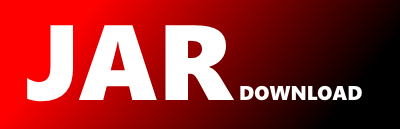
com.pulumi.awsnative.cognito.kotlin.IdentityPoolRoleAttachmentArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.cognito.kotlin
import com.pulumi.awsnative.cognito.IdentityPoolRoleAttachmentArgs.builder
import com.pulumi.awsnative.cognito.kotlin.inputs.IdentityPoolRoleAttachmentRoleMappingArgs
import com.pulumi.awsnative.cognito.kotlin.inputs.IdentityPoolRoleAttachmentRoleMappingArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Cognito::IdentityPoolRoleAttachment
* @property identityPoolId An identity pool ID in the format `REGION:GUID` .
* @property roleMappings How users for a specific identity provider are mapped to roles. This is a string to the `RoleMapping` object map. The string identifies the identity provider. For example: `graph.facebook.com` or `cognito-idp.us-east-1.amazonaws.com/us-east-1_abcdefghi:app_client_id` .
* If the `IdentityProvider` field isn't provided in this object, the string is used as the identity provider name.
* For more information, see the [RoleMapping property](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-cognito-identitypoolroleattachment-rolemapping.html) .
* @property roles The map of the roles associated with this pool. For a given role, the key is either "authenticated" or "unauthenticated". The value is the role ARN.
*/
public data class IdentityPoolRoleAttachmentArgs(
public val identityPoolId: Output? = null,
public val roleMappings: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy