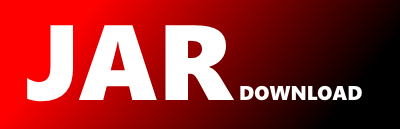
com.pulumi.awsnative.backup.kotlin.BackupVaultArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.backup.kotlin
import com.pulumi.awsnative.backup.BackupVaultArgs.builder
import com.pulumi.awsnative.backup.kotlin.inputs.BackupVaultLockConfigurationTypeArgs
import com.pulumi.awsnative.backup.kotlin.inputs.BackupVaultLockConfigurationTypeArgsBuilder
import com.pulumi.awsnative.backup.kotlin.inputs.BackupVaultNotificationObjectTypeArgs
import com.pulumi.awsnative.backup.kotlin.inputs.BackupVaultNotificationObjectTypeArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Backup::BackupVault
* @property accessPolicy A resource-based policy that is used to manage access permissions on the target backup vault.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::Backup::BackupVault` for more information about the expected schema for this property.
* @property backupVaultName The name of a logical container where backups are stored. Backup vaults are identified by names that are unique to the account used to create them and the AWS Region where they are created.
* @property backupVaultTags The tags to assign to the backup vault.
* @property encryptionKeyArn A server-side encryption key you can specify to encrypt your backups from services that support full AWS Backup management; for example, `arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab` . If you specify a key, you must specify its ARN, not its alias. If you do not specify a key, AWS Backup creates a KMS key for you by default.
* To learn which AWS Backup services support full AWS Backup management and how AWS Backup handles encryption for backups from services that do not yet support full AWS Backup , see [Encryption for backups in AWS Backup](https://docs.aws.amazon.com/aws-backup/latest/devguide/encryption.html)
* @property lockConfiguration Configuration for [AWS Backup Vault Lock](https://docs.aws.amazon.com/aws-backup/latest/devguide/vault-lock.html) .
* @property notifications The SNS event notifications for the specified backup vault.
*/
public data class BackupVaultArgs(
public val accessPolicy: Output? = null,
public val backupVaultName: Output? = null,
public val backupVaultTags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy