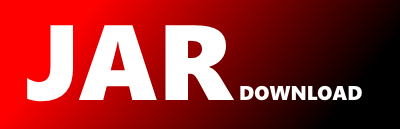
com.pulumi.awsnative.auditmanager.kotlin.Assessment.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.auditmanager.kotlin
import com.pulumi.awsnative.auditmanager.kotlin.enums.AssessmentStatus
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentAwsAccount
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentDelegation
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentReportsDestination
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentRole
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentScope
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.auditmanager.kotlin.enums.AssessmentStatus.Companion.toKotlin as assessmentStatusToKotlin
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentAwsAccount.Companion.toKotlin as assessmentAwsAccountToKotlin
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentDelegation.Companion.toKotlin as assessmentDelegationToKotlin
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentReportsDestination.Companion.toKotlin as assessmentReportsDestinationToKotlin
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentRole.Companion.toKotlin as assessmentRoleToKotlin
import com.pulumi.awsnative.auditmanager.kotlin.outputs.AssessmentScope.Companion.toKotlin as assessmentScopeToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [Assessment].
*/
@PulumiTagMarker
public class AssessmentResourceBuilder internal constructor() {
public var name: String? = null
public var args: AssessmentArgs = AssessmentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AssessmentArgsBuilder.() -> Unit) {
val builder = AssessmentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Assessment {
val builtJavaResource = com.pulumi.awsnative.auditmanager.Assessment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Assessment(builtJavaResource)
}
}
/**
* An entity that defines the scope of audit evidence collected by AWS Audit Manager.
*/
public class Assessment internal constructor(
override val javaResource: com.pulumi.awsnative.auditmanager.Assessment,
) : KotlinCustomResource(javaResource, AssessmentMapper) {
/**
* The Amazon Resource Name (ARN) of the assessment.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The unique identifier for the assessment.
*/
public val assessmentId: Output
get() = javaResource.assessmentId().applyValue({ args0 -> args0 })
/**
* The destination that evidence reports are stored in for the assessment.
*/
public val assessmentReportsDestination: Output?
get() = javaResource.assessmentReportsDestination().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> assessmentReportsDestinationToKotlin(args0) })
}).orElse(null)
})
/**
* The AWS account that's associated with the assessment.
*/
public val awsAccount: Output?
get() = javaResource.awsAccount().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
assessmentAwsAccountToKotlin(args0)
})
}).orElse(null)
})
/**
* Specifies when the assessment was created.
*/
public val creationTime: Output
get() = javaResource.creationTime().applyValue({ args0 -> args0 })
/**
* The list of delegations.
*/
public val delegations: Output>?
get() = javaResource.delegations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> assessmentDelegationToKotlin(args0) })
})
}).orElse(null)
})
/**
* The description of the assessment.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The unique identifier for the framework.
*/
public val frameworkId: Output?
get() = javaResource.frameworkId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the assessment.
*/
public val name: Output?
get() = javaResource.name().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The list of roles for the specified assessment.
*/
public val roles: Output>?
get() = javaResource.roles().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> assessmentRoleToKotlin(args0) })
})
}).orElse(null)
})
/**
* The wrapper of AWS accounts and services that are in scope for the assessment.
*/
public val scope: Output?
get() = javaResource.scope().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
assessmentScopeToKotlin(args0)
})
}).orElse(null)
})
/**
* The overall status of the assessment.
* When you create a new assessment, the initial `Status` value is always `ACTIVE` . When you create an assessment, even if you specify the value as `INACTIVE` , the value overrides to `ACTIVE` .
* After you create an assessment, you can change the value of the `Status` property at any time. For example, when you want to stop collecting evidence for your assessment, you can change the assessment status to `INACTIVE` .
*/
public val status: Output?
get() = javaResource.status().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
assessmentStatusToKotlin(args0)
})
}).orElse(null)
})
/**
* The tags associated with the assessment.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
}
public object AssessmentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.auditmanager.Assessment::class == javaResource::class
override fun map(javaResource: Resource): Assessment = Assessment(
javaResource as
com.pulumi.awsnative.auditmanager.Assessment,
)
}
/**
* @see [Assessment].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Assessment].
*/
public suspend fun assessment(name: String, block: suspend AssessmentResourceBuilder.() -> Unit): Assessment {
val builder = AssessmentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Assessment].
* @param name The _unique_ name of the resulting resource.
*/
public fun assessment(name: String): Assessment {
val builder = AssessmentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy