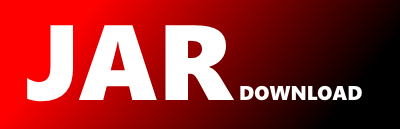
com.pulumi.awsnative.configuration.kotlin.ConfigurationFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.configuration.kotlin
import com.pulumi.awsnative.configuration.ConfigurationFunctions.getAggregationAuthorizationPlain
import com.pulumi.awsnative.configuration.ConfigurationFunctions.getConfigRulePlain
import com.pulumi.awsnative.configuration.ConfigurationFunctions.getConfigurationAggregatorPlain
import com.pulumi.awsnative.configuration.ConfigurationFunctions.getConformancePackPlain
import com.pulumi.awsnative.configuration.ConfigurationFunctions.getOrganizationConformancePackPlain
import com.pulumi.awsnative.configuration.ConfigurationFunctions.getStoredQueryPlain
import com.pulumi.awsnative.configuration.kotlin.inputs.GetAggregationAuthorizationPlainArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.GetAggregationAuthorizationPlainArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.GetConfigRulePlainArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.GetConfigRulePlainArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.GetConfigurationAggregatorPlainArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.GetConfigurationAggregatorPlainArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.GetConformancePackPlainArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.GetConformancePackPlainArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.GetOrganizationConformancePackPlainArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.GetOrganizationConformancePackPlainArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.inputs.GetStoredQueryPlainArgs
import com.pulumi.awsnative.configuration.kotlin.inputs.GetStoredQueryPlainArgsBuilder
import com.pulumi.awsnative.configuration.kotlin.outputs.GetAggregationAuthorizationResult
import com.pulumi.awsnative.configuration.kotlin.outputs.GetConfigRuleResult
import com.pulumi.awsnative.configuration.kotlin.outputs.GetConfigurationAggregatorResult
import com.pulumi.awsnative.configuration.kotlin.outputs.GetConformancePackResult
import com.pulumi.awsnative.configuration.kotlin.outputs.GetOrganizationConformancePackResult
import com.pulumi.awsnative.configuration.kotlin.outputs.GetStoredQueryResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.configuration.kotlin.outputs.GetAggregationAuthorizationResult.Companion.toKotlin as getAggregationAuthorizationResultToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.GetConfigRuleResult.Companion.toKotlin as getConfigRuleResultToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.GetConfigurationAggregatorResult.Companion.toKotlin as getConfigurationAggregatorResultToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.GetConformancePackResult.Companion.toKotlin as getConformancePackResultToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.GetOrganizationConformancePackResult.Companion.toKotlin as getOrganizationConformancePackResultToKotlin
import com.pulumi.awsnative.configuration.kotlin.outputs.GetStoredQueryResult.Companion.toKotlin as getStoredQueryResultToKotlin
public object ConfigurationFunctions {
/**
* Resource Type definition for AWS::Config::AggregationAuthorization
* @param argument null
* @return null
*/
public suspend fun getAggregationAuthorization(argument: GetAggregationAuthorizationPlainArgs): GetAggregationAuthorizationResult =
getAggregationAuthorizationResultToKotlin(getAggregationAuthorizationPlain(argument.toJava()).await())
/**
* @see [getAggregationAuthorization].
* @param authorizedAccountId The 12-digit account ID of the account authorized to aggregate data.
* @param authorizedAwsRegion The region authorized to collect aggregated data.
* @return null
*/
public suspend fun getAggregationAuthorization(
authorizedAccountId: String,
authorizedAwsRegion: String,
): GetAggregationAuthorizationResult {
val argument = GetAggregationAuthorizationPlainArgs(
authorizedAccountId = authorizedAccountId,
authorizedAwsRegion = authorizedAwsRegion,
)
return getAggregationAuthorizationResultToKotlin(getAggregationAuthorizationPlain(argument.toJava()).await())
}
/**
* @see [getAggregationAuthorization].
* @param argument Builder for [com.pulumi.awsnative.configuration.kotlin.inputs.GetAggregationAuthorizationPlainArgs].
* @return null
*/
public suspend fun getAggregationAuthorization(argument: suspend GetAggregationAuthorizationPlainArgsBuilder.() -> Unit): GetAggregationAuthorizationResult {
val builder = GetAggregationAuthorizationPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAggregationAuthorizationResultToKotlin(getAggregationAuthorizationPlain(builtArgument.toJava()).await())
}
/**
* You must first create and start the CC configuration recorder in order to create CC managed rules with CFNlong. For more information, see [Managing the Configuration Recorder](https://docs.aws.amazon.com/config/latest/developerguide/stop-start-recorder.html).
* Adds or updates an CC rule to evaluate if your AWS resources comply with your desired configurations. For information on how many CC rules you can have per account, see [Service Limits](https://docs.aws.amazon.com/config/latest/developerguide/configlimits.html) in the *Developer Guide*.
* There are two types of rules: *Managed Rules* and *Custom Rules*. You can use the ``ConfigRule`` resource to create both CC Managed Rules and CC Custom Rules.
* CC Managed Rules are predefined, customizable rules created by CC. For a list of managed rules, see [List of Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/managed-rules-by-aws-config.html). If you are adding an CC managed rule, you must specify the rule's identifier for the ``SourceIdentifier`` key.
* CC Custom Rules are rules that you create from scratch. There are two ways to create CC custom rules: with Lambda functions ([Developer Guide](https://docs.aws.amazon.com/config/latest/developerguide/gettingstarted-concepts.html#gettingstarted-concepts-function)) and with CFNGUARDshort ([Guard GitHub Repository](https://docs.aws.amazon.com/https://github.com/aws-cloudformation/cloudformation-guard)), a policy-as-code language. CC custom rules created with LAMlong are called *Custom Lambda Rules* and CC custom rules created with CFNGUARDshort are called *Custom Policy Rules*.
* If you are adding a new CC Custom LAM rule, you first need to create an LAMlong function that the rule invokes to evaluate your resources. When you use the ``ConfigRule`` resource to add a Custom LAM rule to CC, you must specify the Amazon Resource Name (ARN) that LAMlong assigns to the function. You specify the ARN in the ``SourceIdentifier`` key. This key is part of the ``Source`` object, which is part of the ``ConfigRule`` object.
* For any new CC rule that you add, specify the ``ConfigRuleName`` in the ``ConfigRule`` object. Do not specify the ``ConfigRuleArn`` or the ``ConfigRuleId``. These values are generated by CC for new rules.
* If you are updating a rule that you added previously, you can specify the rule by ``ConfigRuleName``, ``ConfigRuleId``, or ``ConfigRuleArn`` in the ``ConfigRule`` data type that you use in this request.
* For more information about developing and using CC rules, see [Evaluating Resources with Rules](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config.html) in the *Developer Guide*.
* @param argument null
* @return null
*/
public suspend fun getConfigRule(argument: GetConfigRulePlainArgs): GetConfigRuleResult =
getConfigRuleResultToKotlin(getConfigRulePlain(argument.toJava()).await())
/**
* @see [getConfigRule].
* @param configRuleName A name for the CC rule. If you don't specify a name, CFN generates a unique physical ID and uses that ID for the rule name. For more information, see [Name Type](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-name.html).
* @return null
*/
public suspend fun getConfigRule(configRuleName: String): GetConfigRuleResult {
val argument = GetConfigRulePlainArgs(
configRuleName = configRuleName,
)
return getConfigRuleResultToKotlin(getConfigRulePlain(argument.toJava()).await())
}
/**
* @see [getConfigRule].
* @param argument Builder for [com.pulumi.awsnative.configuration.kotlin.inputs.GetConfigRulePlainArgs].
* @return null
*/
public suspend fun getConfigRule(argument: suspend GetConfigRulePlainArgsBuilder.() -> Unit): GetConfigRuleResult {
val builder = GetConfigRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getConfigRuleResultToKotlin(getConfigRulePlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::Config::ConfigurationAggregator
* @param argument null
* @return null
*/
public suspend fun getConfigurationAggregator(argument: GetConfigurationAggregatorPlainArgs): GetConfigurationAggregatorResult =
getConfigurationAggregatorResultToKotlin(getConfigurationAggregatorPlain(argument.toJava()).await())
/**
* @see [getConfigurationAggregator].
* @param configurationAggregatorName The name of the aggregator.
* @return null
*/
public suspend fun getConfigurationAggregator(configurationAggregatorName: String): GetConfigurationAggregatorResult {
val argument = GetConfigurationAggregatorPlainArgs(
configurationAggregatorName = configurationAggregatorName,
)
return getConfigurationAggregatorResultToKotlin(getConfigurationAggregatorPlain(argument.toJava()).await())
}
/**
* @see [getConfigurationAggregator].
* @param argument Builder for [com.pulumi.awsnative.configuration.kotlin.inputs.GetConfigurationAggregatorPlainArgs].
* @return null
*/
public suspend fun getConfigurationAggregator(argument: suspend GetConfigurationAggregatorPlainArgsBuilder.() -> Unit): GetConfigurationAggregatorResult {
val builder = GetConfigurationAggregatorPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getConfigurationAggregatorResultToKotlin(getConfigurationAggregatorPlain(builtArgument.toJava()).await())
}
/**
* A conformance pack is a collection of AWS Config rules and remediation actions that can be easily deployed as a single entity in an account and a region or across an entire AWS Organization.
* @param argument null
* @return null
*/
public suspend fun getConformancePack(argument: GetConformancePackPlainArgs): GetConformancePackResult =
getConformancePackResultToKotlin(getConformancePackPlain(argument.toJava()).await())
/**
* @see [getConformancePack].
* @param conformancePackName Name of the conformance pack which will be assigned as the unique identifier.
* @return null
*/
public suspend fun getConformancePack(conformancePackName: String): GetConformancePackResult {
val argument = GetConformancePackPlainArgs(
conformancePackName = conformancePackName,
)
return getConformancePackResultToKotlin(getConformancePackPlain(argument.toJava()).await())
}
/**
* @see [getConformancePack].
* @param argument Builder for [com.pulumi.awsnative.configuration.kotlin.inputs.GetConformancePackPlainArgs].
* @return null
*/
public suspend fun getConformancePack(argument: suspend GetConformancePackPlainArgsBuilder.() -> Unit): GetConformancePackResult {
val builder = GetConformancePackPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getConformancePackResultToKotlin(getConformancePackPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::Config::OrganizationConformancePack.
* @param argument null
* @return null
*/
public suspend fun getOrganizationConformancePack(argument: GetOrganizationConformancePackPlainArgs): GetOrganizationConformancePackResult =
getOrganizationConformancePackResultToKotlin(getOrganizationConformancePackPlain(argument.toJava()).await())
/**
* @see [getOrganizationConformancePack].
* @param organizationConformancePackName The name of the organization conformance pack.
* @return null
*/
public suspend fun getOrganizationConformancePack(organizationConformancePackName: String): GetOrganizationConformancePackResult {
val argument = GetOrganizationConformancePackPlainArgs(
organizationConformancePackName = organizationConformancePackName,
)
return getOrganizationConformancePackResultToKotlin(getOrganizationConformancePackPlain(argument.toJava()).await())
}
/**
* @see [getOrganizationConformancePack].
* @param argument Builder for [com.pulumi.awsnative.configuration.kotlin.inputs.GetOrganizationConformancePackPlainArgs].
* @return null
*/
public suspend fun getOrganizationConformancePack(argument: suspend GetOrganizationConformancePackPlainArgsBuilder.() -> Unit): GetOrganizationConformancePackResult {
val builder = GetOrganizationConformancePackPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getOrganizationConformancePackResultToKotlin(getOrganizationConformancePackPlain(builtArgument.toJava()).await())
}
/**
* Resource Type definition for AWS::Config::StoredQuery
* @param argument null
* @return null
*/
public suspend fun getStoredQuery(argument: GetStoredQueryPlainArgs): GetStoredQueryResult =
getStoredQueryResultToKotlin(getStoredQueryPlain(argument.toJava()).await())
/**
* @see [getStoredQuery].
* @param queryName The name of the query.
* @return null
*/
public suspend fun getStoredQuery(queryName: String): GetStoredQueryResult {
val argument = GetStoredQueryPlainArgs(
queryName = queryName,
)
return getStoredQueryResultToKotlin(getStoredQueryPlain(argument.toJava()).await())
}
/**
* @see [getStoredQuery].
* @param argument Builder for [com.pulumi.awsnative.configuration.kotlin.inputs.GetStoredQueryPlainArgs].
* @return null
*/
public suspend fun getStoredQuery(argument: suspend GetStoredQueryPlainArgsBuilder.() -> Unit): GetStoredQueryResult {
val builder = GetStoredQueryPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStoredQueryResultToKotlin(getStoredQueryPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy