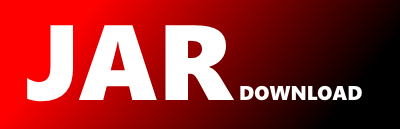
com.pulumi.awsnative.cleanrooms.kotlin.PrivacyBudgetTemplate.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.cleanrooms.kotlin
import com.pulumi.awsnative.cleanrooms.kotlin.enums.PrivacyBudgetTemplateAutoRefresh
import com.pulumi.awsnative.cleanrooms.kotlin.enums.PrivacyBudgetTemplatePrivacyBudgetType
import com.pulumi.awsnative.cleanrooms.kotlin.outputs.ParametersProperties
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.cleanrooms.kotlin.enums.PrivacyBudgetTemplateAutoRefresh.Companion.toKotlin as privacyBudgetTemplateAutoRefreshToKotlin
import com.pulumi.awsnative.cleanrooms.kotlin.enums.PrivacyBudgetTemplatePrivacyBudgetType.Companion.toKotlin as privacyBudgetTemplatePrivacyBudgetTypeToKotlin
import com.pulumi.awsnative.cleanrooms.kotlin.outputs.ParametersProperties.Companion.toKotlin as parametersPropertiesToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [PrivacyBudgetTemplate].
*/
@PulumiTagMarker
public class PrivacyBudgetTemplateResourceBuilder internal constructor() {
public var name: String? = null
public var args: PrivacyBudgetTemplateArgs = PrivacyBudgetTemplateArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend PrivacyBudgetTemplateArgsBuilder.() -> Unit) {
val builder = PrivacyBudgetTemplateArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): PrivacyBudgetTemplate {
val builtJavaResource =
com.pulumi.awsnative.cleanrooms.PrivacyBudgetTemplate(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return PrivacyBudgetTemplate(builtJavaResource)
}
}
/**
* Represents a privacy budget within a collaboration
*/
public class PrivacyBudgetTemplate internal constructor(
override val javaResource: com.pulumi.awsnative.cleanrooms.PrivacyBudgetTemplate,
) : KotlinCustomResource(javaResource, PrivacyBudgetTemplateMapper) {
/**
* The ARN of the privacy budget template.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* How often the privacy budget refreshes.
* > If you plan to regularly bring new data into the collaboration, use `CALENDAR_MONTH` to automatically get a new privacy budget for the collaboration every calendar month. Choosing this option allows arbitrary amounts of information to be revealed about rows of the data when repeatedly queried across refreshes. Avoid choosing this if the same rows will be repeatedly queried between privacy budget refreshes.
*/
public val autoRefresh: Output
get() = javaResource.autoRefresh().applyValue({ args0 ->
args0.let({ args0 ->
privacyBudgetTemplateAutoRefreshToKotlin(args0)
})
})
/**
* The ARN of the collaboration that contains this privacy budget template.
*/
public val collaborationArn: Output
get() = javaResource.collaborationArn().applyValue({ args0 -> args0 })
/**
* The unique ID of the collaboration that contains this privacy budget template.
*/
public val collaborationIdentifier: Output
get() = javaResource.collaborationIdentifier().applyValue({ args0 -> args0 })
/**
* The Amazon Resource Name (ARN) of the member who created the privacy budget template.
*/
public val membershipArn: Output
get() = javaResource.membershipArn().applyValue({ args0 -> args0 })
/**
* The identifier for a membership resource.
*/
public val membershipIdentifier: Output
get() = javaResource.membershipIdentifier().applyValue({ args0 -> args0 })
/**
* Specifies the epsilon and noise parameters for the privacy budget template.
*/
public val parameters: Output
get() = javaResource.parameters().applyValue({ args0 ->
args0.let({ args0 ->
parametersPropertiesToKotlin(args0)
})
})
/**
* A unique identifier for one of your memberships for a collaboration. The privacy budget template is created in the collaboration that this membership belongs to. Accepts a membership ID.
*/
public val privacyBudgetTemplateIdentifier: Output
get() = javaResource.privacyBudgetTemplateIdentifier().applyValue({ args0 -> args0 })
/**
* Specifies the type of the privacy budget template.
*/
public val privacyBudgetType: Output
get() = javaResource.privacyBudgetType().applyValue({ args0 ->
args0.let({ args0 ->
privacyBudgetTemplatePrivacyBudgetTypeToKotlin(args0)
})
})
/**
* An arbitrary set of tags (key-value pairs) for this cleanrooms privacy budget template.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
}
public object PrivacyBudgetTemplateMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.cleanrooms.PrivacyBudgetTemplate::class == javaResource::class
override fun map(javaResource: Resource): PrivacyBudgetTemplate =
PrivacyBudgetTemplate(javaResource as com.pulumi.awsnative.cleanrooms.PrivacyBudgetTemplate)
}
/**
* @see [PrivacyBudgetTemplate].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [PrivacyBudgetTemplate].
*/
public suspend fun privacyBudgetTemplate(
name: String,
block: suspend PrivacyBudgetTemplateResourceBuilder.() -> Unit,
): PrivacyBudgetTemplate {
val builder = PrivacyBudgetTemplateResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [PrivacyBudgetTemplate].
* @param name The _unique_ name of the resulting resource.
*/
public fun privacyBudgetTemplate(name: String): PrivacyBudgetTemplate {
val builder = PrivacyBudgetTemplateResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy