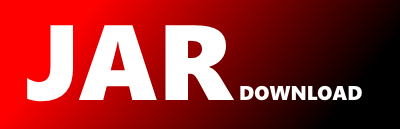
com.pulumi.awsnative.cloudfront.kotlin.Function.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.cloudfront.kotlin
import com.pulumi.awsnative.cloudfront.kotlin.outputs.FunctionConfig
import com.pulumi.awsnative.cloudfront.kotlin.outputs.FunctionMetadata
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.cloudfront.kotlin.outputs.FunctionConfig.Companion.toKotlin as functionConfigToKotlin
import com.pulumi.awsnative.cloudfront.kotlin.outputs.FunctionMetadata.Companion.toKotlin as functionMetadataToKotlin
/**
* Builder for [Function].
*/
@PulumiTagMarker
public class FunctionResourceBuilder internal constructor() {
public var name: String? = null
public var args: FunctionArgs = FunctionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FunctionArgsBuilder.() -> Unit) {
val builder = FunctionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Function {
val builtJavaResource = com.pulumi.awsnative.cloudfront.Function(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Function(builtJavaResource)
}
}
/**
* Resource Type definition for AWS::CloudFront::Function
*/
public class Function internal constructor(
override val javaResource: com.pulumi.awsnative.cloudfront.Function,
) : KotlinCustomResource(javaResource, FunctionMapper) {
/**
* A flag that determines whether to automatically publish the function to the `LIVE` stage when it’s created. To automatically publish to the `LIVE` stage, set this property to `true` .
*/
public val autoPublish: Output?
get() = javaResource.autoPublish().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The ARN of the function. For example:
* `arn:aws:cloudfront::123456789012:function/ExampleFunction` .
* To get the function ARN, use the following syntax:
* `!GetAtt *Function_Logical_ID* .FunctionMetadata.FunctionARN`
*/
public val functionArn: Output
get() = javaResource.functionArn().applyValue({ args0 -> args0 })
/**
* The function code. For more information about writing a CloudFront function, see [Writing function code for CloudFront Functions](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/writing-function-code.html) in the *Amazon CloudFront Developer Guide* .
*/
public val functionCode: Output
get() = javaResource.functionCode().applyValue({ args0 -> args0 })
/**
* Contains configuration information about a CloudFront function.
*/
public val functionConfig: Output
get() = javaResource.functionConfig().applyValue({ args0 ->
args0.let({ args0 ->
functionConfigToKotlin(args0)
})
})
/**
* Contains metadata about a CloudFront function.
*/
public val functionMetadata: Output?
get() = javaResource.functionMetadata().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> functionMetadataToKotlin(args0) })
}).orElse(null)
})
/**
* A name to identify the function.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val stage: Output
get() = javaResource.stage().applyValue({ args0 -> args0 })
}
public object FunctionMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.cloudfront.Function::class == javaResource::class
override fun map(javaResource: Resource): Function = Function(
javaResource as
com.pulumi.awsnative.cloudfront.Function,
)
}
/**
* @see [Function].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Function].
*/
public suspend fun function(name: String, block: suspend FunctionResourceBuilder.() -> Unit): Function {
val builder = FunctionResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Function].
* @param name The _unique_ name of the resulting resource.
*/
public fun function(name: String): Function {
val builder = FunctionResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy