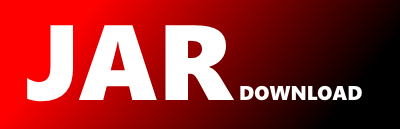
com.pulumi.awsnative.connect.kotlin.inputs.UserIdentityInfoArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.connect.kotlin.inputs
import com.pulumi.awsnative.connect.inputs.UserIdentityInfoArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Contains information about the identity of a user.
* @property email The email address. If you are using SAML for identity management and include this parameter, an error is returned.
* @property firstName The first name. This is required if you are using Amazon Connect or SAML for identity management.
* @property lastName The last name. This is required if you are using Amazon Connect or SAML for identity management.
* @property mobile The user's mobile number.
* @property secondaryEmail The user's secondary email address. If you provide a secondary email, the user receives email notifications -- other than password reset notifications -- to this email address instead of to their primary email address.
* *Pattern* : `(?=^.{0,265}$)[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,63}`
*/
public data class UserIdentityInfoArgs(
public val email: Output? = null,
public val firstName: Output? = null,
public val lastName: Output? = null,
public val mobile: Output? = null,
public val secondaryEmail: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.connect.inputs.UserIdentityInfoArgs =
com.pulumi.awsnative.connect.inputs.UserIdentityInfoArgs.builder()
.email(email?.applyValue({ args0 -> args0 }))
.firstName(firstName?.applyValue({ args0 -> args0 }))
.lastName(lastName?.applyValue({ args0 -> args0 }))
.mobile(mobile?.applyValue({ args0 -> args0 }))
.secondaryEmail(secondaryEmail?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UserIdentityInfoArgs].
*/
@PulumiTagMarker
public class UserIdentityInfoArgsBuilder internal constructor() {
private var email: Output? = null
private var firstName: Output? = null
private var lastName: Output? = null
private var mobile: Output? = null
private var secondaryEmail: Output? = null
/**
* @param value The email address. If you are using SAML for identity management and include this parameter, an error is returned.
*/
@JvmName("oodhnxupdqvbogas")
public suspend fun email(`value`: Output) {
this.email = value
}
/**
* @param value The first name. This is required if you are using Amazon Connect or SAML for identity management.
*/
@JvmName("wsvyghpqobpvuwvd")
public suspend fun firstName(`value`: Output) {
this.firstName = value
}
/**
* @param value The last name. This is required if you are using Amazon Connect or SAML for identity management.
*/
@JvmName("angtptrlscvtbqtp")
public suspend fun lastName(`value`: Output) {
this.lastName = value
}
/**
* @param value The user's mobile number.
*/
@JvmName("xxwcrbkgdcvbkqfe")
public suspend fun mobile(`value`: Output) {
this.mobile = value
}
/**
* @param value The user's secondary email address. If you provide a secondary email, the user receives email notifications -- other than password reset notifications -- to this email address instead of to their primary email address.
* *Pattern* : `(?=^.{0,265}$)[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,63}`
*/
@JvmName("shkpwrnncghlyaej")
public suspend fun secondaryEmail(`value`: Output) {
this.secondaryEmail = value
}
/**
* @param value The email address. If you are using SAML for identity management and include this parameter, an error is returned.
*/
@JvmName("mtqlkfdfcyxugrvi")
public suspend fun email(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.email = mapped
}
/**
* @param value The first name. This is required if you are using Amazon Connect or SAML for identity management.
*/
@JvmName("mqlobygisvifbsht")
public suspend fun firstName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.firstName = mapped
}
/**
* @param value The last name. This is required if you are using Amazon Connect or SAML for identity management.
*/
@JvmName("qkmfoqkctphkficx")
public suspend fun lastName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastName = mapped
}
/**
* @param value The user's mobile number.
*/
@JvmName("gqsnxeytwdvhjkim")
public suspend fun mobile(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mobile = mapped
}
/**
* @param value The user's secondary email address. If you provide a secondary email, the user receives email notifications -- other than password reset notifications -- to this email address instead of to their primary email address.
* *Pattern* : `(?=^.{0,265}$)[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,63}`
*/
@JvmName("isvqmahvjfovwloc")
public suspend fun secondaryEmail(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryEmail = mapped
}
internal fun build(): UserIdentityInfoArgs = UserIdentityInfoArgs(
email = email,
firstName = firstName,
lastName = lastName,
mobile = mobile,
secondaryEmail = secondaryEmail,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy