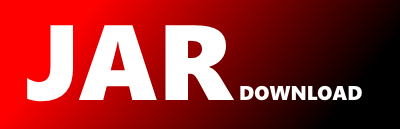
com.pulumi.awsnative.appflow.kotlin.inputs.FlowPrefixConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appflow.kotlin.inputs
import com.pulumi.awsnative.appflow.inputs.FlowPrefixConfigArgs.builder
import com.pulumi.awsnative.appflow.kotlin.enums.FlowPathPrefix
import com.pulumi.awsnative.appflow.kotlin.enums.FlowPrefixFormat
import com.pulumi.awsnative.appflow.kotlin.enums.FlowPrefixType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property pathPrefixHierarchy Specifies whether the destination file path includes either or both of the following elements:
* - **EXECUTION_ID** - The ID that Amazon AppFlow assigns to the flow run.
* - **SCHEMA_VERSION** - The version number of your data schema. Amazon AppFlow assigns this version number. The version number increases by one when you change any of the following settings in your flow configuration:
* - Source-to-destination field mappings
* - Field data types
* - Partition keys
* @property prefixFormat Determines the level of granularity for the date and time that's included in the prefix.
* @property prefixType Determines the format of the prefix, and whether it applies to the file name, file path, or both.
*/
public data class FlowPrefixConfigArgs(
public val pathPrefixHierarchy: Output>? = null,
public val prefixFormat: Output? = null,
public val prefixType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.appflow.inputs.FlowPrefixConfigArgs =
com.pulumi.awsnative.appflow.inputs.FlowPrefixConfigArgs.builder()
.pathPrefixHierarchy(
pathPrefixHierarchy?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.prefixFormat(prefixFormat?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.prefixType(prefixType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [FlowPrefixConfigArgs].
*/
@PulumiTagMarker
public class FlowPrefixConfigArgsBuilder internal constructor() {
private var pathPrefixHierarchy: Output>? = null
private var prefixFormat: Output? = null
private var prefixType: Output? = null
/**
* @param value Specifies whether the destination file path includes either or both of the following elements:
* - **EXECUTION_ID** - The ID that Amazon AppFlow assigns to the flow run.
* - **SCHEMA_VERSION** - The version number of your data schema. Amazon AppFlow assigns this version number. The version number increases by one when you change any of the following settings in your flow configuration:
* - Source-to-destination field mappings
* - Field data types
* - Partition keys
*/
@JvmName("ciymusbekyhaioqr")
public suspend fun pathPrefixHierarchy(`value`: Output>) {
this.pathPrefixHierarchy = value
}
@JvmName("lpifvdnhevinidik")
public suspend fun pathPrefixHierarchy(vararg values: Output) {
this.pathPrefixHierarchy = Output.all(values.asList())
}
/**
* @param values Specifies whether the destination file path includes either or both of the following elements:
* - **EXECUTION_ID** - The ID that Amazon AppFlow assigns to the flow run.
* - **SCHEMA_VERSION** - The version number of your data schema. Amazon AppFlow assigns this version number. The version number increases by one when you change any of the following settings in your flow configuration:
* - Source-to-destination field mappings
* - Field data types
* - Partition keys
*/
@JvmName("iwhyolftrjllhgcj")
public suspend fun pathPrefixHierarchy(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy