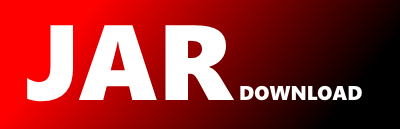
com.pulumi.awsnative.autoscaling.kotlin.ScheduledActionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.autoscaling.kotlin
import com.pulumi.awsnative.autoscaling.ScheduledActionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The AWS::AutoScaling::ScheduledAction resource specifies an Amazon EC2 Auto Scaling scheduled action so that the Auto Scaling group can change the number of instances available for your application in response to predictable load changes.
* @property autoScalingGroupName The name of the Auto Scaling group.
* @property desiredCapacity The desired capacity is the initial capacity of the Auto Scaling group after the scheduled action runs and the capacity it attempts to maintain.
* @property endTime The latest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
* @property maxSize The minimum size of the Auto Scaling group.
* @property minSize The minimum size of the Auto Scaling group.
* @property recurrence The recurring schedule for the action, in Unix cron syntax format. When StartTime and EndTime are specified with Recurrence , they form the boundaries of when the recurring action starts and stops.
* @property startTime The earliest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
* @property timeZone The time zone for the cron expression.
*/
public data class ScheduledActionArgs(
public val autoScalingGroupName: Output? = null,
public val desiredCapacity: Output? = null,
public val endTime: Output? = null,
public val maxSize: Output? = null,
public val minSize: Output? = null,
public val recurrence: Output? = null,
public val startTime: Output? = null,
public val timeZone: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.autoscaling.ScheduledActionArgs =
com.pulumi.awsnative.autoscaling.ScheduledActionArgs.builder()
.autoScalingGroupName(autoScalingGroupName?.applyValue({ args0 -> args0 }))
.desiredCapacity(desiredCapacity?.applyValue({ args0 -> args0 }))
.endTime(endTime?.applyValue({ args0 -> args0 }))
.maxSize(maxSize?.applyValue({ args0 -> args0 }))
.minSize(minSize?.applyValue({ args0 -> args0 }))
.recurrence(recurrence?.applyValue({ args0 -> args0 }))
.startTime(startTime?.applyValue({ args0 -> args0 }))
.timeZone(timeZone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScheduledActionArgs].
*/
@PulumiTagMarker
public class ScheduledActionArgsBuilder internal constructor() {
private var autoScalingGroupName: Output? = null
private var desiredCapacity: Output? = null
private var endTime: Output? = null
private var maxSize: Output? = null
private var minSize: Output? = null
private var recurrence: Output? = null
private var startTime: Output? = null
private var timeZone: Output? = null
/**
* @param value The name of the Auto Scaling group.
*/
@JvmName("yyyrliveowysafcq")
public suspend fun autoScalingGroupName(`value`: Output) {
this.autoScalingGroupName = value
}
/**
* @param value The desired capacity is the initial capacity of the Auto Scaling group after the scheduled action runs and the capacity it attempts to maintain.
*/
@JvmName("ttkaqaragxqljibg")
public suspend fun desiredCapacity(`value`: Output) {
this.desiredCapacity = value
}
/**
* @param value The latest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
*/
@JvmName("dsjxndhfyvhlruca")
public suspend fun endTime(`value`: Output) {
this.endTime = value
}
/**
* @param value The minimum size of the Auto Scaling group.
*/
@JvmName("uurscxqtqolbolxd")
public suspend fun maxSize(`value`: Output) {
this.maxSize = value
}
/**
* @param value The minimum size of the Auto Scaling group.
*/
@JvmName("vqejlkmmnkblusbr")
public suspend fun minSize(`value`: Output) {
this.minSize = value
}
/**
* @param value The recurring schedule for the action, in Unix cron syntax format. When StartTime and EndTime are specified with Recurrence , they form the boundaries of when the recurring action starts and stops.
*/
@JvmName("wjwggabxksopsiuo")
public suspend fun recurrence(`value`: Output) {
this.recurrence = value
}
/**
* @param value The earliest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
*/
@JvmName("hslgejkaieshtvcx")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value The time zone for the cron expression.
*/
@JvmName("vppxcppphrjriraf")
public suspend fun timeZone(`value`: Output) {
this.timeZone = value
}
/**
* @param value The name of the Auto Scaling group.
*/
@JvmName("wbdhnthuelmwfdfx")
public suspend fun autoScalingGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoScalingGroupName = mapped
}
/**
* @param value The desired capacity is the initial capacity of the Auto Scaling group after the scheduled action runs and the capacity it attempts to maintain.
*/
@JvmName("uxcbujioecvyurqe")
public suspend fun desiredCapacity(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.desiredCapacity = mapped
}
/**
* @param value The latest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
*/
@JvmName("xrdbckcavykmrrjp")
public suspend fun endTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTime = mapped
}
/**
* @param value The minimum size of the Auto Scaling group.
*/
@JvmName("phchmytjjjjmtrgm")
public suspend fun maxSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxSize = mapped
}
/**
* @param value The minimum size of the Auto Scaling group.
*/
@JvmName("khssapusdiukcpli")
public suspend fun minSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minSize = mapped
}
/**
* @param value The recurring schedule for the action, in Unix cron syntax format. When StartTime and EndTime are specified with Recurrence , they form the boundaries of when the recurring action starts and stops.
*/
@JvmName("swisfbkpjlbwxfga")
public suspend fun recurrence(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recurrence = mapped
}
/**
* @param value The earliest scheduled start time to return. If scheduled action names are provided, this parameter is ignored.
*/
@JvmName("qbvhitrbdxupcqem")
public suspend fun startTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTime = mapped
}
/**
* @param value The time zone for the cron expression.
*/
@JvmName("bxkbaxrjcsudfqwi")
public suspend fun timeZone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeZone = mapped
}
internal fun build(): ScheduledActionArgs = ScheduledActionArgs(
autoScalingGroupName = autoScalingGroupName,
desiredCapacity = desiredCapacity,
endTime = endTime,
maxSize = maxSize,
minSize = minSize,
recurrence = recurrence,
startTime = startTime,
timeZone = timeZone,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy