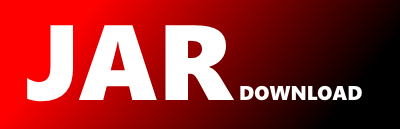
com.pulumi.awsnative.bedrock.kotlin.Flow.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.bedrock.kotlin
import com.pulumi.awsnative.bedrock.kotlin.enums.FlowStatus
import com.pulumi.awsnative.bedrock.kotlin.outputs.FlowDefinition
import com.pulumi.awsnative.bedrock.kotlin.outputs.FlowS3Location
import com.pulumi.awsnative.bedrock.kotlin.outputs.FlowValidation
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.awsnative.bedrock.kotlin.enums.FlowStatus.Companion.toKotlin as flowStatusToKotlin
import com.pulumi.awsnative.bedrock.kotlin.outputs.FlowDefinition.Companion.toKotlin as flowDefinitionToKotlin
import com.pulumi.awsnative.bedrock.kotlin.outputs.FlowS3Location.Companion.toKotlin as flowS3LocationToKotlin
import com.pulumi.awsnative.bedrock.kotlin.outputs.FlowValidation.Companion.toKotlin as flowValidationToKotlin
/**
* Builder for [Flow].
*/
@PulumiTagMarker
public class FlowResourceBuilder internal constructor() {
public var name: String? = null
public var args: FlowArgs = FlowArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FlowArgsBuilder.() -> Unit) {
val builder = FlowArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Flow {
val builtJavaResource = com.pulumi.awsnative.bedrock.Flow(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Flow(builtJavaResource)
}
}
/**
* Definition of AWS::Bedrock::Flow Resource Type
*/
public class Flow internal constructor(
override val javaResource: com.pulumi.awsnative.bedrock.Flow,
) : KotlinCustomResource(javaResource, FlowMapper) {
/**
* Arn representation of the Flow
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Identifier for a Flow
*/
public val awsId: Output
get() = javaResource.awsId().applyValue({ args0 -> args0 })
/**
* Time Stamp.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* A KMS key ARN
*/
public val customerEncryptionKeyArn: Output?
get() = javaResource.customerEncryptionKeyArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The definition of the nodes and connections between the nodes in the flow.
*/
public val definition: Output?
get() = javaResource.definition().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
flowDefinitionToKotlin(args0)
})
}).orElse(null)
})
/**
* The Amazon S3 location of the flow definition.
*/
public val definitionS3Location: Output?
get() = javaResource.definitionS3Location().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> flowS3LocationToKotlin(args0) })
}).orElse(null)
})
/**
* A JSON string containing a Definition with the same schema as the Definition property of this resource
*/
public val definitionString: Output?
get() = javaResource.definitionString().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A map that specifies the mappings for placeholder variables in the prompt flow definition. This enables the customer to inject values obtained at runtime. Variables can be template parameter names, resource logical IDs, resource attributes, or a variable in a key-value map. Only supported with the `DefinitionString` and `DefinitionS3Location` fields.
* Substitutions must follow the syntax: `${key_name}` or `${variable_1,variable_2,...}` .
*/
public val definitionSubstitutions: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy