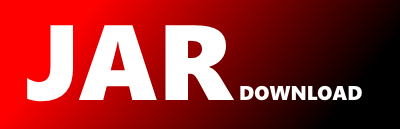
com.pulumi.awsnative.customerprofiles.kotlin.inputs.IntegrationSourceConnectorPropertiesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.customerprofiles.kotlin.inputs
import com.pulumi.awsnative.customerprofiles.inputs.IntegrationSourceConnectorPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property marketo The properties that are applied when Marketo is being used as a source.
* @property s3 The properties that are applied when Amazon S3 is being used as the flow source.
* @property salesforce The properties that are applied when Salesforce is being used as a source.
* @property serviceNow The properties that are applied when ServiceNow is being used as a source.
* @property zendesk The properties that are applied when using Zendesk as a flow source.
*/
public data class IntegrationSourceConnectorPropertiesArgs(
public val marketo: Output? = null,
public val s3: Output? = null,
public val salesforce: Output? = null,
public val serviceNow: Output? = null,
public val zendesk: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.customerprofiles.inputs.IntegrationSourceConnectorPropertiesArgs =
com.pulumi.awsnative.customerprofiles.inputs.IntegrationSourceConnectorPropertiesArgs.builder()
.marketo(marketo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.s3(s3?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.salesforce(salesforce?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.serviceNow(serviceNow?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.zendesk(zendesk?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [IntegrationSourceConnectorPropertiesArgs].
*/
@PulumiTagMarker
public class IntegrationSourceConnectorPropertiesArgsBuilder internal constructor() {
private var marketo: Output? = null
private var s3: Output? = null
private var salesforce: Output? = null
private var serviceNow: Output? = null
private var zendesk: Output? = null
/**
* @param value The properties that are applied when Marketo is being used as a source.
*/
@JvmName("biufwodrhxyrsxuk")
public suspend fun marketo(`value`: Output) {
this.marketo = value
}
/**
* @param value The properties that are applied when Amazon S3 is being used as the flow source.
*/
@JvmName("ibbfhhrjxkikctri")
public suspend fun s3(`value`: Output) {
this.s3 = value
}
/**
* @param value The properties that are applied when Salesforce is being used as a source.
*/
@JvmName("nglkxudipiooflbd")
public suspend fun salesforce(`value`: Output) {
this.salesforce = value
}
/**
* @param value The properties that are applied when ServiceNow is being used as a source.
*/
@JvmName("rnqvvjgocyxktraw")
public suspend fun serviceNow(`value`: Output) {
this.serviceNow = value
}
/**
* @param value The properties that are applied when using Zendesk as a flow source.
*/
@JvmName("rrrhvleqqygwaolh")
public suspend fun zendesk(`value`: Output) {
this.zendesk = value
}
/**
* @param value The properties that are applied when Marketo is being used as a source.
*/
@JvmName("xyjalneexsgkfbxc")
public suspend fun marketo(`value`: IntegrationMarketoSourcePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.marketo = mapped
}
/**
* @param argument The properties that are applied when Marketo is being used as a source.
*/
@JvmName("rplbrixciexrimaa")
public suspend fun marketo(argument: suspend IntegrationMarketoSourcePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = IntegrationMarketoSourcePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.marketo = mapped
}
/**
* @param value The properties that are applied when Amazon S3 is being used as the flow source.
*/
@JvmName("ivydlsvjsfndyxaw")
public suspend fun s3(`value`: IntegrationS3SourcePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3 = mapped
}
/**
* @param argument The properties that are applied when Amazon S3 is being used as the flow source.
*/
@JvmName("jmhukgsiwenobbwb")
public suspend fun s3(argument: suspend IntegrationS3SourcePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = IntegrationS3SourcePropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.s3 = mapped
}
/**
* @param value The properties that are applied when Salesforce is being used as a source.
*/
@JvmName("nqfevuffjnjhijku")
public suspend fun salesforce(`value`: IntegrationSalesforceSourcePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.salesforce = mapped
}
/**
* @param argument The properties that are applied when Salesforce is being used as a source.
*/
@JvmName("hxbabegmwhwjtmgk")
public suspend fun salesforce(argument: suspend IntegrationSalesforceSourcePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = IntegrationSalesforceSourcePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.salesforce = mapped
}
/**
* @param value The properties that are applied when ServiceNow is being used as a source.
*/
@JvmName("xgsbjducocmvytme")
public suspend fun serviceNow(`value`: IntegrationServiceNowSourcePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceNow = mapped
}
/**
* @param argument The properties that are applied when ServiceNow is being used as a source.
*/
@JvmName("snhohjiukdxcaqmk")
public suspend fun serviceNow(argument: suspend IntegrationServiceNowSourcePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = IntegrationServiceNowSourcePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.serviceNow = mapped
}
/**
* @param value The properties that are applied when using Zendesk as a flow source.
*/
@JvmName("gvjwnlphrnrwbrfs")
public suspend fun zendesk(`value`: IntegrationZendeskSourcePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zendesk = mapped
}
/**
* @param argument The properties that are applied when using Zendesk as a flow source.
*/
@JvmName("eymbeiwdmqxvegcp")
public suspend fun zendesk(argument: suspend IntegrationZendeskSourcePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = IntegrationZendeskSourcePropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.zendesk = mapped
}
internal fun build(): IntegrationSourceConnectorPropertiesArgs =
IntegrationSourceConnectorPropertiesArgs(
marketo = marketo,
s3 = s3,
salesforce = salesforce,
serviceNow = serviceNow,
zendesk = zendesk,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy