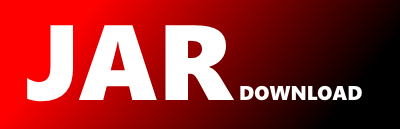
com.pulumi.awsnative.codebuild.kotlin.FleetArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.codebuild.kotlin
import com.pulumi.awsnative.codebuild.FleetArgs.builder
import com.pulumi.awsnative.codebuild.kotlin.enums.FleetComputeType
import com.pulumi.awsnative.codebuild.kotlin.enums.FleetEnvironmentType
import com.pulumi.awsnative.codebuild.kotlin.enums.FleetOverflowBehavior
import com.pulumi.awsnative.codebuild.kotlin.inputs.FleetVpcConfigArgs
import com.pulumi.awsnative.codebuild.kotlin.inputs.FleetVpcConfigArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::CodeBuild::Fleet
* @property baseCapacity The initial number of machines allocated to the compute fleet, which defines the number of builds that can run in parallel.
* @property computeType > Updating this field is not allowed for `MAC_ARM` .
* Information about the compute resources the compute fleet uses. Available values include:
* - `BUILD_GENERAL1_SMALL` : Use up to 3 GB memory and 2 vCPUs for builds.
* - `BUILD_GENERAL1_MEDIUM` : Use up to 7 GB memory and 4 vCPUs for builds.
* - `BUILD_GENERAL1_LARGE` : Use up to 16 GB memory and 8 vCPUs for builds, depending on your environment type.
* - `BUILD_GENERAL1_XLARGE` : Use up to 70 GB memory and 36 vCPUs for builds, depending on your environment type.
* - `BUILD_GENERAL1_2XLARGE` : Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for builds. This compute type supports Docker images up to 100 GB uncompressed.
* If you use `BUILD_GENERAL1_SMALL` :
* - For environment type `LINUX_CONTAINER` , you can use up to 3 GB memory and 2 vCPUs for builds.
* - For environment type `LINUX_GPU_CONTAINER` , you can use up to 16 GB memory, 4 vCPUs, and 1 NVIDIA A10G Tensor Core GPU for builds.
* - For environment type `ARM_CONTAINER` , you can use up to 4 GB memory and 2 vCPUs on ARM-based processors for builds.
* If you use `BUILD_GENERAL1_LARGE` :
* - For environment type `LINUX_CONTAINER` , you can use up to 15 GB memory and 8 vCPUs for builds.
* - For environment type `LINUX_GPU_CONTAINER` , you can use up to 255 GB memory, 32 vCPUs, and 4 NVIDIA Tesla V100 GPUs for builds.
* - For environment type `ARM_CONTAINER` , you can use up to 16 GB memory and 8 vCPUs on ARM-based processors for builds.
* For more information, see [Build environment compute types](https://docs.aws.amazon.com/codebuild/latest/userguide/build-env-ref-compute-types.html) in the *AWS CodeBuild User Guide.*
* @property environmentType > Updating this field is not allowed for `MAC_ARM` .
* The environment type of the compute fleet.
* - The environment type `ARM_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific (Singapore), Asia Pacific (Sydney), EU (Frankfurt), and South America (São Paulo).
* - The environment type `LINUX_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), EU (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Singapore), Asia Pacific (Sydney), South America (São Paulo), and Asia Pacific (Mumbai).
* - The environment type `LINUX_GPU_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), EU (Frankfurt), Asia Pacific (Tokyo), and Asia Pacific (Sydney).
* - The environment type `WINDOWS_SERVER_2019_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), Asia Pacific (Sydney), Asia Pacific (Tokyo), Asia Pacific (Mumbai) and EU (Ireland).
* - The environment type `WINDOWS_SERVER_2022_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), EU (Frankfurt), Asia Pacific (Sydney), Asia Pacific (Singapore), Asia Pacific (Tokyo), South America (São Paulo) and Asia Pacific (Mumbai).
* For more information, see [Build environment compute types](https://docs.aws.amazon.com//codebuild/latest/userguide/build-env-ref-compute-types.html) in the *AWS CodeBuild user guide* .
* @property fleetServiceRole The service role associated with the compute fleet. For more information, see [Allow a user to add a permission policy for a fleet service role](https://docs.aws.amazon.com/codebuild/latest/userguide/auth-and-access-control-iam-identity-based-access-control.html#customer-managed-policies-example-permission-policy-fleet-service-role.html) in the *AWS CodeBuild User Guide* .
* @property fleetVpcConfig > Updating this field is not allowed for `MAC_ARM` .
* Information about the VPC configuration that AWS CodeBuild accesses.
* @property imageId > Updating this field is not allowed for `MAC_ARM` .
* The Amazon Machine Image (AMI) of the compute fleet.
* @property name The name of the compute fleet.
* @property overflowBehavior The compute fleet overflow behavior.
* - For overflow behavior `QUEUE` , your overflow builds need to wait on the existing fleet instance to become available.
* - For overflow behavior `ON_DEMAND` , your overflow builds run on CodeBuild on-demand.
* > If you choose to set your overflow behavior to on-demand while creating a VPC-connected fleet, make sure that you add the required VPC permissions to your project service role. For more information, see [Example policy statement to allow CodeBuild access to AWS services required to create a VPC network interface](https://docs.aws.amazon.com/codebuild/latest/userguide/auth-and-access-control-iam-identity-based-access-control.html#customer-managed-policies-example-create-vpc-network-interface) .
* @property tags A list of tag key and value pairs associated with this compute fleet.
* These tags are available for use by AWS services that support AWS CodeBuild compute fleet tags.
*/
public data class FleetArgs(
public val baseCapacity: Output? = null,
public val computeType: Output? = null,
public val environmentType: Output? = null,
public val fleetServiceRole: Output? = null,
public val fleetVpcConfig: Output? = null,
public val imageId: Output? = null,
public val name: Output? = null,
public val overflowBehavior: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.codebuild.FleetArgs =
com.pulumi.awsnative.codebuild.FleetArgs.builder()
.baseCapacity(baseCapacity?.applyValue({ args0 -> args0 }))
.computeType(computeType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.environmentType(environmentType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fleetServiceRole(fleetServiceRole?.applyValue({ args0 -> args0 }))
.fleetVpcConfig(fleetVpcConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.imageId(imageId?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.overflowBehavior(overflowBehavior?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [FleetArgs].
*/
@PulumiTagMarker
public class FleetArgsBuilder internal constructor() {
private var baseCapacity: Output? = null
private var computeType: Output? = null
private var environmentType: Output? = null
private var fleetServiceRole: Output? = null
private var fleetVpcConfig: Output? = null
private var imageId: Output? = null
private var name: Output? = null
private var overflowBehavior: Output? = null
private var tags: Output>? = null
/**
* @param value The initial number of machines allocated to the compute fleet, which defines the number of builds that can run in parallel.
*/
@JvmName("hlltfcxnahpvgmrg")
public suspend fun baseCapacity(`value`: Output) {
this.baseCapacity = value
}
/**
* @param value > Updating this field is not allowed for `MAC_ARM` .
* Information about the compute resources the compute fleet uses. Available values include:
* - `BUILD_GENERAL1_SMALL` : Use up to 3 GB memory and 2 vCPUs for builds.
* - `BUILD_GENERAL1_MEDIUM` : Use up to 7 GB memory and 4 vCPUs for builds.
* - `BUILD_GENERAL1_LARGE` : Use up to 16 GB memory and 8 vCPUs for builds, depending on your environment type.
* - `BUILD_GENERAL1_XLARGE` : Use up to 70 GB memory and 36 vCPUs for builds, depending on your environment type.
* - `BUILD_GENERAL1_2XLARGE` : Use up to 145 GB memory, 72 vCPUs, and 824 GB of SSD storage for builds. This compute type supports Docker images up to 100 GB uncompressed.
* If you use `BUILD_GENERAL1_SMALL` :
* - For environment type `LINUX_CONTAINER` , you can use up to 3 GB memory and 2 vCPUs for builds.
* - For environment type `LINUX_GPU_CONTAINER` , you can use up to 16 GB memory, 4 vCPUs, and 1 NVIDIA A10G Tensor Core GPU for builds.
* - For environment type `ARM_CONTAINER` , you can use up to 4 GB memory and 2 vCPUs on ARM-based processors for builds.
* If you use `BUILD_GENERAL1_LARGE` :
* - For environment type `LINUX_CONTAINER` , you can use up to 15 GB memory and 8 vCPUs for builds.
* - For environment type `LINUX_GPU_CONTAINER` , you can use up to 255 GB memory, 32 vCPUs, and 4 NVIDIA Tesla V100 GPUs for builds.
* - For environment type `ARM_CONTAINER` , you can use up to 16 GB memory and 8 vCPUs on ARM-based processors for builds.
* For more information, see [Build environment compute types](https://docs.aws.amazon.com/codebuild/latest/userguide/build-env-ref-compute-types.html) in the *AWS CodeBuild User Guide.*
*/
@JvmName("ixfgdjafqsrehpto")
public suspend fun computeType(`value`: Output) {
this.computeType = value
}
/**
* @param value > Updating this field is not allowed for `MAC_ARM` .
* The environment type of the compute fleet.
* - The environment type `ARM_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), Asia Pacific (Mumbai), Asia Pacific (Tokyo), Asia Pacific (Singapore), Asia Pacific (Sydney), EU (Frankfurt), and South America (São Paulo).
* - The environment type `LINUX_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), EU (Frankfurt), Asia Pacific (Tokyo), Asia Pacific (Singapore), Asia Pacific (Sydney), South America (São Paulo), and Asia Pacific (Mumbai).
* - The environment type `LINUX_GPU_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), EU (Frankfurt), Asia Pacific (Tokyo), and Asia Pacific (Sydney).
* - The environment type `WINDOWS_SERVER_2019_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), Asia Pacific (Sydney), Asia Pacific (Tokyo), Asia Pacific (Mumbai) and EU (Ireland).
* - The environment type `WINDOWS_SERVER_2022_CONTAINER` is available only in regions US East (N. Virginia), US East (Ohio), US West (Oregon), EU (Ireland), EU (Frankfurt), Asia Pacific (Sydney), Asia Pacific (Singapore), Asia Pacific (Tokyo), South America (São Paulo) and Asia Pacific (Mumbai).
* For more information, see [Build environment compute types](https://docs.aws.amazon.com//codebuild/latest/userguide/build-env-ref-compute-types.html) in the *AWS CodeBuild user guide* .
*/
@JvmName("unicqyuxcsdcsdhs")
public suspend fun environmentType(`value`: Output) {
this.environmentType = value
}
/**
* @param value The service role associated with the compute fleet. For more information, see [Allow a user to add a permission policy for a fleet service role](https://docs.aws.amazon.com/codebuild/latest/userguide/auth-and-access-control-iam-identity-based-access-control.html#customer-managed-policies-example-permission-policy-fleet-service-role.html) in the *AWS CodeBuild User Guide* .
*/
@JvmName("pnewefxwebjxlfhc")
public suspend fun fleetServiceRole(`value`: Output) {
this.fleetServiceRole = value
}
/**
* @param value > Updating this field is not allowed for `MAC_ARM` .
* Information about the VPC configuration that AWS CodeBuild accesses.
*/
@JvmName("nvakljrrunldoqok")
public suspend fun fleetVpcConfig(`value`: Output) {
this.fleetVpcConfig = value
}
/**
* @param value > Updating this field is not allowed for `MAC_ARM` .
* The Amazon Machine Image (AMI) of the compute fleet.
*/
@JvmName("nlgmtsbrbeytxyhg")
public suspend fun imageId(`value`: Output) {
this.imageId = value
}
/**
* @param value The name of the compute fleet.
*/
@JvmName("ovfileukkbqbnmfn")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The compute fleet overflow behavior.
* - For overflow behavior `QUEUE` , your overflow builds need to wait on the existing fleet instance to become available.
* - For overflow behavior `ON_DEMAND` , your overflow builds run on CodeBuild on-demand.
* > If you choose to set your overflow behavior to on-demand while creating a VPC-connected fleet, make sure that you add the required VPC permissions to your project service role. For more information, see [Example policy statement to allow CodeBuild access to AWS services required to create a VPC network interface](https://docs.aws.amazon.com/codebuild/latest/userguide/auth-and-access-control-iam-identity-based-access-control.html#customer-managed-policies-example-create-vpc-network-interface) .
*/
@JvmName("lagynkkjbybkcfvq")
public suspend fun overflowBehavior(`value`: Output) {
this.overflowBehavior = value
}
/**
* @param value A list of tag key and value pairs associated with this compute fleet.
* These tags are available for use by AWS services that support AWS CodeBuild compute fleet tags.
*/
@JvmName("mhwqcjqvpauodmci")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("cxfsdrxyacekljqh")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values A list of tag key and value pairs associated with this compute fleet.
* These tags are available for use by AWS services that support AWS CodeBuild compute fleet tags.
*/
@JvmName("twbymsbgurxfwkfb")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy