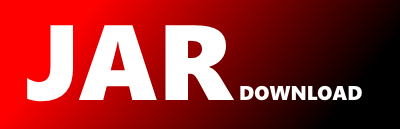
com.pulumi.awsnative.batch.kotlin.inputs.JobDefinitionSecretArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.batch.kotlin.inputs
import com.pulumi.awsnative.batch.inputs.JobDefinitionSecretArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property name The name of the secret.
* @property valueFrom The secret to expose to the container. The supported values are either the full Amazon Resource Name (ARN) of the AWS Secrets Manager secret or the full ARN of the parameter in the AWS Systems Manager Parameter Store.
* > If the AWS Systems Manager Parameter Store parameter exists in the same Region as the job you're launching, then you can use either the full Amazon Resource Name (ARN) or name of the parameter. If the parameter exists in a different Region, then the full ARN must be specified.
*/
public data class JobDefinitionSecretArgs(
public val name: Output,
public val valueFrom: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.batch.inputs.JobDefinitionSecretArgs =
com.pulumi.awsnative.batch.inputs.JobDefinitionSecretArgs.builder()
.name(name.applyValue({ args0 -> args0 }))
.valueFrom(valueFrom.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [JobDefinitionSecretArgs].
*/
@PulumiTagMarker
public class JobDefinitionSecretArgsBuilder internal constructor() {
private var name: Output? = null
private var valueFrom: Output? = null
/**
* @param value The name of the secret.
*/
@JvmName("jfnaulydmhwwdulb")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The secret to expose to the container. The supported values are either the full Amazon Resource Name (ARN) of the AWS Secrets Manager secret or the full ARN of the parameter in the AWS Systems Manager Parameter Store.
* > If the AWS Systems Manager Parameter Store parameter exists in the same Region as the job you're launching, then you can use either the full Amazon Resource Name (ARN) or name of the parameter. If the parameter exists in a different Region, then the full ARN must be specified.
*/
@JvmName("rurbybsvrjfujxur")
public suspend fun valueFrom(`value`: Output) {
this.valueFrom = value
}
/**
* @param value The name of the secret.
*/
@JvmName("gnrdvjigvmkcaidy")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The secret to expose to the container. The supported values are either the full Amazon Resource Name (ARN) of the AWS Secrets Manager secret or the full ARN of the parameter in the AWS Systems Manager Parameter Store.
* > If the AWS Systems Manager Parameter Store parameter exists in the same Region as the job you're launching, then you can use either the full Amazon Resource Name (ARN) or name of the parameter. If the parameter exists in a different Region, then the full ARN must be specified.
*/
@JvmName("lajtdhiuorsisqyh")
public suspend fun valueFrom(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.valueFrom = mapped
}
internal fun build(): JobDefinitionSecretArgs = JobDefinitionSecretArgs(
name = name ?: throw PulumiNullFieldException("name"),
valueFrom = valueFrom ?: throw PulumiNullFieldException("valueFrom"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy