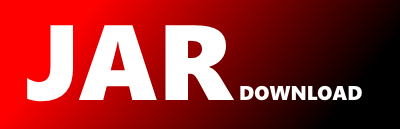
com.pulumi.awsnative.appintegrations.kotlin.DataIntegrationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appintegrations.kotlin
import com.pulumi.awsnative.appintegrations.DataIntegrationArgs.builder
import com.pulumi.awsnative.appintegrations.kotlin.inputs.DataIntegrationFileConfigurationArgs
import com.pulumi.awsnative.appintegrations.kotlin.inputs.DataIntegrationFileConfigurationArgsBuilder
import com.pulumi.awsnative.appintegrations.kotlin.inputs.DataIntegrationScheduleConfigArgs
import com.pulumi.awsnative.appintegrations.kotlin.inputs.DataIntegrationScheduleConfigArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::AppIntegrations::DataIntegration
* @property description The data integration description.
* @property fileConfiguration The configuration for what files should be pulled from the source.
* @property kmsKey The KMS key of the data integration.
* @property name The name of the data integration.
* @property objectConfiguration The configuration for what data should be pulled from the source.
* @property scheduleConfig The name of the data and how often it should be pulled from the source.
* @property sourceUri The URI of the data source.
* @property tags The tags (keys and values) associated with the data integration.
*/
public data class DataIntegrationArgs(
public val description: Output? = null,
public val fileConfiguration: Output? = null,
public val kmsKey: Output? = null,
public val name: Output? = null,
public val objectConfiguration: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy