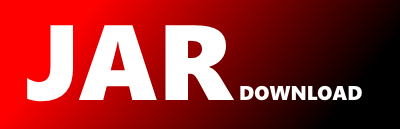
com.pulumi.awsnative.cloudtrail.kotlin.ResourcePolicyArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.cloudtrail.kotlin
import com.pulumi.awsnative.cloudtrail.ResourcePolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::CloudTrail::ResourcePolicy
* @property resourceArn The ARN of the AWS CloudTrail resource to which the policy applies.
* @property resourcePolicy A policy document containing permissions to add to the specified resource. In IAM, you must provide policy documents in JSON format. However, in CloudFormation you can provide the policy in JSON or YAML format because CloudFormation converts YAML to JSON before submitting it to IAM.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::CloudTrail::ResourcePolicy` for more information about the expected schema for this property.
*/
public data class ResourcePolicyArgs(
public val resourceArn: Output? = null,
public val resourcePolicy: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.cloudtrail.ResourcePolicyArgs =
com.pulumi.awsnative.cloudtrail.ResourcePolicyArgs.builder()
.resourceArn(resourceArn?.applyValue({ args0 -> args0 }))
.resourcePolicy(resourcePolicy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ResourcePolicyArgs].
*/
@PulumiTagMarker
public class ResourcePolicyArgsBuilder internal constructor() {
private var resourceArn: Output? = null
private var resourcePolicy: Output? = null
/**
* @param value The ARN of the AWS CloudTrail resource to which the policy applies.
*/
@JvmName("nxipnumfoeieqnky")
public suspend fun resourceArn(`value`: Output) {
this.resourceArn = value
}
/**
* @param value A policy document containing permissions to add to the specified resource. In IAM, you must provide policy documents in JSON format. However, in CloudFormation you can provide the policy in JSON or YAML format because CloudFormation converts YAML to JSON before submitting it to IAM.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::CloudTrail::ResourcePolicy` for more information about the expected schema for this property.
*/
@JvmName("ffgpsuioasriomlj")
public suspend fun resourcePolicy(`value`: Output) {
this.resourcePolicy = value
}
/**
* @param value The ARN of the AWS CloudTrail resource to which the policy applies.
*/
@JvmName("oavvcbwjsjjrfjfg")
public suspend fun resourceArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceArn = mapped
}
/**
* @param value A policy document containing permissions to add to the specified resource. In IAM, you must provide policy documents in JSON format. However, in CloudFormation you can provide the policy in JSON or YAML format because CloudFormation converts YAML to JSON before submitting it to IAM.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::CloudTrail::ResourcePolicy` for more information about the expected schema for this property.
*/
@JvmName("escutlhjphoasbwt")
public suspend fun resourcePolicy(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourcePolicy = mapped
}
internal fun build(): ResourcePolicyArgs = ResourcePolicyArgs(
resourceArn = resourceArn,
resourcePolicy = resourcePolicy,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy