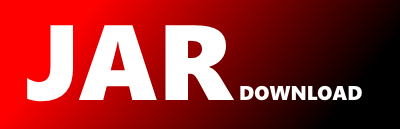
com.pulumi.awsnative.autoscaling.kotlin.ScheduledAction.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.autoscaling.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [ScheduledAction].
*/
@PulumiTagMarker
public class ScheduledActionResourceBuilder internal constructor() {
public var name: String? = null
public var args: ScheduledActionArgs = ScheduledActionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ScheduledActionArgsBuilder.() -> Unit) {
val builder = ScheduledActionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ScheduledAction {
val builtJavaResource =
com.pulumi.awsnative.autoscaling.ScheduledAction(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ScheduledAction(builtJavaResource)
}
}
/**
* The AWS::AutoScaling::ScheduledAction resource specifies an Amazon EC2 Auto Scaling scheduled action so that the Auto Scaling group can change the number of instances available for your application in response to predictable load changes.
*/
public class ScheduledAction internal constructor(
override val javaResource: com.pulumi.awsnative.autoscaling.ScheduledAction,
) : KotlinCustomResource(javaResource, ScheduledActionMapper) {
/**
* The name of the Auto Scaling group.
*/
public val autoScalingGroupName: Output
get() = javaResource.autoScalingGroupName().applyValue({ args0 -> args0 })
/**
* The desired capacity is the initial capacity of the Auto Scaling group after the scheduled action runs and the capacity it attempts to maintain.
*/
public val desiredCapacity: Output?
get() = javaResource.desiredCapacity().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The latest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
*/
public val endTime: Output?
get() = javaResource.endTime().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The minimum size of the Auto Scaling group.
*/
public val maxSize: Output?
get() = javaResource.maxSize().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The minimum size of the Auto Scaling group.
*/
public val minSize: Output?
get() = javaResource.minSize().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The recurring schedule for the action, in Unix cron syntax format. When StartTime and EndTime are specified with Recurrence , they form the boundaries of when the recurring action starts and stops.
*/
public val recurrence: Output?
get() = javaResource.recurrence().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Auto-generated unique identifier
*/
public val scheduledActionName: Output
get() = javaResource.scheduledActionName().applyValue({ args0 -> args0 })
/**
* The earliest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
*/
public val startTime: Output?
get() = javaResource.startTime().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The time zone for the cron expression.
*/
public val timeZone: Output?
get() = javaResource.timeZone().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object ScheduledActionMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.autoscaling.ScheduledAction::class == javaResource::class
override fun map(javaResource: Resource): ScheduledAction = ScheduledAction(
javaResource as
com.pulumi.awsnative.autoscaling.ScheduledAction,
)
}
/**
* @see [ScheduledAction].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ScheduledAction].
*/
public suspend fun scheduledAction(
name: String,
block: suspend ScheduledActionResourceBuilder.() -> Unit,
): ScheduledAction {
val builder = ScheduledActionResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ScheduledAction].
* @param name The _unique_ name of the resulting resource.
*/
public fun scheduledAction(name: String): ScheduledAction {
val builder = ScheduledActionResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy