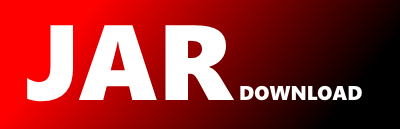
com.pulumi.awsnative.appconfig.kotlin.ConfigurationProfile.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appconfig.kotlin
import com.pulumi.awsnative.appconfig.kotlin.outputs.ConfigurationProfileValidators
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.appconfig.kotlin.outputs.ConfigurationProfileValidators.Companion.toKotlin as configurationProfileValidatorsToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [ConfigurationProfile].
*/
@PulumiTagMarker
public class ConfigurationProfileResourceBuilder internal constructor() {
public var name: String? = null
public var args: ConfigurationProfileArgs = ConfigurationProfileArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ConfigurationProfileArgsBuilder.() -> Unit) {
val builder = ConfigurationProfileArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ConfigurationProfile {
val builtJavaResource =
com.pulumi.awsnative.appconfig.ConfigurationProfile(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ConfigurationProfile(builtJavaResource)
}
}
/**
* An example resource schema demonstrating some basic constructs and validation rules.
*/
public class ConfigurationProfile internal constructor(
override val javaResource: com.pulumi.awsnative.appconfig.ConfigurationProfile,
) : KotlinCustomResource(javaResource, ConfigurationProfileMapper) {
/**
* The application ID.
*/
public val applicationId: Output
get() = javaResource.applicationId().applyValue({ args0 -> args0 })
/**
* The configuration profile ID
*/
public val configurationProfileId: Output
get() = javaResource.configurationProfileId().applyValue({ args0 -> args0 })
/**
* A description of the configuration profile.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Amazon Resource Name of the AWS Key Management Service key to encrypt new configuration data versions in the AWS AppConfig hosted configuration store. This attribute is only used for hosted configuration types. To encrypt data managed in other configuration stores, see the documentation for how to specify an AWS KMS key for that particular service.
*/
public val kmsKeyArn: Output
get() = javaResource.kmsKeyArn().applyValue({ args0 -> args0 })
/**
* The AWS Key Management Service key identifier (key ID, key alias, or key ARN) provided when the resource was created or updated.
*/
public val kmsKeyIdentifier: Output?
get() = javaResource.kmsKeyIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A URI to locate the configuration. You can specify the AWS AppConfig hosted configuration store, Systems Manager (SSM) document, an SSM Parameter Store parameter, or an Amazon S3 object.
*/
public val locationUri: Output
get() = javaResource.locationUri().applyValue({ args0 -> args0 })
/**
* A name for the configuration profile.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The ARN of an IAM role with permission to access the configuration at the specified LocationUri.
*/
public val retrievalRoleArn: Output?
get() = javaResource.retrievalRoleArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Metadata to assign to the configuration profile. Tags help organize and categorize your AWS AppConfig resources. Each tag consists of a key and an optional value, both of which you define.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The type of configurations contained in the profile. When calling this API, enter one of the following values for Type: AWS.AppConfig.FeatureFlags, AWS.Freeform
*/
public val type: Output?
get() = javaResource.type().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A list of methods for validating the configuration.
*/
public val validators: Output>?
get() = javaResource.validators().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> configurationProfileValidatorsToKotlin(args0) })
})
}).orElse(null)
})
}
public object ConfigurationProfileMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.appconfig.ConfigurationProfile::class == javaResource::class
override fun map(javaResource: Resource): ConfigurationProfile = ConfigurationProfile(
javaResource
as com.pulumi.awsnative.appconfig.ConfigurationProfile,
)
}
/**
* @see [ConfigurationProfile].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ConfigurationProfile].
*/
public suspend fun configurationProfile(
name: String,
block: suspend ConfigurationProfileResourceBuilder.() -> Unit,
): ConfigurationProfile {
val builder = ConfigurationProfileResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ConfigurationProfile].
* @param name The _unique_ name of the resulting resource.
*/
public fun configurationProfile(name: String): ConfigurationProfile {
val builder = ConfigurationProfileResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy