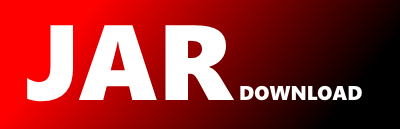
com.pulumi.awsnative.apigatewayv2.kotlin.Route.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.apigatewayv2.kotlin
import com.pulumi.awsnative.apigatewayv2.kotlin.outputs.RouteParameterConstraints
import com.pulumi.awsnative.apigatewayv2.kotlin.outputs.RouteParameterConstraints.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [Route].
*/
@PulumiTagMarker
public class RouteResourceBuilder internal constructor() {
public var name: String? = null
public var args: RouteArgs = RouteArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RouteArgsBuilder.() -> Unit) {
val builder = RouteArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Route {
val builtJavaResource = com.pulumi.awsnative.apigatewayv2.Route(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Route(builtJavaResource)
}
}
/**
* The ``AWS::ApiGatewayV2::Route`` resource creates a route for an API.
*/
public class Route internal constructor(
override val javaResource: com.pulumi.awsnative.apigatewayv2.Route,
) : KotlinCustomResource(javaResource, RouteMapper) {
/**
* The API identifier.
*/
public val apiId: Output
get() = javaResource.apiId().applyValue({ args0 -> args0 })
/**
* Specifies whether an API key is required for the route. Supported only for WebSocket APIs.
*/
public val apiKeyRequired: Output?
get() = javaResource.apiKeyRequired().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The authorization scopes supported by this route.
*/
public val authorizationScopes: Output>?
get() = javaResource.authorizationScopes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The authorization type for the route. For WebSocket APIs, valid values are ``NONE`` for open access, ``AWS_IAM`` for using AWS IAM permissions, and ``CUSTOM`` for using a Lambda authorizer. For HTTP APIs, valid values are ``NONE`` for open access, ``JWT`` for using JSON Web Tokens, ``AWS_IAM`` for using AWS IAM permissions, and ``CUSTOM`` for using a Lambda authorizer.
*/
public val authorizationType: Output?
get() = javaResource.authorizationType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The identifier of the ``Authorizer`` resource to be associated with this route. The authorizer identifier is generated by API Gateway when you created the authorizer.
*/
public val authorizerId: Output?
get() = javaResource.authorizerId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The model selection expression for the route. Supported only for WebSocket APIs.
*/
public val modelSelectionExpression: Output?
get() = javaResource.modelSelectionExpression().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The operation name for the route.
*/
public val operationName: Output?
get() = javaResource.operationName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The request models for the route. Supported only for WebSocket APIs.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::ApiGatewayV2::Route` for more information about the expected schema for this property.
*/
public val requestModels: Output?
get() = javaResource.requestModels().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The request parameters for the route. Supported only for WebSocket APIs.
*/
public val requestParameters: Output>?
get() = javaResource.requestParameters().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
}).orElse(null)
})
/**
* The route ID.
*/
public val routeId: Output
get() = javaResource.routeId().applyValue({ args0 -> args0 })
/**
* The route key for the route. For HTTP APIs, the route key can be either ``$default``, or a combination of an HTTP method and resource path, for example, ``GET /pets``.
*/
public val routeKey: Output
get() = javaResource.routeKey().applyValue({ args0 -> args0 })
/**
* The route response selection expression for the route. Supported only for WebSocket APIs.
*/
public val routeResponseSelectionExpression: Output?
get() = javaResource.routeResponseSelectionExpression().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The target for the route.
*/
public val target: Output?
get() = javaResource.target().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object RouteMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.apigatewayv2.Route::class == javaResource::class
override fun map(javaResource: Resource): Route = Route(
javaResource as
com.pulumi.awsnative.apigatewayv2.Route,
)
}
/**
* @see [Route].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Route].
*/
public suspend fun route(name: String, block: suspend RouteResourceBuilder.() -> Unit): Route {
val builder = RouteResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Route].
* @param name The _unique_ name of the resulting resource.
*/
public fun route(name: String): Route {
val builder = RouteResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy