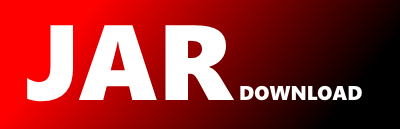
com.pulumi.awsnative.applicationautoscaling.kotlin.ApplicationautoscalingFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.applicationautoscaling.kotlin
import com.pulumi.awsnative.applicationautoscaling.ApplicationautoscalingFunctions.getScalableTargetPlain
import com.pulumi.awsnative.applicationautoscaling.ApplicationautoscalingFunctions.getScalingPolicyPlain
import com.pulumi.awsnative.applicationautoscaling.kotlin.inputs.GetScalableTargetPlainArgs
import com.pulumi.awsnative.applicationautoscaling.kotlin.inputs.GetScalableTargetPlainArgsBuilder
import com.pulumi.awsnative.applicationautoscaling.kotlin.inputs.GetScalingPolicyPlainArgs
import com.pulumi.awsnative.applicationautoscaling.kotlin.inputs.GetScalingPolicyPlainArgsBuilder
import com.pulumi.awsnative.applicationautoscaling.kotlin.outputs.GetScalableTargetResult
import com.pulumi.awsnative.applicationautoscaling.kotlin.outputs.GetScalingPolicyResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.applicationautoscaling.kotlin.outputs.GetScalableTargetResult.Companion.toKotlin as getScalableTargetResultToKotlin
import com.pulumi.awsnative.applicationautoscaling.kotlin.outputs.GetScalingPolicyResult.Companion.toKotlin as getScalingPolicyResultToKotlin
public object ApplicationautoscalingFunctions {
/**
* The ``AWS::ApplicationAutoScaling::ScalableTarget`` resource specifies a resource that Application Auto Scaling can scale, such as an AWS::DynamoDB::Table or AWS::ECS::Service resource.
* For more information, see [Getting started](https://docs.aws.amazon.com/autoscaling/application/userguide/getting-started.html) in the *Application Auto Scaling User Guide*.
* If the resource that you want Application Auto Scaling to scale is not yet created in your account, add a dependency on the resource when registering it as a scalable target using the [DependsOn](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-attribute-dependson.html) attribute.
* @param argument null
* @return null
*/
public suspend fun getScalableTarget(argument: GetScalableTargetPlainArgs): GetScalableTargetResult =
getScalableTargetResultToKotlin(getScalableTargetPlain(argument.toJava()).await())
/**
* @see [getScalableTarget].
* @param resourceId The identifier of the resource associated with the scalable target. This string consists of the resource type and unique identifier.
* + ECS service - The resource type is ``service`` and the unique identifier is the cluster name and service name. Example: ``service/my-cluster/my-service``.
* + Spot Fleet - The resource type is ``spot-fleet-request`` and the unique identifier is the Spot Fleet request ID. Example: ``spot-fleet-request/sfr-73fbd2ce-aa30-494c-8788-1cee4EXAMPLE``.
* + EMR cluster - The resource type is ``instancegroup`` and the unique identifier is the cluster ID and instance group ID. Example: ``instancegroup/j-2EEZNYKUA1NTV/ig-1791Y4E1L8YI0``.
* + AppStream 2.0 fleet - The resource type is ``fleet`` and the unique identifier is the fleet name. Example: ``fleet/sample-fleet``.
* + DynamoDB table - The resource type is ``table`` and the unique identifier is the table name. Example: ``table/my-table``.
* + DynamoDB global secondary index - The resource type is ``index`` and the unique identifier is the index name. Example: ``table/my-table/index/my-table-index``.
* + Aurora DB cluster - The resource type is ``cluster`` and the unique identifier is the cluster name. Example: ``cluster:my-db-cluster``.
* + SageMaker endpoint variant - The resource type is ``variant`` and the unique identifier is the resource ID. Example: ``endpoint/my-end-point/variant/KMeansClustering``.
* + Custom resources are not supported with a resource type. This parameter must specify the ``OutputValue`` from the CloudFormation template stack used to access the resources. The unique identifier is defined by the service provider. More information is available in our [GitHub repository](https://docs.aws.amazon.com/https://github.com/aws/aws-auto-scaling-custom-resource).
* + Amazon Comprehend document classification endpoint - The resource type and unique identifier are specified using the endpoint ARN. Example: ``arn:aws:comprehend:us-west-2:123456789012:document-classifier-endpoint/EXAMPLE``.
* + Amazon Comprehend entity recognizer endpoint - The resource type and unique identifier are specified using the endpoint ARN. Example: ``arn:aws:comprehend:us-west-2:123456789012:entity-recognizer-endpoint/EXAMPLE``.
* + Lambda provisioned concurrency - The resource type is ``function`` and the unique identifier is the function name with a function version or alias name suffix that is not ``$LATEST``. Example: ``function:my-function:prod`` or ``function:my-function:1``.
* + Amazon Keyspaces table - The resource type is ``table`` and the unique identifier is the table name. Example: ``keyspace/mykeyspace/table/mytable``.
* + Amazon MSK cluster - The resource type and unique identifier are specified using the cluster ARN. Example: ``arn:aws:kafka:us-east-1:123456789012:cluster/demo-cluster-1/6357e0b2-0e6a-4b86-a0b4-70df934c2e31-5``.
* + Amazon ElastiCache replication group - The resource type is ``replication-group`` and the unique identifier is the replication group name. Example: ``replication-group/mycluster``.
* + Neptune cluster - The resource type is ``cluster`` and the unique identifier is the cluster name. Example: ``cluster:mycluster``.
* + SageMaker serverless endpoint - The resource type is ``variant`` and the unique identifier is the resource ID. Example: ``endpoint/my-end-point/variant/KMeansClustering``.
* + SageMaker inference component - The resource type is ``inference-component`` and the unique identifier is the resource ID. Example: ``inference-component/my-inference-component``.
* @param scalableDimension The scalable dimension associated with the scalable target. This string consists of the service namespace, resource type, and scaling property.
* + ``ecs:service:DesiredCount`` - The desired task count of an ECS service.
* + ``elasticmapreduce:instancegroup:InstanceCount`` - The instance count of an EMR Instance Group.
* + ``ec2:spot-fleet-request:TargetCapacity`` - The target capacity of a Spot Fleet.
* + ``appstream:fleet:DesiredCapacity`` - The desired capacity of an AppStream 2.0 fleet.
* + ``dynamodb:table:ReadCapacityUnits`` - The provisioned read capacity for a DynamoDB table.
* + ``dynamodb:table:WriteCapacityUnits`` - The provisioned write capacity for a DynamoDB table.
* + ``dynamodb:index:ReadCapacityUnits`` - The provisioned read capacity for a DynamoDB global secondary index.
* + ``dynamodb:index:WriteCapacityUnits`` - The provisioned write capacity for a DynamoDB global secondary index.
* + ``rds:cluster:ReadReplicaCount`` - The count of Aurora Replicas in an Aurora DB cluster. Available for Aurora MySQL-compatible edition and Aurora PostgreSQL-compatible edition.
* + ``sagemaker:variant:DesiredInstanceCount`` - The number of EC2 instances for a SageMaker model endpoint variant.
* + ``custom-resource:ResourceType:Property`` - The scalable dimension for a custom resource provided by your own application or service.
* + ``comprehend:document-classifier-endpoint:DesiredInferenceUnits`` - The number of inference units for an Amazon Comprehend document classification endpoint.
* + ``comprehend:entity-recognizer-endpoint:DesiredInferenceUnits`` - The number of inference units for an Amazon Comprehend entity recognizer endpoint.
* + ``lambda:function:ProvisionedConcurrency`` - The provisioned concurrency for a Lambda function.
* + ``cassandra:table:ReadCapacityUnits`` - The provisioned read capacity for an Amazon Keyspaces table.
* + ``cassandra:table:WriteCapacityUnits`` - The provisioned write capacity for an Amazon Keyspaces table.
* + ``kafka:broker-storage:VolumeSize`` - The provisioned volume size (in GiB) for brokers in an Amazon MSK cluster.
* + ``elasticache:replication-group:NodeGroups`` - The number of node groups for an Amazon ElastiCache replication group.
* + ``elasticache:replication-group:Replicas`` - The number of replicas per node group for an Amazon ElastiCache replication group.
* + ``neptune:cluster:ReadReplicaCount`` - The count of read replicas in an Amazon Neptune DB cluster.
* + ``sagemaker:variant:DesiredProvisionedConcurrency`` - The provisioned concurrency for a SageMaker serverless endpoint.
* + ``sagemaker:inference-component:DesiredCopyCount`` - The number of copies across an endpoint for a SageMaker inference component.
* @param serviceNamespace The namespace of the AWS service that provides the resource, or a ``custom-resource``.
* @return null
*/
public suspend fun getScalableTarget(
resourceId: String,
scalableDimension: String,
serviceNamespace: String,
): GetScalableTargetResult {
val argument = GetScalableTargetPlainArgs(
resourceId = resourceId,
scalableDimension = scalableDimension,
serviceNamespace = serviceNamespace,
)
return getScalableTargetResultToKotlin(getScalableTargetPlain(argument.toJava()).await())
}
/**
* @see [getScalableTarget].
* @param argument Builder for [com.pulumi.awsnative.applicationautoscaling.kotlin.inputs.GetScalableTargetPlainArgs].
* @return null
*/
public suspend fun getScalableTarget(argument: suspend GetScalableTargetPlainArgsBuilder.() -> Unit): GetScalableTargetResult {
val builder = GetScalableTargetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getScalableTargetResultToKotlin(getScalableTargetPlain(builtArgument.toJava()).await())
}
/**
* The ``AWS::ApplicationAutoScaling::ScalingPolicy`` resource defines a scaling policy that Application Auto Scaling uses to adjust the capacity of a scalable target.
* For more information, see [Target tracking scaling policies](https://docs.aws.amazon.com/autoscaling/application/userguide/application-auto-scaling-target-tracking.html) and [Step scaling policies](https://docs.aws.amazon.com/autoscaling/application/userguide/application-auto-scaling-step-scaling-policies.html) in the *Application Auto Scaling User Guide*.
* @param argument null
* @return null
*/
public suspend fun getScalingPolicy(argument: GetScalingPolicyPlainArgs): GetScalingPolicyResult =
getScalingPolicyResultToKotlin(getScalingPolicyPlain(argument.toJava()).await())
/**
* @see [getScalingPolicy].
* @param arn Returns the ARN of a scaling policy.
* @param scalableDimension The scalable dimension. This string consists of the service namespace, resource type, and scaling property.
* + ``ecs:service:DesiredCount`` - The task count of an ECS service.
* + ``elasticmapreduce:instancegroup:InstanceCount`` - The instance count of an EMR Instance Group.
* + ``ec2:spot-fleet-request:TargetCapacity`` - The target capacity of a Spot Fleet.
* + ``appstream:fleet:DesiredCapacity`` - The capacity of an AppStream 2.0 fleet.
* + ``dynamodb:table:ReadCapacityUnits`` - The provisioned read capacity for a DynamoDB table.
* + ``dynamodb:table:WriteCapacityUnits`` - The provisioned write capacity for a DynamoDB table.
* + ``dynamodb:index:ReadCapacityUnits`` - The provisioned read capacity for a DynamoDB global secondary index.
* + ``dynamodb:index:WriteCapacityUnits`` - The provisioned write capacity for a DynamoDB global secondary index.
* + ``rds:cluster:ReadReplicaCount`` - The count of Aurora Replicas in an Aurora DB cluster. Available for Aurora MySQL-compatible edition and Aurora PostgreSQL-compatible edition.
* + ``sagemaker:variant:DesiredInstanceCount`` - The number of EC2 instances for a SageMaker model endpoint variant.
* + ``custom-resource:ResourceType:Property`` - The scalable dimension for a custom resource provided by your own application or service.
* + ``comprehend:document-classifier-endpoint:DesiredInferenceUnits`` - The number of inference units for an Amazon Comprehend document classification endpoint.
* + ``comprehend:entity-recognizer-endpoint:DesiredInferenceUnits`` - The number of inference units for an Amazon Comprehend entity recognizer endpoint.
* + ``lambda:function:ProvisionedConcurrency`` - The provisioned concurrency for a Lambda function.
* + ``cassandra:table:ReadCapacityUnits`` - The provisioned read capacity for an Amazon Keyspaces table.
* + ``cassandra:table:WriteCapacityUnits`` - The provisioned write capacity for an Amazon Keyspaces table.
* + ``kafka:broker-storage:VolumeSize`` - The provisioned volume size (in GiB) for brokers in an Amazon MSK cluster.
* + ``elasticache:replication-group:NodeGroups`` - The number of node groups for an Amazon ElastiCache replication group.
* + ``elasticache:replication-group:Replicas`` - The number of replicas per node group for an Amazon ElastiCache replication group.
* + ``neptune:cluster:ReadReplicaCount`` - The count of read replicas in an Amazon Neptune DB cluster.
* + ``sagemaker:variant:DesiredProvisionedConcurrency`` - The provisioned concurrency for a SageMaker serverless endpoint.
* + ``sagemaker:inference-component:DesiredCopyCount`` - The number of copies across an endpoint for a SageMaker inference component.
* + ``workspaces:workspacespool:DesiredUserSessions`` - The number of user sessions for the WorkSpaces in the pool.
* @return null
*/
public suspend fun getScalingPolicy(arn: String, scalableDimension: String): GetScalingPolicyResult {
val argument = GetScalingPolicyPlainArgs(
arn = arn,
scalableDimension = scalableDimension,
)
return getScalingPolicyResultToKotlin(getScalingPolicyPlain(argument.toJava()).await())
}
/**
* @see [getScalingPolicy].
* @param argument Builder for [com.pulumi.awsnative.applicationautoscaling.kotlin.inputs.GetScalingPolicyPlainArgs].
* @return null
*/
public suspend fun getScalingPolicy(argument: suspend GetScalingPolicyPlainArgsBuilder.() -> Unit): GetScalingPolicyResult {
val builder = GetScalingPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getScalingPolicyResultToKotlin(getScalingPolicyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy