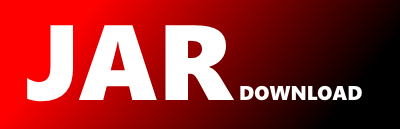
com.pulumi.awsnative.athena.kotlin.inputs.WorkGroupConfigurationUpdatesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.athena.kotlin.inputs
import com.pulumi.awsnative.athena.inputs.WorkGroupConfigurationUpdatesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The configuration information that will be updated for this workgroup, which includes the location in Amazon S3 where query results are stored, the encryption option, if any, used for query results, whether the Amazon CloudWatch Metrics are enabled for the workgroup, whether the workgroup settings override the client-side settings, and the data usage limit for the amount of bytes scanned per query, if it is specified.
* @property additionalConfiguration
* @property bytesScannedCutoffPerQuery
* @property customerContentEncryptionConfiguration
* @property enforceWorkGroupConfiguration
* @property engineVersion
* @property executionRole
* @property publishCloudWatchMetricsEnabled
* @property removeBytesScannedCutoffPerQuery
* @property removeCustomerContentEncryptionConfiguration
* @property requesterPaysEnabled
* @property resultConfigurationUpdates
*/
public data class WorkGroupConfigurationUpdatesArgs(
public val additionalConfiguration: Output? = null,
public val bytesScannedCutoffPerQuery: Output? = null,
public val customerContentEncryptionConfiguration: Output? = null,
public val enforceWorkGroupConfiguration: Output? = null,
public val engineVersion: Output? = null,
public val executionRole: Output? = null,
public val publishCloudWatchMetricsEnabled: Output? = null,
public val removeBytesScannedCutoffPerQuery: Output? = null,
public val removeCustomerContentEncryptionConfiguration: Output? = null,
public val requesterPaysEnabled: Output? = null,
public val resultConfigurationUpdates: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.athena.inputs.WorkGroupConfigurationUpdatesArgs =
com.pulumi.awsnative.athena.inputs.WorkGroupConfigurationUpdatesArgs.builder()
.additionalConfiguration(additionalConfiguration?.applyValue({ args0 -> args0 }))
.bytesScannedCutoffPerQuery(bytesScannedCutoffPerQuery?.applyValue({ args0 -> args0 }))
.customerContentEncryptionConfiguration(
customerContentEncryptionConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.enforceWorkGroupConfiguration(enforceWorkGroupConfiguration?.applyValue({ args0 -> args0 }))
.engineVersion(engineVersion?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.executionRole(executionRole?.applyValue({ args0 -> args0 }))
.publishCloudWatchMetricsEnabled(publishCloudWatchMetricsEnabled?.applyValue({ args0 -> args0 }))
.removeBytesScannedCutoffPerQuery(removeBytesScannedCutoffPerQuery?.applyValue({ args0 -> args0 }))
.removeCustomerContentEncryptionConfiguration(
removeCustomerContentEncryptionConfiguration?.applyValue({ args0 ->
args0
}),
)
.requesterPaysEnabled(requesterPaysEnabled?.applyValue({ args0 -> args0 }))
.resultConfigurationUpdates(
resultConfigurationUpdates?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [WorkGroupConfigurationUpdatesArgs].
*/
@PulumiTagMarker
public class WorkGroupConfigurationUpdatesArgsBuilder internal constructor() {
private var additionalConfiguration: Output? = null
private var bytesScannedCutoffPerQuery: Output? = null
private var customerContentEncryptionConfiguration:
Output? = null
private var enforceWorkGroupConfiguration: Output? = null
private var engineVersion: Output? = null
private var executionRole: Output? = null
private var publishCloudWatchMetricsEnabled: Output? = null
private var removeBytesScannedCutoffPerQuery: Output? = null
private var removeCustomerContentEncryptionConfiguration: Output? = null
private var requesterPaysEnabled: Output? = null
private var resultConfigurationUpdates: Output? = null
/**
* @param value
*/
@JvmName("wmulnvspeyehqdll")
public suspend fun additionalConfiguration(`value`: Output) {
this.additionalConfiguration = value
}
/**
* @param value
*/
@JvmName("upqcmunvjsasekjm")
public suspend fun bytesScannedCutoffPerQuery(`value`: Output) {
this.bytesScannedCutoffPerQuery = value
}
/**
* @param value
*/
@JvmName("jvwhyogjojmakuuw")
public suspend fun customerContentEncryptionConfiguration(`value`: Output) {
this.customerContentEncryptionConfiguration = value
}
/**
* @param value
*/
@JvmName("vowvcwyugmdywtsw")
public suspend fun enforceWorkGroupConfiguration(`value`: Output) {
this.enforceWorkGroupConfiguration = value
}
/**
* @param value
*/
@JvmName("lrkoydlvexqnbsfx")
public suspend fun engineVersion(`value`: Output) {
this.engineVersion = value
}
/**
* @param value
*/
@JvmName("offnyhagiuqdpbau")
public suspend fun executionRole(`value`: Output) {
this.executionRole = value
}
/**
* @param value
*/
@JvmName("xibiekdcwvrabxjd")
public suspend fun publishCloudWatchMetricsEnabled(`value`: Output) {
this.publishCloudWatchMetricsEnabled = value
}
/**
* @param value
*/
@JvmName("ofsfnvxeuyebibgt")
public suspend fun removeBytesScannedCutoffPerQuery(`value`: Output) {
this.removeBytesScannedCutoffPerQuery = value
}
/**
* @param value
*/
@JvmName("katggdrntgilmekj")
public suspend fun removeCustomerContentEncryptionConfiguration(`value`: Output) {
this.removeCustomerContentEncryptionConfiguration = value
}
/**
* @param value
*/
@JvmName("ukdondnvjnxnmieu")
public suspend fun requesterPaysEnabled(`value`: Output) {
this.requesterPaysEnabled = value
}
/**
* @param value
*/
@JvmName("cyvoaalckaerbhhy")
public suspend fun resultConfigurationUpdates(`value`: Output) {
this.resultConfigurationUpdates = value
}
/**
* @param value
*/
@JvmName("sjhjwkdukyfotdlw")
public suspend fun additionalConfiguration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.additionalConfiguration = mapped
}
/**
* @param value
*/
@JvmName("dhrwpkldjkrhelqy")
public suspend fun bytesScannedCutoffPerQuery(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bytesScannedCutoffPerQuery = mapped
}
/**
* @param value
*/
@JvmName("nuwhrbpiixwkphgn")
public suspend fun customerContentEncryptionConfiguration(`value`: WorkGroupCustomerContentEncryptionConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customerContentEncryptionConfiguration = mapped
}
/**
* @param argument
*/
@JvmName("pyxjcfatlgwruybh")
public suspend fun customerContentEncryptionConfiguration(argument: suspend WorkGroupCustomerContentEncryptionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = WorkGroupCustomerContentEncryptionConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customerContentEncryptionConfiguration = mapped
}
/**
* @param value
*/
@JvmName("xrlkkxffljlrfibv")
public suspend fun enforceWorkGroupConfiguration(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enforceWorkGroupConfiguration = mapped
}
/**
* @param value
*/
@JvmName("hrcqilkwolavkadm")
public suspend fun engineVersion(`value`: WorkGroupEngineVersionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.engineVersion = mapped
}
/**
* @param argument
*/
@JvmName("pevrrgrkdqjwntel")
public suspend fun engineVersion(argument: suspend WorkGroupEngineVersionArgsBuilder.() -> Unit) {
val toBeMapped = WorkGroupEngineVersionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.engineVersion = mapped
}
/**
* @param value
*/
@JvmName("rjpryraqjsimugvd")
public suspend fun executionRole(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.executionRole = mapped
}
/**
* @param value
*/
@JvmName("gynumihtjnxdjxkc")
public suspend fun publishCloudWatchMetricsEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publishCloudWatchMetricsEnabled = mapped
}
/**
* @param value
*/
@JvmName("ndeqepksijawibjb")
public suspend fun removeBytesScannedCutoffPerQuery(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.removeBytesScannedCutoffPerQuery = mapped
}
/**
* @param value
*/
@JvmName("pllaqitwcbcrcjsa")
public suspend fun removeCustomerContentEncryptionConfiguration(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.removeCustomerContentEncryptionConfiguration = mapped
}
/**
* @param value
*/
@JvmName("qhygicbhgjefxvke")
public suspend fun requesterPaysEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requesterPaysEnabled = mapped
}
/**
* @param value
*/
@JvmName("xruhwfvgglheomyv")
public suspend fun resultConfigurationUpdates(`value`: WorkGroupResultConfigurationUpdatesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resultConfigurationUpdates = mapped
}
/**
* @param argument
*/
@JvmName("bifsixcqqlvctuvm")
public suspend fun resultConfigurationUpdates(argument: suspend WorkGroupResultConfigurationUpdatesArgsBuilder.() -> Unit) {
val toBeMapped = WorkGroupResultConfigurationUpdatesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.resultConfigurationUpdates = mapped
}
internal fun build(): WorkGroupConfigurationUpdatesArgs = WorkGroupConfigurationUpdatesArgs(
additionalConfiguration = additionalConfiguration,
bytesScannedCutoffPerQuery = bytesScannedCutoffPerQuery,
customerContentEncryptionConfiguration = customerContentEncryptionConfiguration,
enforceWorkGroupConfiguration = enforceWorkGroupConfiguration,
engineVersion = engineVersion,
executionRole = executionRole,
publishCloudWatchMetricsEnabled = publishCloudWatchMetricsEnabled,
removeBytesScannedCutoffPerQuery = removeBytesScannedCutoffPerQuery,
removeCustomerContentEncryptionConfiguration = removeCustomerContentEncryptionConfiguration,
requesterPaysEnabled = requesterPaysEnabled,
resultConfigurationUpdates = resultConfigurationUpdates,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy