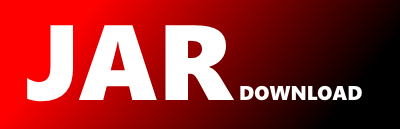
com.pulumi.awsnative.appflow.kotlin.inputs.ConnectorProfilePropertiesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appflow.kotlin.inputs
import com.pulumi.awsnative.appflow.inputs.ConnectorProfilePropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Connector specific properties needed to create connector profile - currently not needed for Amplitude, Trendmicro, Googleanalytics and Singular
* @property customConnector The properties required by the custom connector.
* @property datadog The connector-specific properties required by Datadog.
* @property dynatrace The connector-specific properties required by Dynatrace.
* @property inforNexus The connector-specific properties required by Infor Nexus.
* @property marketo The connector-specific properties required by Marketo.
* @property pardot The connector-specific properties required by Salesforce Pardot.
* @property redshift The connector-specific properties required by Amazon Redshift.
* @property salesforce The connector-specific properties required by Salesforce.
* @property sapoData The connector-specific profile properties required when using SAPOData.
* @property serviceNow The connector-specific properties required by serviceNow.
* @property slack The connector-specific properties required by Slack.
* @property snowflake The connector-specific properties required by Snowflake.
* @property veeva The connector-specific properties required by Veeva.
* @property zendesk The connector-specific properties required by Zendesk.
*/
public data class ConnectorProfilePropertiesArgs(
public val customConnector: Output? = null,
public val datadog: Output? = null,
public val dynatrace: Output? = null,
public val inforNexus: Output? = null,
public val marketo: Output? = null,
public val pardot: Output? = null,
public val redshift: Output? = null,
public val salesforce: Output? = null,
public val sapoData: Output? = null,
public val serviceNow: Output? = null,
public val slack: Output? = null,
public val snowflake: Output? = null,
public val veeva: Output? = null,
public val zendesk: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.appflow.inputs.ConnectorProfilePropertiesArgs =
com.pulumi.awsnative.appflow.inputs.ConnectorProfilePropertiesArgs.builder()
.customConnector(customConnector?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.datadog(datadog?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dynatrace(dynatrace?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.inforNexus(inforNexus?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.marketo(marketo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.pardot(pardot?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.redshift(redshift?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.salesforce(salesforce?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sapoData(sapoData?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.serviceNow(serviceNow?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.slack(slack?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.snowflake(snowflake?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.veeva(veeva?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.zendesk(zendesk?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ConnectorProfilePropertiesArgs].
*/
@PulumiTagMarker
public class ConnectorProfilePropertiesArgsBuilder internal constructor() {
private var customConnector: Output? = null
private var datadog: Output? = null
private var dynatrace: Output? = null
private var inforNexus: Output? = null
private var marketo: Output? = null
private var pardot: Output? = null
private var redshift: Output? = null
private var salesforce: Output? = null
private var sapoData: Output? = null
private var serviceNow: Output? = null
private var slack: Output? = null
private var snowflake: Output? = null
private var veeva: Output? = null
private var zendesk: Output? = null
/**
* @param value The properties required by the custom connector.
*/
@JvmName("mylljuueisvvgevt")
public suspend fun customConnector(`value`: Output) {
this.customConnector = value
}
/**
* @param value The connector-specific properties required by Datadog.
*/
@JvmName("ksrmgvvpfjvnxolx")
public suspend fun datadog(`value`: Output) {
this.datadog = value
}
/**
* @param value The connector-specific properties required by Dynatrace.
*/
@JvmName("biupmpwmtygbjxub")
public suspend fun dynatrace(`value`: Output) {
this.dynatrace = value
}
/**
* @param value The connector-specific properties required by Infor Nexus.
*/
@JvmName("ffqkdwuchsacuttw")
public suspend fun inforNexus(`value`: Output) {
this.inforNexus = value
}
/**
* @param value The connector-specific properties required by Marketo.
*/
@JvmName("uilthchjttcmcruh")
public suspend fun marketo(`value`: Output) {
this.marketo = value
}
/**
* @param value The connector-specific properties required by Salesforce Pardot.
*/
@JvmName("wcnpseqtyolrligw")
public suspend fun pardot(`value`: Output) {
this.pardot = value
}
/**
* @param value The connector-specific properties required by Amazon Redshift.
*/
@JvmName("edhcxushkemurkio")
public suspend fun redshift(`value`: Output) {
this.redshift = value
}
/**
* @param value The connector-specific properties required by Salesforce.
*/
@JvmName("cjwtwvrogvdeqccv")
public suspend fun salesforce(`value`: Output) {
this.salesforce = value
}
/**
* @param value The connector-specific profile properties required when using SAPOData.
*/
@JvmName("nxogwkwbuvulaqwj")
public suspend fun sapoData(`value`: Output) {
this.sapoData = value
}
/**
* @param value The connector-specific properties required by serviceNow.
*/
@JvmName("iibfhlmmqswqlhca")
public suspend fun serviceNow(`value`: Output) {
this.serviceNow = value
}
/**
* @param value The connector-specific properties required by Slack.
*/
@JvmName("rwldgwsypmllaqts")
public suspend fun slack(`value`: Output) {
this.slack = value
}
/**
* @param value The connector-specific properties required by Snowflake.
*/
@JvmName("vkpkhpvcqwggfpse")
public suspend fun snowflake(`value`: Output) {
this.snowflake = value
}
/**
* @param value The connector-specific properties required by Veeva.
*/
@JvmName("irjajsfracrcwtxe")
public suspend fun veeva(`value`: Output) {
this.veeva = value
}
/**
* @param value The connector-specific properties required by Zendesk.
*/
@JvmName("ysiqmnbiwraqykwx")
public suspend fun zendesk(`value`: Output) {
this.zendesk = value
}
/**
* @param value The properties required by the custom connector.
*/
@JvmName("afffmtlylabxjghy")
public suspend fun customConnector(`value`: ConnectorProfileCustomConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customConnector = mapped
}
/**
* @param argument The properties required by the custom connector.
*/
@JvmName("xlcklimgkochyncv")
public suspend fun customConnector(argument: suspend ConnectorProfileCustomConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileCustomConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customConnector = mapped
}
/**
* @param value The connector-specific properties required by Datadog.
*/
@JvmName("cetipphkgshpswwj")
public suspend fun datadog(`value`: ConnectorProfileDatadogConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datadog = mapped
}
/**
* @param argument The connector-specific properties required by Datadog.
*/
@JvmName("qlbnyhkiifinqulw")
public suspend fun datadog(argument: suspend ConnectorProfileDatadogConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileDatadogConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.datadog = mapped
}
/**
* @param value The connector-specific properties required by Dynatrace.
*/
@JvmName("ihcxuhxsyyomqdhu")
public suspend fun dynatrace(`value`: ConnectorProfileDynatraceConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dynatrace = mapped
}
/**
* @param argument The connector-specific properties required by Dynatrace.
*/
@JvmName("kcskwfsraicudclx")
public suspend fun dynatrace(argument: suspend ConnectorProfileDynatraceConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileDynatraceConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dynatrace = mapped
}
/**
* @param value The connector-specific properties required by Infor Nexus.
*/
@JvmName("qekdljcaxjojwalv")
public suspend fun inforNexus(`value`: ConnectorProfileInforNexusConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inforNexus = mapped
}
/**
* @param argument The connector-specific properties required by Infor Nexus.
*/
@JvmName("wtpypogufuhebrbc")
public suspend fun inforNexus(argument: suspend ConnectorProfileInforNexusConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileInforNexusConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.inforNexus = mapped
}
/**
* @param value The connector-specific properties required by Marketo.
*/
@JvmName("krvrdauvlxlajkyu")
public suspend fun marketo(`value`: ConnectorProfileMarketoConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.marketo = mapped
}
/**
* @param argument The connector-specific properties required by Marketo.
*/
@JvmName("utvfgjrnashowfpl")
public suspend fun marketo(argument: suspend ConnectorProfileMarketoConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileMarketoConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.marketo = mapped
}
/**
* @param value The connector-specific properties required by Salesforce Pardot.
*/
@JvmName("trohycwovseyyuhp")
public suspend fun pardot(`value`: ConnectorProfilePardotConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pardot = mapped
}
/**
* @param argument The connector-specific properties required by Salesforce Pardot.
*/
@JvmName("ilktkorbjwjyqblx")
public suspend fun pardot(argument: suspend ConnectorProfilePardotConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfilePardotConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.pardot = mapped
}
/**
* @param value The connector-specific properties required by Amazon Redshift.
*/
@JvmName("qcfebndxveavnngk")
public suspend fun redshift(`value`: ConnectorProfileRedshiftConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redshift = mapped
}
/**
* @param argument The connector-specific properties required by Amazon Redshift.
*/
@JvmName("ixajghdvmytccowa")
public suspend fun redshift(argument: suspend ConnectorProfileRedshiftConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileRedshiftConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.redshift = mapped
}
/**
* @param value The connector-specific properties required by Salesforce.
*/
@JvmName("krjlyetwuidvoilp")
public suspend fun salesforce(`value`: ConnectorProfileSalesforceConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.salesforce = mapped
}
/**
* @param argument The connector-specific properties required by Salesforce.
*/
@JvmName("aawnlsxtdgtjovdt")
public suspend fun salesforce(argument: suspend ConnectorProfileSalesforceConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileSalesforceConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.salesforce = mapped
}
/**
* @param value The connector-specific profile properties required when using SAPOData.
*/
@JvmName("aorajpgtkrstbkbx")
public suspend fun sapoData(`value`: ConnectorProfileSapoDataConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sapoData = mapped
}
/**
* @param argument The connector-specific profile properties required when using SAPOData.
*/
@JvmName("gvxnnuqulmyvejmn")
public suspend fun sapoData(argument: suspend ConnectorProfileSapoDataConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileSapoDataConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sapoData = mapped
}
/**
* @param value The connector-specific properties required by serviceNow.
*/
@JvmName("wfsjgnbmmceoeuqp")
public suspend fun serviceNow(`value`: ConnectorProfileServiceNowConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceNow = mapped
}
/**
* @param argument The connector-specific properties required by serviceNow.
*/
@JvmName("pvetqyjvxdhacxvj")
public suspend fun serviceNow(argument: suspend ConnectorProfileServiceNowConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileServiceNowConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.serviceNow = mapped
}
/**
* @param value The connector-specific properties required by Slack.
*/
@JvmName("oihmrxscmsnvkict")
public suspend fun slack(`value`: ConnectorProfileSlackConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.slack = mapped
}
/**
* @param argument The connector-specific properties required by Slack.
*/
@JvmName("vfytmoetknthwxhb")
public suspend fun slack(argument: suspend ConnectorProfileSlackConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileSlackConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.slack = mapped
}
/**
* @param value The connector-specific properties required by Snowflake.
*/
@JvmName("vwcebjbpyueblome")
public suspend fun snowflake(`value`: ConnectorProfileSnowflakeConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snowflake = mapped
}
/**
* @param argument The connector-specific properties required by Snowflake.
*/
@JvmName("eorgwmqecwqqbhxu")
public suspend fun snowflake(argument: suspend ConnectorProfileSnowflakeConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileSnowflakeConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.snowflake = mapped
}
/**
* @param value The connector-specific properties required by Veeva.
*/
@JvmName("iqullnrggabetlmm")
public suspend fun veeva(`value`: ConnectorProfileVeevaConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.veeva = mapped
}
/**
* @param argument The connector-specific properties required by Veeva.
*/
@JvmName("yeblwmqoaswpdita")
public suspend fun veeva(argument: suspend ConnectorProfileVeevaConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileVeevaConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.veeva = mapped
}
/**
* @param value The connector-specific properties required by Zendesk.
*/
@JvmName("dqqyyxosiqgxdoss")
public suspend fun zendesk(`value`: ConnectorProfileZendeskConnectorProfilePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zendesk = mapped
}
/**
* @param argument The connector-specific properties required by Zendesk.
*/
@JvmName("uugjtmqhtbvhyxtl")
public suspend fun zendesk(argument: suspend ConnectorProfileZendeskConnectorProfilePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorProfileZendeskConnectorProfilePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.zendesk = mapped
}
internal fun build(): ConnectorProfilePropertiesArgs = ConnectorProfilePropertiesArgs(
customConnector = customConnector,
datadog = datadog,
dynatrace = dynatrace,
inforNexus = inforNexus,
marketo = marketo,
pardot = pardot,
redshift = redshift,
salesforce = salesforce,
sapoData = sapoData,
serviceNow = serviceNow,
slack = slack,
snowflake = snowflake,
veeva = veeva,
zendesk = zendesk,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy