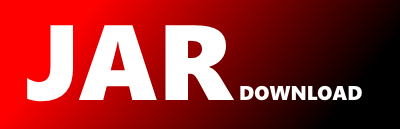
com.pulumi.awsnative.amplify.kotlin.Domain.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.amplify.kotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.DomainCertificate
import com.pulumi.awsnative.amplify.kotlin.outputs.DomainCertificateSettings
import com.pulumi.awsnative.amplify.kotlin.outputs.DomainSubDomainSetting
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.amplify.kotlin.outputs.DomainCertificate.Companion.toKotlin as domainCertificateToKotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.DomainCertificateSettings.Companion.toKotlin as domainCertificateSettingsToKotlin
import com.pulumi.awsnative.amplify.kotlin.outputs.DomainSubDomainSetting.Companion.toKotlin as domainSubDomainSettingToKotlin
/**
* Builder for [Domain].
*/
@PulumiTagMarker
public class DomainResourceBuilder internal constructor() {
public var name: String? = null
public var args: DomainArgs = DomainArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DomainArgsBuilder.() -> Unit) {
val builder = DomainArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Domain {
val builtJavaResource = com.pulumi.awsnative.amplify.Domain(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Domain(builtJavaResource)
}
}
/**
* The AWS::Amplify::Domain resource allows you to connect a custom domain to your app.
*/
public class Domain internal constructor(
override val javaResource: com.pulumi.awsnative.amplify.Domain,
) : KotlinCustomResource(javaResource, DomainMapper) {
/**
* The unique ID for an Amplify app.
*/
public val appId: Output
get() = javaResource.appId().applyValue({ args0 -> args0 })
/**
* ARN for the Domain Association.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Sets the branch patterns for automatic subdomain creation.
*/
public val autoSubDomainCreationPatterns: Output>?
get() = javaResource.autoSubDomainCreationPatterns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The required AWS Identity and Access Management (IAMlong) service role for the Amazon Resource Name (ARN) for automatically creating subdomains.
*/
public val autoSubDomainIamRole: Output?
get() = javaResource.autoSubDomainIamRole().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val certificate: Output
get() = javaResource.certificate().applyValue({ args0 ->
args0.let({ args0 ->
domainCertificateToKotlin(args0)
})
})
/**
* DNS Record for certificate verification.
*/
public val certificateRecord: Output
get() = javaResource.certificateRecord().applyValue({ args0 -> args0 })
/**
* The type of SSL/TLS certificate to use for your custom domain. If you don't specify a certificate type, Amplify uses the default certificate that it provisions and manages for you.
*/
public val certificateSettings: Output?
get() = javaResource.certificateSettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> domainCertificateSettingsToKotlin(args0) })
}).orElse(null)
})
/**
* The domain name for the domain association.
*/
public val domainName: Output
get() = javaResource.domainName().applyValue({ args0 -> args0 })
/**
* Status for the Domain Association.
*/
public val domainStatus: Output
get() = javaResource.domainStatus().applyValue({ args0 -> args0 })
/**
* Enables the automated creation of subdomains for branches.
*/
public val enableAutoSubDomain: Output?
get() = javaResource.enableAutoSubDomain().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Reason for the current status of the domain.
*/
public val statusReason: Output
get() = javaResource.statusReason().applyValue({ args0 -> args0 })
/**
* The setting for the subdomain.
*/
public val subDomainSettings: Output>
get() = javaResource.subDomainSettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> domainSubDomainSettingToKotlin(args0) })
})
})
/**
* The status of the domain update operation that is currently in progress. The following list describes the valid update states.
* - **REQUESTING_CERTIFICATE** - The certificate is in the process of being updated.
* - **PENDING_VERIFICATION** - Indicates that an Amplify managed certificate is in the process of being verified. This occurs during the creation of a custom domain or when a custom domain is updated to use a managed certificate.
* - **IMPORTING_CUSTOM_CERTIFICATE** - Indicates that an Amplify custom certificate is in the process of being imported. This occurs during the creation of a custom domain or when a custom domain is updated to use a custom certificate.
* - **PENDING_DEPLOYMENT** - Indicates that the subdomain or certificate changes are being propagated.
* - **AWAITING_APP_CNAME** - Amplify is waiting for CNAME records corresponding to subdomains to be propagated. If your custom domain is on Route 53, Amplify handles this for you automatically. For more information about custom domains, see [Setting up custom domains](https://docs.aws.amazon.com/amplify/latest/userguide/custom-domains.html) in the *Amplify Hosting User Guide* .
* - **UPDATE_COMPLETE** - The certificate has been associated with a domain.
* - **UPDATE_FAILED** - The certificate has failed to be provisioned or associated, and there is no existing active certificate to roll back to.
*/
public val updateStatus: Output
get() = javaResource.updateStatus().applyValue({ args0 -> args0 })
}
public object DomainMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.amplify.Domain::class == javaResource::class
override fun map(javaResource: Resource): Domain = Domain(
javaResource as
com.pulumi.awsnative.amplify.Domain,
)
}
/**
* @see [Domain].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Domain].
*/
public suspend fun domain(name: String, block: suspend DomainResourceBuilder.() -> Unit): Domain {
val builder = DomainResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Domain].
* @param name The _unique_ name of the resulting resource.
*/
public fun domain(name: String): Domain {
val builder = DomainResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy