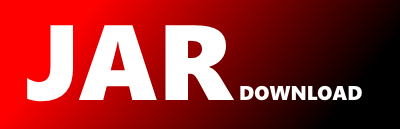
com.pulumi.awsnative.amplify.kotlin.outputs.GetAppResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.amplify.kotlin.outputs
import com.pulumi.awsnative.amplify.kotlin.enums.AppPlatform
import com.pulumi.awsnative.kotlin.outputs.Tag
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property appId Unique Id for the Amplify App.
* @property appName Name for the Amplify App.
* @property arn ARN for the Amplify App.
* @property buildSpec The build specification (build spec) for an Amplify app.
* @property cacheConfig The cache configuration for the Amplify app. If you don't specify the cache configuration `type` , Amplify uses the default `AMPLIFY_MANAGED` setting.
* @property customHeaders The custom HTTP headers for an Amplify app.
* @property customRules The custom rewrite and redirect rules for an Amplify app.
* @property defaultDomain Default domain for the Amplify App.
* @property description The description of the Amplify app.
* @property enableBranchAutoDeletion Automatically disconnect a branch in Amplify Hosting when you delete a branch from your Git repository.
* @property environmentVariables The environment variables for the Amplify app.
* For a list of the environment variables that are accessible to Amplify by default, see [Amplify Environment variables](https://docs.aws.amazon.com/amplify/latest/userguide/amplify-console-environment-variables.html) in the *Amplify Hosting User Guide* .
* @property iamServiceRole AWS Identity and Access Management ( IAM ) service role for the Amazon Resource Name (ARN) of the Amplify app.
* @property name The name of the Amplify app.
* @property platform The platform for the Amplify app. For a static app, set the platform type to `WEB` . For a dynamic server-side rendered (SSR) app, set the platform type to `WEB_COMPUTE` . For an app requiring Amplify Hosting's original SSR support only, set the platform type to `WEB_DYNAMIC` .
* If you are deploying an SSG only app with Next.js version 14 or later, you must set the platform type to `WEB_COMPUTE` and set the artifacts `baseDirectory` to `.next` in the application's build settings. For an example of the build specification settings, see [Amplify build settings for a Next.js 14 SSG application](https://docs.aws.amazon.com/amplify/latest/userguide/deploy-nextjs-app.html#build-setting-detection-ssg-14) in the *Amplify Hosting User Guide* .
* @property repository The Git repository for the Amplify app.
* @property tags The tag for an Amplify app.
*/
public data class GetAppResult(
public val appId: String? = null,
public val appName: String? = null,
public val arn: String? = null,
public val buildSpec: String? = null,
public val cacheConfig: AppCacheConfig? = null,
public val customHeaders: String? = null,
public val customRules: List? = null,
public val defaultDomain: String? = null,
public val description: String? = null,
public val enableBranchAutoDeletion: Boolean? = null,
public val environmentVariables: List? = null,
public val iamServiceRole: String? = null,
public val name: String? = null,
public val platform: AppPlatform? = null,
public val repository: String? = null,
public val tags: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.amplify.outputs.GetAppResult): GetAppResult =
GetAppResult(
appId = javaType.appId().map({ args0 -> args0 }).orElse(null),
appName = javaType.appName().map({ args0 -> args0 }).orElse(null),
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
buildSpec = javaType.buildSpec().map({ args0 -> args0 }).orElse(null),
cacheConfig = javaType.cacheConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.amplify.kotlin.outputs.AppCacheConfig.Companion.toKotlin(args0)
})
}).orElse(null),
customHeaders = javaType.customHeaders().map({ args0 -> args0 }).orElse(null),
customRules = javaType.customRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.amplify.kotlin.outputs.AppCustomRule.Companion.toKotlin(args0)
})
}),
defaultDomain = javaType.defaultDomain().map({ args0 -> args0 }).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
enableBranchAutoDeletion = javaType.enableBranchAutoDeletion().map({ args0 -> args0 }).orElse(null),
environmentVariables = javaType.environmentVariables().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.amplify.kotlin.outputs.AppEnvironmentVariable.Companion.toKotlin(args0)
})
}),
iamServiceRole = javaType.iamServiceRole().map({ args0 -> args0 }).orElse(null),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
platform = javaType.platform().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.amplify.kotlin.enums.AppPlatform.Companion.toKotlin(args0)
})
}).orElse(null),
repository = javaType.repository().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy