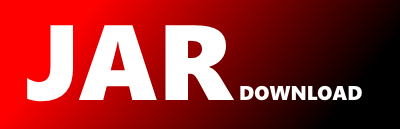
com.pulumi.awsnative.appflow.kotlin.ConnectorProfileArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appflow.kotlin
import com.pulumi.awsnative.appflow.ConnectorProfileArgs.builder
import com.pulumi.awsnative.appflow.kotlin.enums.ConnectorProfileConnectionMode
import com.pulumi.awsnative.appflow.kotlin.enums.ConnectorProfileConnectorType
import com.pulumi.awsnative.appflow.kotlin.inputs.ConnectorProfileConfigArgs
import com.pulumi.awsnative.appflow.kotlin.inputs.ConnectorProfileConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::AppFlow::ConnectorProfile
* @property connectionMode Mode in which data transfer should be enabled. Private connection mode is currently enabled for Salesforce, Snowflake, Trendmicro and Singular
* @property connectorLabel The label of the connector. The label is unique for each ConnectorRegistration in your AWS account. Only needed if calling for CUSTOMCONNECTOR connector type/.
* @property connectorProfileConfig Connector specific configurations needed to create connector profile
* @property connectorProfileName The maximum number of items to retrieve in a single batch.
* @property connectorType List of Saas providers that need connector profile to be created
* @property kmsArn The ARN of the AWS Key Management Service (AWS KMS) key that's used to encrypt your function's environment variables. If it's not provided, AWS Lambda uses a default service key.
*/
public data class ConnectorProfileArgs(
public val connectionMode: Output? = null,
public val connectorLabel: Output? = null,
public val connectorProfileConfig: Output? = null,
public val connectorProfileName: Output? = null,
public val connectorType: Output? = null,
public val kmsArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.appflow.ConnectorProfileArgs =
com.pulumi.awsnative.appflow.ConnectorProfileArgs.builder()
.connectionMode(connectionMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.connectorLabel(connectorLabel?.applyValue({ args0 -> args0 }))
.connectorProfileConfig(
connectorProfileConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.connectorProfileName(connectorProfileName?.applyValue({ args0 -> args0 }))
.connectorType(connectorType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kmsArn(kmsArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectorProfileArgs].
*/
@PulumiTagMarker
public class ConnectorProfileArgsBuilder internal constructor() {
private var connectionMode: Output? = null
private var connectorLabel: Output? = null
private var connectorProfileConfig: Output? = null
private var connectorProfileName: Output? = null
private var connectorType: Output? = null
private var kmsArn: Output? = null
/**
* @param value Mode in which data transfer should be enabled. Private connection mode is currently enabled for Salesforce, Snowflake, Trendmicro and Singular
*/
@JvmName("nnsftukpusydtfva")
public suspend fun connectionMode(`value`: Output) {
this.connectionMode = value
}
/**
* @param value The label of the connector. The label is unique for each ConnectorRegistration in your AWS account. Only needed if calling for CUSTOMCONNECTOR connector type/.
*/
@JvmName("tjnenkmjcqtuuulj")
public suspend fun connectorLabel(`value`: Output) {
this.connectorLabel = value
}
/**
* @param value Connector specific configurations needed to create connector profile
*/
@JvmName("hmaiwqrhddgywmuo")
public suspend fun connectorProfileConfig(`value`: Output) {
this.connectorProfileConfig = value
}
/**
* @param value The maximum number of items to retrieve in a single batch.
*/
@JvmName("cunqcfyxdrmpdeyu")
public suspend fun connectorProfileName(`value`: Output) {
this.connectorProfileName = value
}
/**
* @param value List of Saas providers that need connector profile to be created
*/
@JvmName("wncybvgxcnrgppek")
public suspend fun connectorType(`value`: Output) {
this.connectorType = value
}
/**
* @param value The ARN of the AWS Key Management Service (AWS KMS) key that's used to encrypt your function's environment variables. If it's not provided, AWS Lambda uses a default service key.
*/
@JvmName("djhpusmossivjqwr")
public suspend fun kmsArn(`value`: Output) {
this.kmsArn = value
}
/**
* @param value Mode in which data transfer should be enabled. Private connection mode is currently enabled for Salesforce, Snowflake, Trendmicro and Singular
*/
@JvmName("tvbgjxwsurikwwlo")
public suspend fun connectionMode(`value`: ConnectorProfileConnectionMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionMode = mapped
}
/**
* @param value The label of the connector. The label is unique for each ConnectorRegistration in your AWS account. Only needed if calling for CUSTOMCONNECTOR connector type/.
*/
@JvmName("fhwuftnyqxejmjhp")
public suspend fun connectorLabel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorLabel = mapped
}
/**
* @param value Connector specific configurations needed to create connector profile
*/
@JvmName("iasilhkmkotfuojs")
public suspend fun connectorProfileConfig(`value`: ConnectorProfileConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorProfileConfig = mapped
}
/**
* @param argument Connector specific configurations needed to create connector profile
*/
@JvmName("wrjcdofmgnradgbc")
public suspend fun connectorProfileConfig(argument: suspend ConnectorProfileConfigArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.connectorProfileConfig = mapped
}
/**
* @param value The maximum number of items to retrieve in a single batch.
*/
@JvmName("klhdoxisfxpgdcou")
public suspend fun connectorProfileName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorProfileName = mapped
}
/**
* @param value List of Saas providers that need connector profile to be created
*/
@JvmName("blyfmloinbvdxdgy")
public suspend fun connectorType(`value`: ConnectorProfileConnectorType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorType = mapped
}
/**
* @param value The ARN of the AWS Key Management Service (AWS KMS) key that's used to encrypt your function's environment variables. If it's not provided, AWS Lambda uses a default service key.
*/
@JvmName("qebtopdhlmucnlga")
public suspend fun kmsArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsArn = mapped
}
internal fun build(): ConnectorProfileArgs = ConnectorProfileArgs(
connectionMode = connectionMode,
connectorLabel = connectorLabel,
connectorProfileConfig = connectorProfileConfig,
connectorProfileName = connectorProfileName,
connectorType = connectorType,
kmsArn = kmsArn,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy