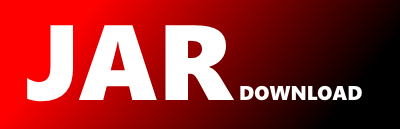
com.pulumi.awsnative.configuration.kotlin.ConfigRuleArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.configuration.kotlin
import com.pulumi.awsnative.configuration.ConfigRuleArgs.builder
import com.pulumi.awsnative.configuration.kotlin.inputs.CompliancePropertiesArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.CompliancePropertiesArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.ConfigRuleEvaluationModeConfigurationArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.ConfigRuleEvaluationModeConfigurationArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.ConfigRuleScopeArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.ConfigRuleScopeArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.ConfigRuleSourceArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.ConfigRuleSourceArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* You must first create and start the CC configuration recorder in order to create CC managed rules with CFNlong. For more information, see [Managing the Configuration Recorder](https://docs.aws.amazon.com/config/latest/developerguide/stop-start-recorder.html).
* Adds or updates an CC rule to evaluate if your AWS resources comply with your desired configurations. For information on how many CC rules you can have per account, see [Service Limits](https://docs.aws.amazon.com/config/latest/developerguide/configlimits.html) in the *Developer Guide*.
* There are two types of rules: *Managed Rules* and *Custom Rules*. You can use the ``ConfigRule`` resource to create both CC Managed Rules and CC Custom Rules.
* CC Managed Rules are predefined, customizable rules created by CC. For a list of managed rules, see [List of Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/managed-rules-by-aws-config.html). If you are adding an CC managed rule, you must specify the rule's identifier for the ``SourceIdentifier`` key.
* CC Custom Rules are rules that you create from scratch. There are two ways to create CC custom rules: with Lambda functions ([Developer Guide](https://docs.aws.amazon.com/config/latest/developerguide/gettingstarted-concepts.html#gettingstarted-concepts-function)) and with CFNGUARDshort ([Guard GitHub Repository](https://docs.aws.amazon.com/https://github.com/aws-cloudformation/cloudformation-guard)), a policy-as-code language. CC custom rules created with LAMlong are called *Custom Lambda Rules* and CC custom rules created with CFNGUARDshort are called *Custom Policy Rules*.
* If you are adding a new CC Custom LAM rule, you first need to create an LAMlong function that the rule invokes to evaluate your resources. When you use the ``ConfigRule`` resource to add a Custom LAM rule to CC, you must specify the Amazon Resource Name (ARN) that LAMlong assigns to the function. You specify the ARN in the ``SourceIdentifier`` key. This key is part of the ``Source`` object, which is part of the ``ConfigRule`` object.
* For any new CC rule that you add, specify the ``ConfigRuleName`` in the ``ConfigRule`` object. Do not specify the ``ConfigRuleArn`` or the ``ConfigRuleId``. These values are generated by CC for new rules.
* If you are updating a rule that you added previously, you can specify the rule by ``ConfigRuleName``, ``ConfigRuleId``, or ``ConfigRuleArn`` in the ``ConfigRule`` data type that you use in this request.
* For more information about developing and using CC rules, see [Evaluating Resources with Rules](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config.html) in the *Developer Guide*.
* @property compliance Indicates whether an AWS resource or CC rule is compliant and provides the number of contributors that affect the compliance.
* @property configRuleName A name for the CC rule. If you don't specify a name, CFN generates a unique physical ID and uses that ID for the rule name. For more information, see [Name Type](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-name.html).
* @property description The description that you provide for the CC rule.
* @property evaluationModes The modes the CC rule can be evaluated in. The valid values are distinct objects. By default, the value is Detective evaluation mode only.
* @property inputParameters A string, in JSON format, that is passed to the CC rule Lambda function.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::Config::ConfigRule` for more information about the expected schema for this property.
* @property maximumExecutionFrequency The maximum frequency with which CC runs evaluations for a rule. You can specify a value for ``MaximumExecutionFrequency`` when:
* + You are using an AWS managed rule that is triggered at a periodic frequency.
* + Your custom rule is triggered when CC delivers the configuration snapshot. For more information, see [ConfigSnapshotDeliveryProperties](https://docs.aws.amazon.com/config/latest/APIReference/API_ConfigSnapshotDeliveryProperties.html).
* By default, rules with a periodic trigger are evaluated every 24 hours. To change the frequency, specify a valid value for the ``MaximumExecutionFrequency`` parameter.
* @property scope Defines which resources can trigger an evaluation for the rule. The scope can include one or more resource types, a combination of one resource type and one resource ID, or a combination of a tag key and value. Specify a scope to constrain the resources that can trigger an evaluation for the rule. If you do not specify a scope, evaluations are triggered when any resource in the recording group changes.
* The scope can be empty.
* @property source Provides the rule owner (```` for managed rules, ``CUSTOM_POLICY`` for Custom Policy rules, and ``CUSTOM_LAMBDA`` for Custom Lambda rules), the rule identifier, and the notifications that cause the function to evaluate your AWS resources.
*/
public data class ConfigRuleArgs(
public val compliance: Output? = null,
public val configRuleName: Output? = null,
public val description: Output? = null,
public val evaluationModes: Output>? = null,
public val inputParameters: Output? = null,
public val maximumExecutionFrequency: Output? = null,
public val scope: Output? = null,
public val source: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.configuration.ConfigRuleArgs =
com.pulumi.awsnative.configuration.ConfigRuleArgs.builder()
.compliance(compliance?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.configRuleName(configRuleName?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.evaluationModes(
evaluationModes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.inputParameters(inputParameters?.applyValue({ args0 -> args0 }))
.maximumExecutionFrequency(maximumExecutionFrequency?.applyValue({ args0 -> args0 }))
.scope(scope?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.source(source?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ConfigRuleArgs].
*/
@PulumiTagMarker
public class ConfigRuleArgsBuilder internal constructor() {
private var compliance: Output? = null
private var configRuleName: Output? = null
private var description: Output? = null
private var evaluationModes: Output>? = null
private var inputParameters: Output? = null
private var maximumExecutionFrequency: Output? = null
private var scope: Output? = null
private var source: Output? = null
/**
* @param value Indicates whether an AWS resource or CC rule is compliant and provides the number of contributors that affect the compliance.
*/
@JvmName("quhoctuughgshjha")
public suspend fun compliance(`value`: Output) {
this.compliance = value
}
/**
* @param value A name for the CC rule. If you don't specify a name, CFN generates a unique physical ID and uses that ID for the rule name. For more information, see [Name Type](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-name.html).
*/
@JvmName("tsyradglebkerfho")
public suspend fun configRuleName(`value`: Output) {
this.configRuleName = value
}
/**
* @param value The description that you provide for the CC rule.
*/
@JvmName("ituggmlyydqmnyqp")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The modes the CC rule can be evaluated in. The valid values are distinct objects. By default, the value is Detective evaluation mode only.
*/
@JvmName("ahfuinuqonjkrdov")
public suspend fun evaluationModes(`value`: Output>) {
this.evaluationModes = value
}
@JvmName("djnwtkphwghoqxcy")
public suspend fun evaluationModes(vararg values: Output) {
this.evaluationModes = Output.all(values.asList())
}
/**
* @param values The modes the CC rule can be evaluated in. The valid values are distinct objects. By default, the value is Detective evaluation mode only.
*/
@JvmName("rncpwtwhcjbhdqal")
public suspend fun evaluationModes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy