,
public val organization: String? = null,
public val organizationalUnit: String? = null,
public val pseudonym: String? = null,
public val serialNumber: String? = null,
public val state: String? = null,
public val surname: String? = null,
public val title: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.acmpca.outputs.CertificateSubject): CertificateSubject = CertificateSubject(
commonName = javaType.commonName().map({ args0 -> args0 }).orElse(null),
country = javaType.country().map({ args0 -> args0 }).orElse(null),
customAttributes = javaType.customAttributes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.acmpca.kotlin.outputs.CertificateCustomAttribute.Companion.toKotlin(args0)
})
}),
distinguishedNameQualifier = javaType.distinguishedNameQualifier().map({ args0 ->
args0
}).orElse(null),
generationQualifier = javaType.generationQualifier().map({ args0 -> args0 }).orElse(null),
givenName = javaType.givenName().map({ args0 -> args0 }).orElse(null),
initials = javaType.initials().map({ args0 -> args0 }).orElse(null),
locality = javaType.locality().map({ args0 -> args0 }).orElse(null),
organization = javaType.organization().map({ args0 -> args0 }).orElse(null),
organizationalUnit = javaType.organizationalUnit().map({ args0 -> args0 }).orElse(null),
pseudonym = javaType.pseudonym().map({ args0 -> args0 }).orElse(null),
serialNumber = javaType.serialNumber().map({ args0 -> args0 }).orElse(null),
state = javaType.state().map({ args0 -> args0 }).orElse(null),
surname = javaType.surname().map({ args0 -> args0 }).orElse(null),
title = javaType.title().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy