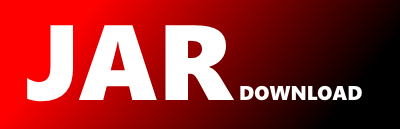
com.pulumi.awsnative.applicationinsights.kotlin.inputs.ApplicationLogArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.applicationinsights.kotlin.inputs
import com.pulumi.awsnative.applicationinsights.inputs.ApplicationLogArgs.builder
import com.pulumi.awsnative.applicationinsights.kotlin.enums.ApplicationLogEncoding
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A log to be monitored for the component.
* @property encoding The type of encoding of the logs to be monitored.
* @property logGroupName The CloudWatch log group name to be associated to the monitored log.
* @property logPath The path of the logs to be monitored.
* @property logType The log type decides the log patterns against which Application Insights analyzes the log.
* @property patternSet The name of the log pattern set.
*/
public data class ApplicationLogArgs(
public val encoding: Output? = null,
public val logGroupName: Output? = null,
public val logPath: Output? = null,
public val logType: Output,
public val patternSet: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.applicationinsights.inputs.ApplicationLogArgs =
com.pulumi.awsnative.applicationinsights.inputs.ApplicationLogArgs.builder()
.encoding(encoding?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.logGroupName(logGroupName?.applyValue({ args0 -> args0 }))
.logPath(logPath?.applyValue({ args0 -> args0 }))
.logType(logType.applyValue({ args0 -> args0 }))
.patternSet(patternSet?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApplicationLogArgs].
*/
@PulumiTagMarker
public class ApplicationLogArgsBuilder internal constructor() {
private var encoding: Output? = null
private var logGroupName: Output? = null
private var logPath: Output? = null
private var logType: Output? = null
private var patternSet: Output? = null
/**
* @param value The type of encoding of the logs to be monitored.
*/
@JvmName("rqbtguveivddxgkm")
public suspend fun encoding(`value`: Output) {
this.encoding = value
}
/**
* @param value The CloudWatch log group name to be associated to the monitored log.
*/
@JvmName("usujnkbvjmbqqhsh")
public suspend fun logGroupName(`value`: Output) {
this.logGroupName = value
}
/**
* @param value The path of the logs to be monitored.
*/
@JvmName("waswjpqmqvfnunhs")
public suspend fun logPath(`value`: Output) {
this.logPath = value
}
/**
* @param value The log type decides the log patterns against which Application Insights analyzes the log.
*/
@JvmName("qtpqjagilwxuboha")
public suspend fun logType(`value`: Output) {
this.logType = value
}
/**
* @param value The name of the log pattern set.
*/
@JvmName("xeorxkqduwsdwfpn")
public suspend fun patternSet(`value`: Output) {
this.patternSet = value
}
/**
* @param value The type of encoding of the logs to be monitored.
*/
@JvmName("asljftkxsgrkpdbc")
public suspend fun encoding(`value`: ApplicationLogEncoding?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encoding = mapped
}
/**
* @param value The CloudWatch log group name to be associated to the monitored log.
*/
@JvmName("mmksjhnxeoxbdipd")
public suspend fun logGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logGroupName = mapped
}
/**
* @param value The path of the logs to be monitored.
*/
@JvmName("widaimdrrjvmxmfh")
public suspend fun logPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logPath = mapped
}
/**
* @param value The log type decides the log patterns against which Application Insights analyzes the log.
*/
@JvmName("hnkmvfoouwljmxpy")
public suspend fun logType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.logType = mapped
}
/**
* @param value The name of the log pattern set.
*/
@JvmName("hwqpujfmylughwlq")
public suspend fun patternSet(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.patternSet = mapped
}
internal fun build(): ApplicationLogArgs = ApplicationLogArgs(
encoding = encoding,
logGroupName = logGroupName,
logPath = logPath,
logType = logType ?: throw PulumiNullFieldException("logType"),
patternSet = patternSet,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy