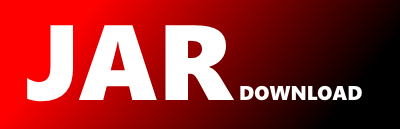
com.pulumi.awsnative.connect.kotlin.RoutingProfileArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.connect.kotlin
import com.pulumi.awsnative.connect.RoutingProfileArgs.builder
import com.pulumi.awsnative.connect.kotlin.enums.RoutingProfileAgentAvailabilityTimer
import com.pulumi.awsnative.connect.kotlin.inputs.RoutingProfileMediaConcurrencyArgs
import com.pulumi.awsnative.connect.kotlin.inputs.RoutingProfileMediaConcurrencyArgsBuilder
import com.pulumi.awsnative.connect.kotlin.inputs.RoutingProfileQueueConfigArgs
import com.pulumi.awsnative.connect.kotlin.inputs.RoutingProfileQueueConfigArgsBuilder
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::Connect::RoutingProfile
* @property agentAvailabilityTimer Whether agents with this routing profile will have their routing order calculated based on longest idle time or time since their last inbound contact.
* @property defaultOutboundQueueArn The identifier of the default outbound queue for this routing profile.
* @property description The description of the routing profile.
* @property instanceArn The identifier of the Amazon Connect instance.
* @property mediaConcurrencies The channels agents can handle in the Contact Control Panel (CCP) for this routing profile.
* @property name The name of the routing profile.
* @property queueConfigs The queues to associate with this routing profile.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class RoutingProfileArgs(
public val agentAvailabilityTimer: Output? = null,
public val defaultOutboundQueueArn: Output? = null,
public val description: Output? = null,
public val instanceArn: Output? = null,
public val mediaConcurrencies: Output>? = null,
public val name: Output? = null,
public val queueConfigs: Output>? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.connect.RoutingProfileArgs =
com.pulumi.awsnative.connect.RoutingProfileArgs.builder()
.agentAvailabilityTimer(
agentAvailabilityTimer?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.defaultOutboundQueueArn(defaultOutboundQueueArn?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.instanceArn(instanceArn?.applyValue({ args0 -> args0 }))
.mediaConcurrencies(
mediaConcurrencies?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.queueConfigs(
queueConfigs?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [RoutingProfileArgs].
*/
@PulumiTagMarker
public class RoutingProfileArgsBuilder internal constructor() {
private var agentAvailabilityTimer: Output? = null
private var defaultOutboundQueueArn: Output? = null
private var description: Output? = null
private var instanceArn: Output? = null
private var mediaConcurrencies: Output>? = null
private var name: Output? = null
private var queueConfigs: Output>? = null
private var tags: Output>? = null
/**
* @param value Whether agents with this routing profile will have their routing order calculated based on longest idle time or time since their last inbound contact.
*/
@JvmName("yrqwnajjgpnjibed")
public suspend fun agentAvailabilityTimer(`value`: Output) {
this.agentAvailabilityTimer = value
}
/**
* @param value The identifier of the default outbound queue for this routing profile.
*/
@JvmName("atobndqkqjqucdbj")
public suspend fun defaultOutboundQueueArn(`value`: Output) {
this.defaultOutboundQueueArn = value
}
/**
* @param value The description of the routing profile.
*/
@JvmName("lnqpkdxiykaftpou")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The identifier of the Amazon Connect instance.
*/
@JvmName("lufuemrvkcmkfaor")
public suspend fun instanceArn(`value`: Output) {
this.instanceArn = value
}
/**
* @param value The channels agents can handle in the Contact Control Panel (CCP) for this routing profile.
*/
@JvmName("pvnkjudxkyjnlmtv")
public suspend fun mediaConcurrencies(`value`: Output>) {
this.mediaConcurrencies = value
}
@JvmName("qdtsbowuapfbobyw")
public suspend fun mediaConcurrencies(vararg values: Output) {
this.mediaConcurrencies = Output.all(values.asList())
}
/**
* @param values The channels agents can handle in the Contact Control Panel (CCP) for this routing profile.
*/
@JvmName("pbwtlylucghwosrv")
public suspend fun mediaConcurrencies(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy