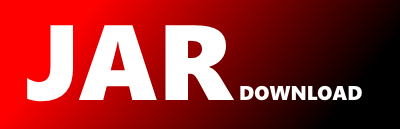
com.pulumi.awsnative.cloudtrail.kotlin.Trail.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.cloudtrail.kotlin
import com.pulumi.awsnative.cloudtrail.kotlin.outputs.TrailAdvancedEventSelector
import com.pulumi.awsnative.cloudtrail.kotlin.outputs.TrailEventSelector
import com.pulumi.awsnative.cloudtrail.kotlin.outputs.TrailInsightSelector
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.cloudtrail.kotlin.outputs.TrailAdvancedEventSelector.Companion.toKotlin as trailAdvancedEventSelectorToKotlin
import com.pulumi.awsnative.cloudtrail.kotlin.outputs.TrailEventSelector.Companion.toKotlin as trailEventSelectorToKotlin
import com.pulumi.awsnative.cloudtrail.kotlin.outputs.TrailInsightSelector.Companion.toKotlin as trailInsightSelectorToKotlin
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
/**
* Builder for [Trail].
*/
@PulumiTagMarker
public class TrailResourceBuilder internal constructor() {
public var name: String? = null
public var args: TrailArgs = TrailArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TrailArgsBuilder.() -> Unit) {
val builder = TrailArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Trail {
val builtJavaResource = com.pulumi.awsnative.cloudtrail.Trail(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Trail(builtJavaResource)
}
}
/**
* Creates a trail that specifies the settings for delivery of log data to an Amazon S3 bucket. A maximum of five trails can exist in a region, irrespective of the region in which they were created.
*/
public class Trail internal constructor(
override val javaResource: com.pulumi.awsnative.cloudtrail.Trail,
) : KotlinCustomResource(javaResource, TrailMapper) {
/**
* The advanced event selectors that were used to select events for the data store.
*/
public val advancedEventSelectors: Output>?
get() = javaResource.advancedEventSelectors().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
trailAdvancedEventSelectorToKotlin(args0)
})
})
}).orElse(null)
})
/**
* `Ref` returns the ARN of the CloudTrail trail, such as `arn:aws:cloudtrail:us-east-2:123456789012:trail/myCloudTrail` .
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Specifies a log group name using an Amazon Resource Name (ARN), a unique identifier that represents the log group to which CloudTrail logs will be delivered. Not required unless you specify CloudWatchLogsRoleArn.
*/
public val cloudWatchLogsLogGroupArn: Output?
get() = javaResource.cloudWatchLogsLogGroupArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the role for the CloudWatch Logs endpoint to assume to write to a user's log group.
*/
public val cloudWatchLogsRoleArn: Output?
get() = javaResource.cloudWatchLogsRoleArn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether log file validation is enabled. The default is false.
*/
public val enableLogFileValidation: Output?
get() = javaResource.enableLogFileValidation().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Use event selectors to further specify the management and data event settings for your trail. By default, trails created without specific event selectors will be configured to log all read and write management events, and no data events. When an event occurs in your account, CloudTrail evaluates the event selector for all trails. For each trail, if the event matches any event selector, the trail processes and logs the event. If the event doesn't match any event selector, the trail doesn't log the event. You can configure up to five event selectors for a trail.
*/
public val eventSelectors: Output>?
get() = javaResource.eventSelectors().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
trailEventSelectorToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Specifies whether the trail is publishing events from global services such as IAM to the log files.
*/
public val includeGlobalServiceEvents: Output?
get() = javaResource.includeGlobalServiceEvents().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Lets you enable Insights event logging by specifying the Insights selectors that you want to enable on an existing trail.
*/
public val insightSelectors: Output>?
get() = javaResource.insightSelectors().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
trailInsightSelectorToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Whether the CloudTrail is currently logging AWS API calls.
*/
public val isLogging: Output
get() = javaResource.isLogging().applyValue({ args0 -> args0 })
/**
* Specifies whether the trail applies only to the current region or to all regions. The default is false. If the trail exists only in the current region and this value is set to true, shadow trails (replications of the trail) will be created in the other regions. If the trail exists in all regions and this value is set to false, the trail will remain in the region where it was created, and its shadow trails in other regions will be deleted. As a best practice, consider using trails that log events in all regions.
*/
public val isMultiRegionTrail: Output?
get() = javaResource.isMultiRegionTrail().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether the trail is created for all accounts in an organization in AWS Organizations, or only for the current AWS account. The default is false, and cannot be true unless the call is made on behalf of an AWS account that is the master account for an organization in AWS Organizations.
*/
public val isOrganizationTrail: Output?
get() = javaResource.isOrganizationTrail().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies the KMS key ID to use to encrypt the logs delivered by CloudTrail. The value can be an alias name prefixed by 'alias/', a fully specified ARN to an alias, a fully specified ARN to a key, or a globally unique identifier.
*/
public val kmsKeyId: Output?
get() = javaResource.kmsKeyId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies the name of the Amazon S3 bucket designated for publishing log files. See Amazon S3 Bucket Naming Requirements.
*/
public val s3BucketName: Output
get() = javaResource.s3BucketName().applyValue({ args0 -> args0 })
/**
* Specifies the Amazon S3 key prefix that comes after the name of the bucket you have designated for log file delivery. For more information, see Finding Your CloudTrail Log Files. The maximum length is 200 characters.
*/
public val s3KeyPrefix: Output?
get() = javaResource.s3KeyPrefix().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* `Ref` returns the ARN of the Amazon SNS topic that's associated with the CloudTrail trail, such as `arn:aws:sns:us-east-2:123456789012:mySNSTopic` .
*/
public val snsTopicArn: Output
get() = javaResource.snsTopicArn().applyValue({ args0 -> args0 })
/**
* Specifies the name of the Amazon SNS topic defined for notification of log file delivery. The maximum length is 256 characters.
*/
public val snsTopicName: Output?
get() = javaResource.snsTopicName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A custom set of tags (key-value pairs) for this trail.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* Specifies the name of the trail. The name must meet the following requirements:
* - Contain only ASCII letters (a-z, A-Z), numbers (0-9), periods (.), underscores (_), or dashes (-)
* - Start with a letter or number, and end with a letter or number
* - Be between 3 and 128 characters
* - Have no adjacent periods, underscores or dashes. Names like `my-_namespace` and `my--namespace` are not valid.
* - Not be in IP address format (for example, 192.168.5.4)
*/
public val trailName: Output?
get() = javaResource.trailName().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object TrailMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.cloudtrail.Trail::class == javaResource::class
override fun map(javaResource: Resource): Trail = Trail(
javaResource as
com.pulumi.awsnative.cloudtrail.Trail,
)
}
/**
* @see [Trail].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Trail].
*/
public suspend fun trail(name: String, block: suspend TrailResourceBuilder.() -> Unit): Trail {
val builder = TrailResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Trail].
* @param name The _unique_ name of the resulting resource.
*/
public fun trail(name: String): Trail {
val builder = TrailResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy