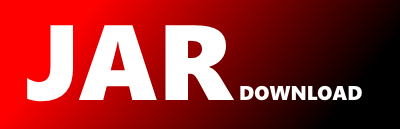
com.pulumi.awsnative.cloudfront.kotlin.inputs.DistributionLambdaFunctionAssociationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.cloudfront.kotlin.inputs
import com.pulumi.awsnative.cloudfront.inputs.DistributionLambdaFunctionAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A complex type that contains a Lambda@Edge function association.
* @property eventType Specifies the event type that triggers a Lambda@Edge function invocation. You can specify the following values:
* + ``viewer-request``: The function executes when CloudFront receives a request from a viewer and before it checks to see whether the requested object is in the edge cache.
* + ``origin-request``: The function executes only when CloudFront sends a request to your origin. When the requested object is in the edge cache, the function doesn't execute.
* + ``origin-response``: The function executes after CloudFront receives a response from the origin and before it caches the object in the response. When the requested object is in the edge cache, the function doesn't execute.
* + ``viewer-response``: The function executes before CloudFront returns the requested object to the viewer. The function executes regardless of whether the object was already in the edge cache.
* If the origin returns an HTTP status code other than HTTP 200 (OK), the function doesn't execute.
* @property includeBody A flag that allows a Lambda@Edge function to have read access to the body content. For more information, see [Accessing the Request Body by Choosing the Include Body Option](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/lambda-include-body-access.html) in the Amazon CloudFront Developer Guide.
* @property lambdaFunctionArn The ARN of the Lambda@Edge function. You must specify the ARN of a function version; you can't specify an alias or $LATEST.
*/
public data class DistributionLambdaFunctionAssociationArgs(
public val eventType: Output? = null,
public val includeBody: Output? = null,
public val lambdaFunctionArn: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.cloudfront.inputs.DistributionLambdaFunctionAssociationArgs =
com.pulumi.awsnative.cloudfront.inputs.DistributionLambdaFunctionAssociationArgs.builder()
.eventType(eventType?.applyValue({ args0 -> args0 }))
.includeBody(includeBody?.applyValue({ args0 -> args0 }))
.lambdaFunctionArn(lambdaFunctionArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DistributionLambdaFunctionAssociationArgs].
*/
@PulumiTagMarker
public class DistributionLambdaFunctionAssociationArgsBuilder internal constructor() {
private var eventType: Output? = null
private var includeBody: Output? = null
private var lambdaFunctionArn: Output? = null
/**
* @param value Specifies the event type that triggers a Lambda@Edge function invocation. You can specify the following values:
* + ``viewer-request``: The function executes when CloudFront receives a request from a viewer and before it checks to see whether the requested object is in the edge cache.
* + ``origin-request``: The function executes only when CloudFront sends a request to your origin. When the requested object is in the edge cache, the function doesn't execute.
* + ``origin-response``: The function executes after CloudFront receives a response from the origin and before it caches the object in the response. When the requested object is in the edge cache, the function doesn't execute.
* + ``viewer-response``: The function executes before CloudFront returns the requested object to the viewer. The function executes regardless of whether the object was already in the edge cache.
* If the origin returns an HTTP status code other than HTTP 200 (OK), the function doesn't execute.
*/
@JvmName("qsneyvljdrolaomi")
public suspend fun eventType(`value`: Output) {
this.eventType = value
}
/**
* @param value A flag that allows a Lambda@Edge function to have read access to the body content. For more information, see [Accessing the Request Body by Choosing the Include Body Option](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/lambda-include-body-access.html) in the Amazon CloudFront Developer Guide.
*/
@JvmName("eaoeqpbyjhblbavt")
public suspend fun includeBody(`value`: Output) {
this.includeBody = value
}
/**
* @param value The ARN of the Lambda@Edge function. You must specify the ARN of a function version; you can't specify an alias or $LATEST.
*/
@JvmName("uydotnonkxivqstj")
public suspend fun lambdaFunctionArn(`value`: Output) {
this.lambdaFunctionArn = value
}
/**
* @param value Specifies the event type that triggers a Lambda@Edge function invocation. You can specify the following values:
* + ``viewer-request``: The function executes when CloudFront receives a request from a viewer and before it checks to see whether the requested object is in the edge cache.
* + ``origin-request``: The function executes only when CloudFront sends a request to your origin. When the requested object is in the edge cache, the function doesn't execute.
* + ``origin-response``: The function executes after CloudFront receives a response from the origin and before it caches the object in the response. When the requested object is in the edge cache, the function doesn't execute.
* + ``viewer-response``: The function executes before CloudFront returns the requested object to the viewer. The function executes regardless of whether the object was already in the edge cache.
* If the origin returns an HTTP status code other than HTTP 200 (OK), the function doesn't execute.
*/
@JvmName("qwiquswnlxkdiqdb")
public suspend fun eventType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventType = mapped
}
/**
* @param value A flag that allows a Lambda@Edge function to have read access to the body content. For more information, see [Accessing the Request Body by Choosing the Include Body Option](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/lambda-include-body-access.html) in the Amazon CloudFront Developer Guide.
*/
@JvmName("tfjaeavhtnslacnc")
public suspend fun includeBody(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeBody = mapped
}
/**
* @param value The ARN of the Lambda@Edge function. You must specify the ARN of a function version; you can't specify an alias or $LATEST.
*/
@JvmName("tarpdoympefyghoc")
public suspend fun lambdaFunctionArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lambdaFunctionArn = mapped
}
internal fun build(): DistributionLambdaFunctionAssociationArgs =
DistributionLambdaFunctionAssociationArgs(
eventType = eventType,
includeBody = includeBody,
lambdaFunctionArn = lambdaFunctionArn,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy