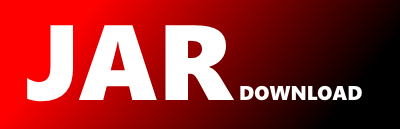
com.pulumi.awsnative.athena.kotlin.AthenaFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.athena.kotlin
import com.pulumi.awsnative.athena.AthenaFunctions.getCapacityReservationPlain
import com.pulumi.awsnative.athena.AthenaFunctions.getDataCatalogPlain
import com.pulumi.awsnative.athena.AthenaFunctions.getNamedQueryPlain
import com.pulumi.awsnative.athena.AthenaFunctions.getPreparedStatementPlain
import com.pulumi.awsnative.athena.AthenaFunctions.getWorkGroupPlain
import com.pulumi.awsnative.athena.kotlin.inputs.GetCapacityReservationPlainArgs
import com.pulumi.awsnative.athena.kotlin.inputs.GetCapacityReservationPlainArgsBuilder
import com.pulumi.awsnative.athena.kotlin.inputs.GetDataCatalogPlainArgs
import com.pulumi.awsnative.athena.kotlin.inputs.GetDataCatalogPlainArgsBuilder
import com.pulumi.awsnative.athena.kotlin.inputs.GetNamedQueryPlainArgs
import com.pulumi.awsnative.athena.kotlin.inputs.GetNamedQueryPlainArgsBuilder
import com.pulumi.awsnative.athena.kotlin.inputs.GetPreparedStatementPlainArgs
import com.pulumi.awsnative.athena.kotlin.inputs.GetPreparedStatementPlainArgsBuilder
import com.pulumi.awsnative.athena.kotlin.inputs.GetWorkGroupPlainArgs
import com.pulumi.awsnative.athena.kotlin.inputs.GetWorkGroupPlainArgsBuilder
import com.pulumi.awsnative.athena.kotlin.outputs.GetCapacityReservationResult
import com.pulumi.awsnative.athena.kotlin.outputs.GetDataCatalogResult
import com.pulumi.awsnative.athena.kotlin.outputs.GetNamedQueryResult
import com.pulumi.awsnative.athena.kotlin.outputs.GetPreparedStatementResult
import com.pulumi.awsnative.athena.kotlin.outputs.GetWorkGroupResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.athena.kotlin.outputs.GetCapacityReservationResult.Companion.toKotlin as getCapacityReservationResultToKotlin
import com.pulumi.awsnative.athena.kotlin.outputs.GetDataCatalogResult.Companion.toKotlin as getDataCatalogResultToKotlin
import com.pulumi.awsnative.athena.kotlin.outputs.GetNamedQueryResult.Companion.toKotlin as getNamedQueryResultToKotlin
import com.pulumi.awsnative.athena.kotlin.outputs.GetPreparedStatementResult.Companion.toKotlin as getPreparedStatementResultToKotlin
import com.pulumi.awsnative.athena.kotlin.outputs.GetWorkGroupResult.Companion.toKotlin as getWorkGroupResultToKotlin
public object AthenaFunctions {
/**
* Resource schema for AWS::Athena::CapacityReservation
* @param argument null
* @return null
*/
public suspend fun getCapacityReservation(argument: GetCapacityReservationPlainArgs): GetCapacityReservationResult =
getCapacityReservationResultToKotlin(getCapacityReservationPlain(argument.toJava()).await())
/**
* @see [getCapacityReservation].
* @param arn The ARN of the capacity reservation.
* @return null
*/
public suspend fun getCapacityReservation(arn: String): GetCapacityReservationResult {
val argument = GetCapacityReservationPlainArgs(
arn = arn,
)
return getCapacityReservationResultToKotlin(getCapacityReservationPlain(argument.toJava()).await())
}
/**
* @see [getCapacityReservation].
* @param argument Builder for [com.pulumi.awsnative.athena.kotlin.inputs.GetCapacityReservationPlainArgs].
* @return null
*/
public suspend fun getCapacityReservation(argument: suspend GetCapacityReservationPlainArgsBuilder.() -> Unit): GetCapacityReservationResult {
val builder = GetCapacityReservationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCapacityReservationResultToKotlin(getCapacityReservationPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::Athena::DataCatalog
* @param argument null
* @return null
*/
public suspend fun getDataCatalog(argument: GetDataCatalogPlainArgs): GetDataCatalogResult =
getDataCatalogResultToKotlin(getDataCatalogPlain(argument.toJava()).await())
/**
* @see [getDataCatalog].
* @param name The name of the data catalog to create. The catalog name must be unique for the AWS account and can use a maximum of 128 alphanumeric, underscore, at sign, or hyphen characters.
* @return null
*/
public suspend fun getDataCatalog(name: String): GetDataCatalogResult {
val argument = GetDataCatalogPlainArgs(
name = name,
)
return getDataCatalogResultToKotlin(getDataCatalogPlain(argument.toJava()).await())
}
/**
* @see [getDataCatalog].
* @param argument Builder for [com.pulumi.awsnative.athena.kotlin.inputs.GetDataCatalogPlainArgs].
* @return null
*/
public suspend fun getDataCatalog(argument: suspend GetDataCatalogPlainArgsBuilder.() -> Unit): GetDataCatalogResult {
val builder = GetDataCatalogPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDataCatalogResultToKotlin(getDataCatalogPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::Athena::NamedQuery
* @param argument null
* @return null
*/
public suspend fun getNamedQuery(argument: GetNamedQueryPlainArgs): GetNamedQueryResult =
getNamedQueryResultToKotlin(getNamedQueryPlain(argument.toJava()).await())
/**
* @see [getNamedQuery].
* @param namedQueryId The unique ID of the query.
* @return null
*/
public suspend fun getNamedQuery(namedQueryId: String): GetNamedQueryResult {
val argument = GetNamedQueryPlainArgs(
namedQueryId = namedQueryId,
)
return getNamedQueryResultToKotlin(getNamedQueryPlain(argument.toJava()).await())
}
/**
* @see [getNamedQuery].
* @param argument Builder for [com.pulumi.awsnative.athena.kotlin.inputs.GetNamedQueryPlainArgs].
* @return null
*/
public suspend fun getNamedQuery(argument: suspend GetNamedQueryPlainArgsBuilder.() -> Unit): GetNamedQueryResult {
val builder = GetNamedQueryPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getNamedQueryResultToKotlin(getNamedQueryPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::Athena::PreparedStatement
* @param argument null
* @return null
*/
public suspend fun getPreparedStatement(argument: GetPreparedStatementPlainArgs): GetPreparedStatementResult =
getPreparedStatementResultToKotlin(getPreparedStatementPlain(argument.toJava()).await())
/**
* @see [getPreparedStatement].
* @param statementName The name of the prepared statement.
* @param workGroup The name of the workgroup to which the prepared statement belongs.
* @return null
*/
public suspend fun getPreparedStatement(statementName: String, workGroup: String): GetPreparedStatementResult {
val argument = GetPreparedStatementPlainArgs(
statementName = statementName,
workGroup = workGroup,
)
return getPreparedStatementResultToKotlin(getPreparedStatementPlain(argument.toJava()).await())
}
/**
* @see [getPreparedStatement].
* @param argument Builder for [com.pulumi.awsnative.athena.kotlin.inputs.GetPreparedStatementPlainArgs].
* @return null
*/
public suspend fun getPreparedStatement(argument: suspend GetPreparedStatementPlainArgsBuilder.() -> Unit): GetPreparedStatementResult {
val builder = GetPreparedStatementPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPreparedStatementResultToKotlin(getPreparedStatementPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::Athena::WorkGroup
* @param argument null
* @return null
*/
public suspend fun getWorkGroup(argument: GetWorkGroupPlainArgs): GetWorkGroupResult =
getWorkGroupResultToKotlin(getWorkGroupPlain(argument.toJava()).await())
/**
* @see [getWorkGroup].
* @param name The workGroup name.
* @return null
*/
public suspend fun getWorkGroup(name: String): GetWorkGroupResult {
val argument = GetWorkGroupPlainArgs(
name = name,
)
return getWorkGroupResultToKotlin(getWorkGroupPlain(argument.toJava()).await())
}
/**
* @see [getWorkGroup].
* @param argument Builder for [com.pulumi.awsnative.athena.kotlin.inputs.GetWorkGroupPlainArgs].
* @return null
*/
public suspend fun getWorkGroup(argument: suspend GetWorkGroupPlainArgsBuilder.() -> Unit): GetWorkGroupResult {
val builder = GetWorkGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWorkGroupResultToKotlin(getWorkGroupPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy