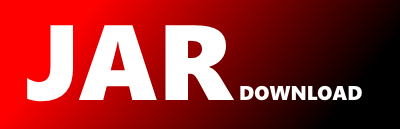
com.pulumi.awsnative.bedrock.kotlin.inputs.DataSourceSharePointSourceConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.bedrock.kotlin.inputs
import com.pulumi.awsnative.bedrock.inputs.DataSourceSharePointSourceConfigurationArgs.builder
import com.pulumi.awsnative.bedrock.kotlin.enums.DataSourceSharePointSourceConfigurationAuthType
import com.pulumi.awsnative.bedrock.kotlin.enums.DataSourceSharePointSourceConfigurationHostType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The endpoint information to connect to your SharePoint data source.
* @property authType The supported authentication type to authenticate and connect to your SharePoint site/sites.
* @property credentialsSecretArn The Amazon Resource Name of an AWS Secrets Manager secret that stores your authentication credentials for your SharePoint site/sites. For more information on the key-value pairs that must be included in your secret, depending on your authentication type, see SharePoint connection configuration.
* @property domain The domain of your SharePoint instance or site URL/URLs.
* @property hostType The supported host type, whether online/cloud or server/on-premises.
* @property siteUrls A list of one or more SharePoint site URLs.
* @property tenantId The identifier of your Microsoft 365 tenant.
*/
public data class DataSourceSharePointSourceConfigurationArgs(
public val authType: Output,
public val credentialsSecretArn: Output,
public val domain: Output,
public val hostType: Output,
public val siteUrls: Output>,
public val tenantId: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.bedrock.inputs.DataSourceSharePointSourceConfigurationArgs =
com.pulumi.awsnative.bedrock.inputs.DataSourceSharePointSourceConfigurationArgs.builder()
.authType(authType.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.credentialsSecretArn(credentialsSecretArn.applyValue({ args0 -> args0 }))
.domain(domain.applyValue({ args0 -> args0 }))
.hostType(hostType.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.siteUrls(siteUrls.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tenantId(tenantId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DataSourceSharePointSourceConfigurationArgs].
*/
@PulumiTagMarker
public class DataSourceSharePointSourceConfigurationArgsBuilder internal constructor() {
private var authType: Output? = null
private var credentialsSecretArn: Output? = null
private var domain: Output? = null
private var hostType: Output? = null
private var siteUrls: Output>? = null
private var tenantId: Output? = null
/**
* @param value The supported authentication type to authenticate and connect to your SharePoint site/sites.
*/
@JvmName("rwskptpkyoyygeng")
public suspend fun authType(`value`: Output) {
this.authType = value
}
/**
* @param value The Amazon Resource Name of an AWS Secrets Manager secret that stores your authentication credentials for your SharePoint site/sites. For more information on the key-value pairs that must be included in your secret, depending on your authentication type, see SharePoint connection configuration.
*/
@JvmName("waumritiukweslqe")
public suspend fun credentialsSecretArn(`value`: Output) {
this.credentialsSecretArn = value
}
/**
* @param value The domain of your SharePoint instance or site URL/URLs.
*/
@JvmName("qratepntpjcojyul")
public suspend fun domain(`value`: Output) {
this.domain = value
}
/**
* @param value The supported host type, whether online/cloud or server/on-premises.
*/
@JvmName("lciyydahhjjvuqym")
public suspend fun hostType(`value`: Output) {
this.hostType = value
}
/**
* @param value A list of one or more SharePoint site URLs.
*/
@JvmName("qixkdevmwwjfrkqw")
public suspend fun siteUrls(`value`: Output>) {
this.siteUrls = value
}
@JvmName("wnmjpsxsgpqboclv")
public suspend fun siteUrls(vararg values: Output) {
this.siteUrls = Output.all(values.asList())
}
/**
* @param values A list of one or more SharePoint site URLs.
*/
@JvmName("yyhxflbmrhmpsbhl")
public suspend fun siteUrls(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy