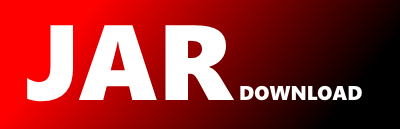
com.pulumi.awsnative.bedrock.kotlin.Agent.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.bedrock.kotlin
import com.pulumi.awsnative.bedrock.kotlin.enums.AgentStatus
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentActionGroup
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentGuardrailConfiguration
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentKnowledgeBase
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentPromptOverrideConfiguration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.awsnative.bedrock.kotlin.enums.AgentStatus.Companion.toKotlin as agentStatusToKotlin
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentActionGroup.Companion.toKotlin as agentActionGroupToKotlin
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentGuardrailConfiguration.Companion.toKotlin as agentGuardrailConfigurationToKotlin
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentKnowledgeBase.Companion.toKotlin as agentKnowledgeBaseToKotlin
import com.pulumi.awsnative.bedrock.kotlin.outputs.AgentPromptOverrideConfiguration.Companion.toKotlin as agentPromptOverrideConfigurationToKotlin
/**
* Builder for [Agent].
*/
@PulumiTagMarker
public class AgentResourceBuilder internal constructor() {
public var name: String? = null
public var args: AgentArgs = AgentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AgentArgsBuilder.() -> Unit) {
val builder = AgentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Agent {
val builtJavaResource = com.pulumi.awsnative.bedrock.Agent(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Agent(builtJavaResource)
}
}
/**
* Definition of AWS::Bedrock::Agent Resource Type
*/
public class Agent internal constructor(
override val javaResource: com.pulumi.awsnative.bedrock.Agent,
) : KotlinCustomResource(javaResource, AgentMapper) {
/**
* List of ActionGroups
*/
public val actionGroups: Output>?
get() = javaResource.actionGroups().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> agentActionGroupToKotlin(args0) })
})
}).orElse(null)
})
/**
* Arn representation of the Agent.
*/
public val agentArn: Output
get() = javaResource.agentArn().applyValue({ args0 -> args0 })
/**
* Identifier for a resource.
*/
public val agentId: Output
get() = javaResource.agentId().applyValue({ args0 -> args0 })
/**
* Name for a resource.
*/
public val agentName: Output
get() = javaResource.agentName().applyValue({ args0 -> args0 })
/**
* ARN of a IAM role.
*/
public val agentResourceRoleArn: Output?
get() = javaResource.agentResourceRoleArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The status of the agent and whether it is ready for use. The following statuses are possible:
* - CREATING – The agent is being created.
* - PREPARING – The agent is being prepared.
* - PREPARED – The agent is prepared and ready to be invoked.
* - NOT_PREPARED – The agent has been created but not yet prepared.
* - FAILED – The agent API operation failed.
* - UPDATING – The agent is being updated.
* - DELETING – The agent is being deleted.
*/
public val agentStatus: Output
get() = javaResource.agentStatus().applyValue({ args0 ->
args0.let({ args0 ->
agentStatusToKotlin(args0)
})
})
/**
* Draft Agent Version.
*/
public val agentVersion: Output
get() = javaResource.agentVersion().applyValue({ args0 -> args0 })
/**
* Specifies whether to automatically prepare after creating or updating the agent.
*/
public val autoPrepare: Output?
get() = javaResource.autoPrepare().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Time Stamp.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* A KMS key ARN
*/
public val customerEncryptionKeyArn: Output?
get() = javaResource.customerEncryptionKeyArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Description of the Resource.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Failure Reasons for Error.
*/
public val failureReasons: Output>
get() = javaResource.failureReasons().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* ARN or name of a Bedrock model.
*/
public val foundationModel: Output?
get() = javaResource.foundationModel().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Details about the guardrail associated with the agent.
*/
public val guardrailConfiguration: Output?
get() = javaResource.guardrailConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> agentGuardrailConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Max Session Time.
*/
public val idleSessionTtlInSeconds: Output?
get() = javaResource.idleSessionTtlInSeconds().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Instruction for the agent.
*/
public val instruction: Output?
get() = javaResource.instruction().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* List of Agent Knowledge Bases
*/
public val knowledgeBases: Output>?
get() = javaResource.knowledgeBases().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
agentKnowledgeBaseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Time Stamp.
*/
public val preparedAt: Output
get() = javaResource.preparedAt().applyValue({ args0 -> args0 })
/**
* Contains configurations to override prompt templates in different parts of an agent sequence. For more information, see [Advanced prompts](https://docs.aws.amazon.com/bedrock/latest/userguide/advanced-prompts.html) .
*/
public val promptOverrideConfiguration: Output?
get() = javaResource.promptOverrideConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> agentPromptOverrideConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* The recommended actions users can take to resolve an error in failureReasons.
*/
public val recommendedActions: Output>
get() = javaResource.recommendedActions().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Specifies whether to allow deleting agent while it is in use.
*/
public val skipResourceInUseCheckOnDelete: Output?
get() = javaResource.skipResourceInUseCheckOnDelete().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Metadata that you can assign to a resource as key-value pairs. For more information, see the following resources:
* - [Tag naming limits and requirements](https://docs.aws.amazon.com/tag-editor/latest/userguide/tagging.html#tag-conventions)
* - [Tagging best practices](https://docs.aws.amazon.com/tag-editor/latest/userguide/tagging.html#tag-best-practices)
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy