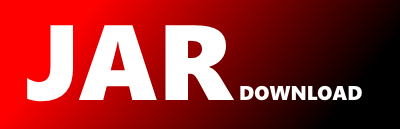
com.pulumi.awsnative.apprunner.kotlin.inputs.ServiceCodeConfigurationValuesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.apprunner.kotlin.inputs
import com.pulumi.awsnative.apprunner.inputs.ServiceCodeConfigurationValuesArgs.builder
import com.pulumi.awsnative.apprunner.kotlin.enums.ServiceCodeConfigurationValuesRuntime
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Code Configuration Values
* @property buildCommand Build Command
* @property port Port
* @property runtime Runtime
* @property runtimeEnvironmentSecrets The secrets and parameters that get referenced by your service as environment variables
* @property runtimeEnvironmentVariables The environment variables that are available to your running AWS App Runner service. An array of key-value pairs.
* @property startCommand Start Command
*/
public data class ServiceCodeConfigurationValuesArgs(
public val buildCommand: Output? = null,
public val port: Output? = null,
public val runtime: Output,
public val runtimeEnvironmentSecrets: Output>? = null,
public val runtimeEnvironmentVariables: Output>? = null,
public val startCommand: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.apprunner.inputs.ServiceCodeConfigurationValuesArgs =
com.pulumi.awsnative.apprunner.inputs.ServiceCodeConfigurationValuesArgs.builder()
.buildCommand(buildCommand?.applyValue({ args0 -> args0 }))
.port(port?.applyValue({ args0 -> args0 }))
.runtime(runtime.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.runtimeEnvironmentSecrets(
runtimeEnvironmentSecrets?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.runtimeEnvironmentVariables(
runtimeEnvironmentVariables?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.startCommand(startCommand?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceCodeConfigurationValuesArgs].
*/
@PulumiTagMarker
public class ServiceCodeConfigurationValuesArgsBuilder internal constructor() {
private var buildCommand: Output? = null
private var port: Output? = null
private var runtime: Output? = null
private var runtimeEnvironmentSecrets: Output>? = null
private var runtimeEnvironmentVariables: Output>? = null
private var startCommand: Output? = null
/**
* @param value Build Command
*/
@JvmName("smmbcskujjcpmugl")
public suspend fun buildCommand(`value`: Output) {
this.buildCommand = value
}
/**
* @param value Port
*/
@JvmName("uqqcbnrjdqpfoiye")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value Runtime
*/
@JvmName("qgthehvmtnnrvwbj")
public suspend fun runtime(`value`: Output) {
this.runtime = value
}
/**
* @param value The secrets and parameters that get referenced by your service as environment variables
*/
@JvmName("gamqkevrfawqtwui")
public suspend fun runtimeEnvironmentSecrets(`value`: Output>) {
this.runtimeEnvironmentSecrets = value
}
@JvmName("nvruiakfwexifvql")
public suspend fun runtimeEnvironmentSecrets(vararg values: Output) {
this.runtimeEnvironmentSecrets = Output.all(values.asList())
}
/**
* @param values The secrets and parameters that get referenced by your service as environment variables
*/
@JvmName("kmohyoxxonjdebqh")
public suspend fun runtimeEnvironmentSecrets(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy