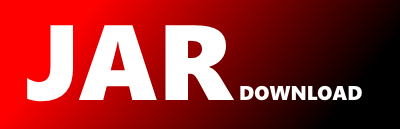
com.pulumi.awsnative.amplify.kotlin.App.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.amplify.kotlin
import com.pulumi.awsnative.amplify.kotlin.enums.AppPlatform
import com.pulumi.awsnative.amplify.kotlin.outputs.AppAutoBranchCreationConfig
import com.pulumi.awsnative.amplify.kotlin.outputs.AppBasicAuthConfig
import com.pulumi.awsnative.amplify.kotlin.outputs.AppCacheConfig
import com.pulumi.awsnative.amplify.kotlin.outputs.AppCustomRule
import com.pulumi.awsnative.amplify.kotlin.outputs.AppEnvironmentVariable
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.amplify.kotlin.enums.AppPlatform.Companion.toKotlin as appPlatformToKotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.AppAutoBranchCreationConfig.Companion.toKotlin as appAutoBranchCreationConfigToKotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.AppBasicAuthConfig.Companion.toKotlin as appBasicAuthConfigToKotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.AppCacheConfig.Companion.toKotlin as appCacheConfigToKotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.AppCustomRule.Companion.toKotlin as appCustomRuleToKotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.AppEnvironmentVariable.Companion.toKotlin as appEnvironmentVariableToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [App].
*/
@PulumiTagMarker
public class AppResourceBuilder internal constructor() {
public var name: String? = null
public var args: AppArgs = AppArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AppArgsBuilder.() -> Unit) {
val builder = AppArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): App {
val builtJavaResource = com.pulumi.awsnative.amplify.App(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return App(builtJavaResource)
}
}
/**
* The AWS::Amplify::App resource creates Apps in the Amplify Console. An App is a collection of branches.
*/
public class App internal constructor(
override val javaResource: com.pulumi.awsnative.amplify.App,
) : KotlinCustomResource(javaResource, AppMapper) {
/**
* The personal access token for a GitHub repository for an Amplify app. The personal access token is used to authorize access to a GitHub repository using the Amplify GitHub App. The token is not stored.
* Use `AccessToken` for GitHub repositories only. To authorize access to a repository provider such as Bitbucket or CodeCommit, use `OauthToken` .
* You must specify either `AccessToken` or `OauthToken` when you create a new app.
* Existing Amplify apps deployed from a GitHub repository using OAuth continue to work with CI/CD. However, we strongly recommend that you migrate these apps to use the GitHub App. For more information, see [Migrating an existing OAuth app to the Amplify GitHub App](https://docs.aws.amazon.com/amplify/latest/userguide/setting-up-GitHub-access.html#migrating-to-github-app-auth) in the *Amplify User Guide* .
*/
public val accessToken: Output?
get() = javaResource.accessToken().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Unique Id for the Amplify App.
*/
public val appId: Output
get() = javaResource.appId().applyValue({ args0 -> args0 })
/**
* Name for the Amplify App.
*/
public val appName: Output
get() = javaResource.appName().applyValue({ args0 -> args0 })
/**
* ARN for the Amplify App.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Sets the configuration for your automatic branch creation.
*/
public val autoBranchCreationConfig: Output?
get() = javaResource.autoBranchCreationConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> appAutoBranchCreationConfigToKotlin(args0) })
}).orElse(null)
})
/**
* The credentials for basic authorization for an Amplify app. You must base64-encode the authorization credentials and provide them in the format `user:password` .
*/
public val basicAuthConfig: Output?
get() = javaResource.basicAuthConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> appBasicAuthConfigToKotlin(args0) })
}).orElse(null)
})
/**
* The build specification (build spec) for an Amplify app.
*/
public val buildSpec: Output?
get() = javaResource.buildSpec().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The cache configuration for the Amplify app. If you don't specify the cache configuration `type` , Amplify uses the default `AMPLIFY_MANAGED` setting.
*/
public val cacheConfig: Output?
get() = javaResource.cacheConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
appCacheConfigToKotlin(args0)
})
}).orElse(null)
})
/**
* The custom HTTP headers for an Amplify app.
*/
public val customHeaders: Output?
get() = javaResource.customHeaders().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The custom rewrite and redirect rules for an Amplify app.
*/
public val customRules: Output>?
get() = javaResource.customRules().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> appCustomRuleToKotlin(args0) })
})
}).orElse(null)
})
/**
* Default domain for the Amplify App.
*/
public val defaultDomain: Output
get() = javaResource.defaultDomain().applyValue({ args0 -> args0 })
/**
* The description of the Amplify app.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Automatically disconnect a branch in Amplify Hosting when you delete a branch from your Git repository.
*/
public val enableBranchAutoDeletion: Output?
get() = javaResource.enableBranchAutoDeletion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The environment variables for the Amplify app.
* For a list of the environment variables that are accessible to Amplify by default, see [Amplify Environment variables](https://docs.aws.amazon.com/amplify/latest/userguide/amplify-console-environment-variables.html) in the *Amplify Hosting User Guide* .
*/
public val environmentVariables: Output>?
get() = javaResource.environmentVariables().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
appEnvironmentVariableToKotlin(args0)
})
})
}).orElse(null)
})
/**
* AWS Identity and Access Management ( IAM ) service role for the Amazon Resource Name (ARN) of the Amplify app.
*/
public val iamServiceRole: Output?
get() = javaResource.iamServiceRole().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the Amplify app.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The OAuth token for a third-party source control system for an Amplify app. The OAuth token is used to create a webhook and a read-only deploy key using SSH cloning. The OAuth token is not stored.
* Use `OauthToken` for repository providers other than GitHub, such as Bitbucket or CodeCommit. To authorize access to GitHub as your repository provider, use `AccessToken` .
* You must specify either `OauthToken` or `AccessToken` when you create a new app.
* Existing Amplify apps deployed from a GitHub repository using OAuth continue to work with CI/CD. However, we strongly recommend that you migrate these apps to use the GitHub App. For more information, see [Migrating an existing OAuth app to the Amplify GitHub App](https://docs.aws.amazon.com/amplify/latest/userguide/setting-up-GitHub-access.html#migrating-to-github-app-auth) in the *Amplify User Guide* .
*/
public val oauthToken: Output?
get() = javaResource.oauthToken().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The platform for the Amplify app. For a static app, set the platform type to `WEB` . For a dynamic server-side rendered (SSR) app, set the platform type to `WEB_COMPUTE` . For an app requiring Amplify Hosting's original SSR support only, set the platform type to `WEB_DYNAMIC` .
* If you are deploying an SSG only app with Next.js version 14 or later, you must set the platform type to `WEB_COMPUTE` and set the artifacts `baseDirectory` to `.next` in the application's build settings. For an example of the build specification settings, see [Amplify build settings for a Next.js 14 SSG application](https://docs.aws.amazon.com/amplify/latest/userguide/deploy-nextjs-app.html#build-setting-detection-ssg-14) in the *Amplify Hosting User Guide* .
*/
public val platform: Output?
get() = javaResource.platform().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
appPlatformToKotlin(args0)
})
}).orElse(null)
})
/**
* The Git repository for the Amplify app.
*/
public val repository: Output?
get() = javaResource.repository().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The tag for an Amplify app.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
}
public object AppMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.amplify.App::class == javaResource::class
override fun map(javaResource: Resource): App = App(
javaResource as
com.pulumi.awsnative.amplify.App,
)
}
/**
* @see [App].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [App].
*/
public suspend fun app(name: String, block: suspend AppResourceBuilder.() -> Unit): App {
val builder = AppResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [App].
* @param name The _unique_ name of the resulting resource.
*/
public fun app(name: String): App {
val builder = AppResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy