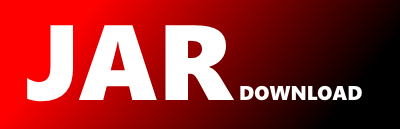
com.pulumi.awsnative.appflow.kotlin.inputs.FlowSourceFlowConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appflow.kotlin.inputs
import com.pulumi.awsnative.appflow.inputs.FlowSourceFlowConfigArgs.builder
import com.pulumi.awsnative.appflow.kotlin.enums.FlowConnectorType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Configurations of Source connector of the flow.
* @property apiVersion The API version that the destination connector uses.
* @property connectorProfileName Name of source connector profile
* @property connectorType Type of source connector
* @property incrementalPullConfig Configuration for scheduled incremental data pull
* @property sourceConnectorProperties Source connector details required to query a connector
*/
public data class FlowSourceFlowConfigArgs(
public val apiVersion: Output? = null,
public val connectorProfileName: Output? = null,
public val connectorType: Output,
public val incrementalPullConfig: Output? = null,
public val sourceConnectorProperties: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.appflow.inputs.FlowSourceFlowConfigArgs =
com.pulumi.awsnative.appflow.inputs.FlowSourceFlowConfigArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.connectorProfileName(connectorProfileName?.applyValue({ args0 -> args0 }))
.connectorType(connectorType.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.incrementalPullConfig(
incrementalPullConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sourceConnectorProperties(
sourceConnectorProperties.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [FlowSourceFlowConfigArgs].
*/
@PulumiTagMarker
public class FlowSourceFlowConfigArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var connectorProfileName: Output? = null
private var connectorType: Output? = null
private var incrementalPullConfig: Output? = null
private var sourceConnectorProperties: Output? = null
/**
* @param value The API version that the destination connector uses.
*/
@JvmName("snjfpgebijurdegj")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Name of source connector profile
*/
@JvmName("fjlblpsullyfilrn")
public suspend fun connectorProfileName(`value`: Output) {
this.connectorProfileName = value
}
/**
* @param value Type of source connector
*/
@JvmName("qjsomsijldrwqfji")
public suspend fun connectorType(`value`: Output) {
this.connectorType = value
}
/**
* @param value Configuration for scheduled incremental data pull
*/
@JvmName("snvptkimcrdkecnw")
public suspend fun incrementalPullConfig(`value`: Output) {
this.incrementalPullConfig = value
}
/**
* @param value Source connector details required to query a connector
*/
@JvmName("hrkrbqovlwaigdja")
public suspend fun sourceConnectorProperties(`value`: Output) {
this.sourceConnectorProperties = value
}
/**
* @param value The API version that the destination connector uses.
*/
@JvmName("kpkmfvcnxydgndhe")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Name of source connector profile
*/
@JvmName("gsakkfvshydahjfr")
public suspend fun connectorProfileName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorProfileName = mapped
}
/**
* @param value Type of source connector
*/
@JvmName("qrrcosawjbehkhok")
public suspend fun connectorType(`value`: FlowConnectorType) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.connectorType = mapped
}
/**
* @param value Configuration for scheduled incremental data pull
*/
@JvmName("acylfrqpqxrpsdno")
public suspend fun incrementalPullConfig(`value`: FlowIncrementalPullConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.incrementalPullConfig = mapped
}
/**
* @param argument Configuration for scheduled incremental data pull
*/
@JvmName("kfoaohgynperlcew")
public suspend fun incrementalPullConfig(argument: suspend FlowIncrementalPullConfigArgsBuilder.() -> Unit) {
val toBeMapped = FlowIncrementalPullConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.incrementalPullConfig = mapped
}
/**
* @param value Source connector details required to query a connector
*/
@JvmName("dkdqghlkcpkgsspk")
public suspend fun sourceConnectorProperties(`value`: FlowSourceConnectorPropertiesArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceConnectorProperties = mapped
}
/**
* @param argument Source connector details required to query a connector
*/
@JvmName("yokmegenmftiqgog")
public suspend fun sourceConnectorProperties(argument: suspend FlowSourceConnectorPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = FlowSourceConnectorPropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sourceConnectorProperties = mapped
}
internal fun build(): FlowSourceFlowConfigArgs = FlowSourceFlowConfigArgs(
apiVersion = apiVersion,
connectorProfileName = connectorProfileName,
connectorType = connectorType ?: throw PulumiNullFieldException("connectorType"),
incrementalPullConfig = incrementalPullConfig,
sourceConnectorProperties = sourceConnectorProperties ?: throw
PulumiNullFieldException("sourceConnectorProperties"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy