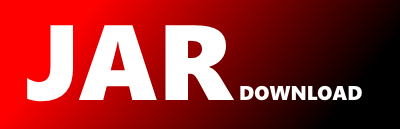
com.pulumi.awsnative.apigateway.kotlin.inputs.DeploymentMethodSettingArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.apigateway.kotlin.inputs
import com.pulumi.awsnative.apigateway.inputs.DeploymentMethodSettingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The ``MethodSetting`` property type configures settings for all methods in a stage.
* The ``MethodSettings`` property of the [Amazon API Gateway Deployment StageDescription](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-apigateway-deployment-stagedescription.html) property type contains a list of ``MethodSetting`` property types.
* @property cacheDataEncrypted Specifies whether the cached responses are encrypted.
* @property cacheTtlInSeconds Specifies the time to live (TTL), in seconds, for cached responses. The higher the TTL, the longer the response will be cached.
* @property cachingEnabled Specifies whether responses should be cached and returned for requests. A cache cluster must be enabled on the stage for responses to be cached.
* @property dataTraceEnabled Specifies whether data trace logging is enabled for this method, which affects the log entries pushed to Amazon CloudWatch Logs. This can be useful to troubleshoot APIs, but can result in logging sensitive data. We recommend that you don't enable this option for production APIs.
* @property httpMethod The HTTP method.
* @property loggingLevel Specifies the logging level for this method, which affects the log entries pushed to Amazon CloudWatch Logs. Valid values are ``OFF``, ``ERROR``, and ``INFO``. Choose ``ERROR`` to write only error-level entries to CloudWatch Logs, or choose ``INFO`` to include all ``ERROR`` events as well as extra informational events.
* @property metricsEnabled Specifies whether Amazon CloudWatch metrics are enabled for this method.
* @property resourcePath The resource path for this method. Forward slashes (``/``) are encoded as ``~1`` and the initial slash must include a forward slash. For example, the path value ``/resource/subresource`` must be encoded as ``/~1resource~1subresource``. To specify the root path, use only a slash (``/``).
* @property throttlingBurstLimit Specifies the throttling burst limit.
* @property throttlingRateLimit Specifies the throttling rate limit.
*/
public data class DeploymentMethodSettingArgs(
public val cacheDataEncrypted: Output? = null,
public val cacheTtlInSeconds: Output? = null,
public val cachingEnabled: Output? = null,
public val dataTraceEnabled: Output? = null,
public val httpMethod: Output? = null,
public val loggingLevel: Output? = null,
public val metricsEnabled: Output? = null,
public val resourcePath: Output? = null,
public val throttlingBurstLimit: Output? = null,
public val throttlingRateLimit: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.apigateway.inputs.DeploymentMethodSettingArgs =
com.pulumi.awsnative.apigateway.inputs.DeploymentMethodSettingArgs.builder()
.cacheDataEncrypted(cacheDataEncrypted?.applyValue({ args0 -> args0 }))
.cacheTtlInSeconds(cacheTtlInSeconds?.applyValue({ args0 -> args0 }))
.cachingEnabled(cachingEnabled?.applyValue({ args0 -> args0 }))
.dataTraceEnabled(dataTraceEnabled?.applyValue({ args0 -> args0 }))
.httpMethod(httpMethod?.applyValue({ args0 -> args0 }))
.loggingLevel(loggingLevel?.applyValue({ args0 -> args0 }))
.metricsEnabled(metricsEnabled?.applyValue({ args0 -> args0 }))
.resourcePath(resourcePath?.applyValue({ args0 -> args0 }))
.throttlingBurstLimit(throttlingBurstLimit?.applyValue({ args0 -> args0 }))
.throttlingRateLimit(throttlingRateLimit?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DeploymentMethodSettingArgs].
*/
@PulumiTagMarker
public class DeploymentMethodSettingArgsBuilder internal constructor() {
private var cacheDataEncrypted: Output? = null
private var cacheTtlInSeconds: Output? = null
private var cachingEnabled: Output? = null
private var dataTraceEnabled: Output? = null
private var httpMethod: Output? = null
private var loggingLevel: Output? = null
private var metricsEnabled: Output? = null
private var resourcePath: Output? = null
private var throttlingBurstLimit: Output? = null
private var throttlingRateLimit: Output? = null
/**
* @param value Specifies whether the cached responses are encrypted.
*/
@JvmName("ppieqnexjwmhkqpl")
public suspend fun cacheDataEncrypted(`value`: Output) {
this.cacheDataEncrypted = value
}
/**
* @param value Specifies the time to live (TTL), in seconds, for cached responses. The higher the TTL, the longer the response will be cached.
*/
@JvmName("gfmkcrxamtorxenk")
public suspend fun cacheTtlInSeconds(`value`: Output) {
this.cacheTtlInSeconds = value
}
/**
* @param value Specifies whether responses should be cached and returned for requests. A cache cluster must be enabled on the stage for responses to be cached.
*/
@JvmName("jdaklfewbfesyjex")
public suspend fun cachingEnabled(`value`: Output) {
this.cachingEnabled = value
}
/**
* @param value Specifies whether data trace logging is enabled for this method, which affects the log entries pushed to Amazon CloudWatch Logs. This can be useful to troubleshoot APIs, but can result in logging sensitive data. We recommend that you don't enable this option for production APIs.
*/
@JvmName("nbqanxrfplspvcyv")
public suspend fun dataTraceEnabled(`value`: Output) {
this.dataTraceEnabled = value
}
/**
* @param value The HTTP method.
*/
@JvmName("rsyyprddcovopwnx")
public suspend fun httpMethod(`value`: Output) {
this.httpMethod = value
}
/**
* @param value Specifies the logging level for this method, which affects the log entries pushed to Amazon CloudWatch Logs. Valid values are ``OFF``, ``ERROR``, and ``INFO``. Choose ``ERROR`` to write only error-level entries to CloudWatch Logs, or choose ``INFO`` to include all ``ERROR`` events as well as extra informational events.
*/
@JvmName("gvcsjdiemvholhvg")
public suspend fun loggingLevel(`value`: Output) {
this.loggingLevel = value
}
/**
* @param value Specifies whether Amazon CloudWatch metrics are enabled for this method.
*/
@JvmName("wqhcxsftgyrovyuq")
public suspend fun metricsEnabled(`value`: Output) {
this.metricsEnabled = value
}
/**
* @param value The resource path for this method. Forward slashes (``/``) are encoded as ``~1`` and the initial slash must include a forward slash. For example, the path value ``/resource/subresource`` must be encoded as ``/~1resource~1subresource``. To specify the root path, use only a slash (``/``).
*/
@JvmName("kqdbnrvxugdahjos")
public suspend fun resourcePath(`value`: Output) {
this.resourcePath = value
}
/**
* @param value Specifies the throttling burst limit.
*/
@JvmName("nbutohqtdkqnbjmg")
public suspend fun throttlingBurstLimit(`value`: Output) {
this.throttlingBurstLimit = value
}
/**
* @param value Specifies the throttling rate limit.
*/
@JvmName("kfadrpswecufuhrt")
public suspend fun throttlingRateLimit(`value`: Output) {
this.throttlingRateLimit = value
}
/**
* @param value Specifies whether the cached responses are encrypted.
*/
@JvmName("hhhqbsixsbuqfvya")
public suspend fun cacheDataEncrypted(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cacheDataEncrypted = mapped
}
/**
* @param value Specifies the time to live (TTL), in seconds, for cached responses. The higher the TTL, the longer the response will be cached.
*/
@JvmName("bouaevbotbsqrinu")
public suspend fun cacheTtlInSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cacheTtlInSeconds = mapped
}
/**
* @param value Specifies whether responses should be cached and returned for requests. A cache cluster must be enabled on the stage for responses to be cached.
*/
@JvmName("oethfawntbynjfcr")
public suspend fun cachingEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cachingEnabled = mapped
}
/**
* @param value Specifies whether data trace logging is enabled for this method, which affects the log entries pushed to Amazon CloudWatch Logs. This can be useful to troubleshoot APIs, but can result in logging sensitive data. We recommend that you don't enable this option for production APIs.
*/
@JvmName("bqadhmbcppgvgntv")
public suspend fun dataTraceEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataTraceEnabled = mapped
}
/**
* @param value The HTTP method.
*/
@JvmName("riwiduryqisxbmac")
public suspend fun httpMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpMethod = mapped
}
/**
* @param value Specifies the logging level for this method, which affects the log entries pushed to Amazon CloudWatch Logs. Valid values are ``OFF``, ``ERROR``, and ``INFO``. Choose ``ERROR`` to write only error-level entries to CloudWatch Logs, or choose ``INFO`` to include all ``ERROR`` events as well as extra informational events.
*/
@JvmName("pgjufbmtejuthcsf")
public suspend fun loggingLevel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.loggingLevel = mapped
}
/**
* @param value Specifies whether Amazon CloudWatch metrics are enabled for this method.
*/
@JvmName("pafhrqxbiffsqlxk")
public suspend fun metricsEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricsEnabled = mapped
}
/**
* @param value The resource path for this method. Forward slashes (``/``) are encoded as ``~1`` and the initial slash must include a forward slash. For example, the path value ``/resource/subresource`` must be encoded as ``/~1resource~1subresource``. To specify the root path, use only a slash (``/``).
*/
@JvmName("wytxwiesqyqlynbk")
public suspend fun resourcePath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourcePath = mapped
}
/**
* @param value Specifies the throttling burst limit.
*/
@JvmName("vgwjlfeifbvlifrt")
public suspend fun throttlingBurstLimit(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.throttlingBurstLimit = mapped
}
/**
* @param value Specifies the throttling rate limit.
*/
@JvmName("qabammdsqvhcplal")
public suspend fun throttlingRateLimit(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.throttlingRateLimit = mapped
}
internal fun build(): DeploymentMethodSettingArgs = DeploymentMethodSettingArgs(
cacheDataEncrypted = cacheDataEncrypted,
cacheTtlInSeconds = cacheTtlInSeconds,
cachingEnabled = cachingEnabled,
dataTraceEnabled = dataTraceEnabled,
httpMethod = httpMethod,
loggingLevel = loggingLevel,
metricsEnabled = metricsEnabled,
resourcePath = resourcePath,
throttlingBurstLimit = throttlingBurstLimit,
throttlingRateLimit = throttlingRateLimit,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy