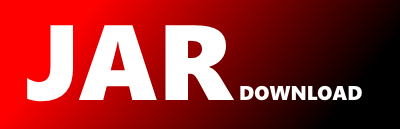
com.pulumi.awsnative.appflow.kotlin.ConnectorArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appflow.kotlin
import com.pulumi.awsnative.appflow.ConnectorArgs.builder
import com.pulumi.awsnative.appflow.kotlin.inputs.ConnectorProvisioningConfigArgs
import com.pulumi.awsnative.appflow.kotlin.inputs.ConnectorProvisioningConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::AppFlow::Connector
* @property connectorLabel The name of the connector. The name is unique for each ConnectorRegistration in your AWS account.
* @property connectorProvisioningConfig Contains information about the configuration of the connector being registered.
* @property connectorProvisioningType The provisioning type of the connector. Currently the only supported value is LAMBDA.
* @property description A description about the connector that's being registered.
*/
public data class ConnectorArgs(
public val connectorLabel: Output? = null,
public val connectorProvisioningConfig: Output? = null,
public val connectorProvisioningType: Output? = null,
public val description: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.appflow.ConnectorArgs =
com.pulumi.awsnative.appflow.ConnectorArgs.builder()
.connectorLabel(connectorLabel?.applyValue({ args0 -> args0 }))
.connectorProvisioningConfig(
connectorProvisioningConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.connectorProvisioningType(connectorProvisioningType?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectorArgs].
*/
@PulumiTagMarker
public class ConnectorArgsBuilder internal constructor() {
private var connectorLabel: Output? = null
private var connectorProvisioningConfig: Output? = null
private var connectorProvisioningType: Output? = null
private var description: Output? = null
/**
* @param value The name of the connector. The name is unique for each ConnectorRegistration in your AWS account.
*/
@JvmName("urotxbmraykswmwq")
public suspend fun connectorLabel(`value`: Output) {
this.connectorLabel = value
}
/**
* @param value Contains information about the configuration of the connector being registered.
*/
@JvmName("xncxlstcsshhnftx")
public suspend fun connectorProvisioningConfig(`value`: Output) {
this.connectorProvisioningConfig = value
}
/**
* @param value The provisioning type of the connector. Currently the only supported value is LAMBDA.
*/
@JvmName("guqxbjxewqxbncxn")
public suspend fun connectorProvisioningType(`value`: Output) {
this.connectorProvisioningType = value
}
/**
* @param value A description about the connector that's being registered.
*/
@JvmName("amvtnyemadksgldf")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The name of the connector. The name is unique for each ConnectorRegistration in your AWS account.
*/
@JvmName("igrilfifjlbrqfff")
public suspend fun connectorLabel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorLabel = mapped
}
/**
* @param value Contains information about the configuration of the connector being registered.
*/
@JvmName("hryblmbmribsfbyk")
public suspend fun connectorProvisioningConfig(`value`: ConnectorProvisioningConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorProvisioningConfig = mapped
}
/**
* @param argument Contains information about the configuration of the connector being registered.
*/
@JvmName("litqfisnwpgdcewl")
public suspend fun connectorProvisioningConfig(argument: suspend ConnectorProvisioningConfigArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProvisioningConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.connectorProvisioningConfig = mapped
}
/**
* @param value The provisioning type of the connector. Currently the only supported value is LAMBDA.
*/
@JvmName("bxxdhekwkkiixcop")
public suspend fun connectorProvisioningType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorProvisioningType = mapped
}
/**
* @param value A description about the connector that's being registered.
*/
@JvmName("soeaasnpeqknkjal")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
internal fun build(): ConnectorArgs = ConnectorArgs(
connectorLabel = connectorLabel,
connectorProvisioningConfig = connectorProvisioningConfig,
connectorProvisioningType = connectorProvisioningType,
description = description,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy