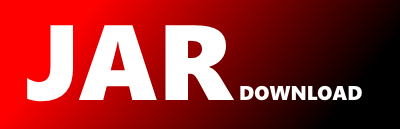
com.pulumi.awsnative.batch.kotlin.JobQueue.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.batch.kotlin
import com.pulumi.awsnative.batch.kotlin.enums.JobQueueState
import com.pulumi.awsnative.batch.kotlin.outputs.JobQueueComputeEnvironmentOrder
import com.pulumi.awsnative.batch.kotlin.outputs.JobQueueJobStateTimeLimitAction
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.awsnative.batch.kotlin.enums.JobQueueState.Companion.toKotlin as jobQueueStateToKotlin
import com.pulumi.awsnative.batch.kotlin.outputs.JobQueueComputeEnvironmentOrder.Companion.toKotlin as jobQueueComputeEnvironmentOrderToKotlin
import com.pulumi.awsnative.batch.kotlin.outputs.JobQueueJobStateTimeLimitAction.Companion.toKotlin as jobQueueJobStateTimeLimitActionToKotlin
/**
* Builder for [JobQueue].
*/
@PulumiTagMarker
public class JobQueueResourceBuilder internal constructor() {
public var name: String? = null
public var args: JobQueueArgs = JobQueueArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend JobQueueArgsBuilder.() -> Unit) {
val builder = JobQueueArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): JobQueue {
val builtJavaResource = com.pulumi.awsnative.batch.JobQueue(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return JobQueue(builtJavaResource)
}
}
/**
* Resource Type definition for AWS::Batch::JobQueue
*/
public class JobQueue internal constructor(
override val javaResource: com.pulumi.awsnative.batch.JobQueue,
) : KotlinCustomResource(javaResource, JobQueueMapper) {
/**
* The set of compute environments mapped to a job queue and their order relative to each other. The job scheduler uses this parameter to determine which compute environment runs a specific job. Compute environments must be in the `VALID` state before you can associate them with a job queue. You can associate up to three compute environments with a job queue. All of the compute environments must be either EC2 ( `EC2` or `SPOT` ) or Fargate ( `FARGATE` or `FARGATE_SPOT` ); EC2 and Fargate compute environments can't be mixed.
* > All compute environments that are associated with a job queue must share the same architecture. AWS Batch doesn't support mixing compute environment architecture types in a single job queue.
*/
public val computeEnvironmentOrder: Output>
get() = javaResource.computeEnvironmentOrder().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> jobQueueComputeEnvironmentOrderToKotlin(args0) })
})
})
/**
* Returns the job queue ARN, such as `batch: *us-east-1* : *111122223333* :job-queue/ *JobQueueName*` .
*/
public val jobQueueArn: Output
get() = javaResource.jobQueueArn().applyValue({ args0 -> args0 })
/**
* The name of the job queue. It can be up to 128 letters long. It can contain uppercase and lowercase letters, numbers, hyphens (-), and underscores (_).
*/
public val jobQueueName: Output?
get() = javaResource.jobQueueName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The set of actions that AWS Batch perform on jobs that remain at the head of the job queue in the specified state longer than specified times. AWS Batch will perform each action after `maxTimeSeconds` has passed.
*/
public val jobStateTimeLimitActions: Output>?
get() = javaResource.jobStateTimeLimitActions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
jobQueueJobStateTimeLimitActionToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The priority of the job queue. Job queues with a higher priority (or a higher integer value for the `priority` parameter) are evaluated first when associated with the same compute environment. Priority is determined in descending order. For example, a job queue with a priority value of `10` is given scheduling preference over a job queue with a priority value of `1` . All of the compute environments must be either EC2 ( `EC2` or `SPOT` ) or Fargate ( `FARGATE` or `FARGATE_SPOT` ); EC2 and Fargate compute environments can't be mixed.
*/
public val priority: Output
get() = javaResource.priority().applyValue({ args0 -> args0 })
/**
* The Amazon Resource Name (ARN) of the scheduling policy. The format is `aws: *Partition* :batch: *Region* : *Account* :scheduling-policy/ *Name*` . For example, `aws:aws:batch:us-west-2:123456789012:scheduling-policy/MySchedulingPolicy` .
*/
public val schedulingPolicyArn: Output?
get() = javaResource.schedulingPolicyArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The state of the job queue. If the job queue state is `ENABLED` , it is able to accept jobs. If the job queue state is `DISABLED` , new jobs can't be added to the queue, but jobs already in the queue can finish.
*/
public val state: Output?
get() = javaResource.state().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
jobQueueStateToKotlin(args0)
})
}).orElse(null)
})
/**
* A key-value pair to associate with a resource.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy