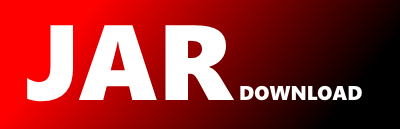
com.pulumi.awsnative.appsync.kotlin.Resolver.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.appsync.kotlin
import com.pulumi.awsnative.appsync.kotlin.enums.ResolverMetricsConfig
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverAppSyncRuntime
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverCachingConfig
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverPipelineConfig
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverSyncConfig
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.appsync.kotlin.enums.ResolverMetricsConfig.Companion.toKotlin as resolverMetricsConfigToKotlin
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverAppSyncRuntime.Companion.toKotlin as resolverAppSyncRuntimeToKotlin
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverCachingConfig.Companion.toKotlin as resolverCachingConfigToKotlin
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverPipelineConfig.Companion.toKotlin as resolverPipelineConfigToKotlin
import com.pulumi.awsnative.appsync.kotlin.outputs.ResolverSyncConfig.Companion.toKotlin as resolverSyncConfigToKotlin
/**
* Builder for [Resolver].
*/
@PulumiTagMarker
public class ResolverResourceBuilder internal constructor() {
public var name: String? = null
public var args: ResolverArgs = ResolverArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ResolverArgsBuilder.() -> Unit) {
val builder = ResolverArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Resolver {
val builtJavaResource = com.pulumi.awsnative.appsync.Resolver(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Resolver(builtJavaResource)
}
}
/**
* The ``AWS::AppSync::Resolver`` resource defines the logical GraphQL resolver that you attach to fields in a schema. Request and response templates for resolvers are written in Apache Velocity Template Language (VTL) format. For more information about resolvers, see [Resolver Mapping Template Reference](https://docs.aws.amazon.com/appsync/latest/devguide/resolver-mapping-template-reference.html).
* When you submit an update, CFNLong updates resources based on differences between what you submit and the stack's current template. To cause this resource to be updated you must change a property value for this resource in the CFNshort template. Changing the S3 file content without changing a property value will not result in an update operation.
* See [Update Behaviors of Stack Resources](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/using-cfn-updating-stacks-update-behaviors.html) in the *User Guide*.
* ## Example Usage
* ### Example
* No Java example available.
*/
public class Resolver internal constructor(
override val javaResource: com.pulumi.awsnative.appsync.Resolver,
) : KotlinCustomResource(javaResource, ResolverMapper) {
/**
* The APSYlong GraphQL API to which you want to attach this resolver.
*/
public val apiId: Output
get() = javaResource.apiId().applyValue({ args0 -> args0 })
/**
* The caching configuration for the resolver.
*/
public val cachingConfig: Output?
get() = javaResource.cachingConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> resolverCachingConfigToKotlin(args0) })
}).orElse(null)
})
/**
* The ``resolver`` code that contains the request and response functions. When code is used, the ``runtime`` is required. The runtime value must be ``APPSYNC_JS``.
*/
public val code: Output?
get() = javaResource.code().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The Amazon S3 endpoint.
*/
public val codeS3Location: Output?
get() = javaResource.codeS3Location().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The resolver data source name.
*/
public val dataSourceName: Output?
get() = javaResource.dataSourceName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The GraphQL field on a type that invokes the resolver.
*/
public val fieldName: Output
get() = javaResource.fieldName().applyValue({ args0 -> args0 })
/**
* The resolver type.
* + *UNIT*: A UNIT resolver type. A UNIT resolver is the default resolver type. You can use a UNIT resolver to run a GraphQL query against a single data source.
* + *PIPELINE*: A PIPELINE resolver type. You can use a PIPELINE resolver to invoke a series of ``Function`` objects in a serial manner. You can use a pipeline resolver to run a GraphQL query against multiple data sources.
*/
public val kind: Output?
get() = javaResource.kind().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The maximum number of resolver request inputs that will be sent to a single LAMlong function in a ``BatchInvoke`` operation.
*/
public val maxBatchSize: Output?
get() = javaResource.maxBatchSize().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Enables or disables enhanced resolver metrics for specified resolvers. Note that ``MetricsConfig`` won't be used unless the ``resolverLevelMetricsBehavior`` value is set to ``PER_RESOLVER_METRICS``. If the ``resolverLevelMetricsBehavior`` is set to ``FULL_REQUEST_RESOLVER_METRICS`` instead, ``MetricsConfig`` will be ignored. However, you can still set its value.
*/
public val metricsConfig: Output?
get() = javaResource.metricsConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> resolverMetricsConfigToKotlin(args0) })
}).orElse(null)
})
/**
* Functions linked with the pipeline resolver.
*/
public val pipelineConfig: Output?
get() = javaResource.pipelineConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> resolverPipelineConfigToKotlin(args0) })
}).orElse(null)
})
/**
* The request mapping template.
* Request mapping templates are optional when using a Lambda data source. For all other data sources, a request mapping template is required.
*/
public val requestMappingTemplate: Output?
get() = javaResource.requestMappingTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The location of a request mapping template in an S3 bucket. Use this if you want to provision with a template file in S3 rather than embedding it in your CFNshort template.
*/
public val requestMappingTemplateS3Location: Output?
get() = javaResource.requestMappingTemplateS3Location().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* ARN of the resolver, such as `arn:aws:appsync:us-east-1:123456789012:apis/graphqlapiid/types/typename/resolvers/resolvername` .
*/
public val resolverArn: Output
get() = javaResource.resolverArn().applyValue({ args0 -> args0 })
/**
* The response mapping template.
*/
public val responseMappingTemplate: Output?
get() = javaResource.responseMappingTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The location of a response mapping template in an S3 bucket. Use this if you want to provision with a template file in S3 rather than embedding it in your CFNshort template.
*/
public val responseMappingTemplateS3Location: Output?
get() = javaResource.responseMappingTemplateS3Location().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* Describes a runtime used by an APSYlong resolver or APSYlong function. Specifies the name and version of the runtime to use. Note that if a runtime is specified, code must also be specified.
*/
public val runtime: Output?
get() = javaResource.runtime().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
resolverAppSyncRuntimeToKotlin(args0)
})
}).orElse(null)
})
/**
* The ``SyncConfig`` for a resolver attached to a versioned data source.
*/
public val syncConfig: Output?
get() = javaResource.syncConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
resolverSyncConfigToKotlin(args0)
})
}).orElse(null)
})
/**
* The GraphQL type that invokes this resolver.
*/
public val typeName: Output
get() = javaResource.typeName().applyValue({ args0 -> args0 })
}
public object ResolverMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.appsync.Resolver::class == javaResource::class
override fun map(javaResource: Resource): Resolver = Resolver(
javaResource as
com.pulumi.awsnative.appsync.Resolver,
)
}
/**
* @see [Resolver].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Resolver].
*/
public suspend fun resolver(name: String, block: suspend ResolverResourceBuilder.() -> Unit): Resolver {
val builder = ResolverResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Resolver].
* @param name The _unique_ name of the resulting resource.
*/
public fun resolver(name: String): Resolver {
val builder = ResolverResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy