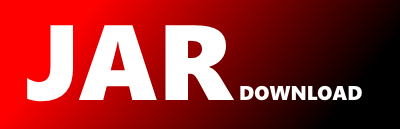
com.pulumi.awsnative.databrew.kotlin.inputs.JobOutputArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.databrew.kotlin.inputs
import com.pulumi.awsnative.databrew.inputs.JobOutputArgs.builder
import com.pulumi.awsnative.databrew.kotlin.enums.JobOutputCompressionFormat
import com.pulumi.awsnative.databrew.kotlin.enums.JobOutputFormat
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property compressionFormat The compression algorithm used to compress the output text of the job.
* @property format The data format of the output of the job.
* @property formatOptions Represents options that define how DataBrew formats job output files.
* @property location The location in Amazon S3 where the job writes its output.
* @property maxOutputFiles The maximum number of files to be generated by the job and written to the output folder.
* @property overwrite A value that, if true, means that any data in the location specified for output is overwritten with new output.
* @property partitionColumns The names of one or more partition columns for the output of the job.
*/
public data class JobOutputArgs(
public val compressionFormat: Output? = null,
public val format: Output? = null,
public val formatOptions: Output? = null,
public val location: Output,
public val maxOutputFiles: Output? = null,
public val overwrite: Output? = null,
public val partitionColumns: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.databrew.inputs.JobOutputArgs =
com.pulumi.awsnative.databrew.inputs.JobOutputArgs.builder()
.compressionFormat(compressionFormat?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.format(format?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.formatOptions(formatOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.location(location.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maxOutputFiles(maxOutputFiles?.applyValue({ args0 -> args0 }))
.overwrite(overwrite?.applyValue({ args0 -> args0 }))
.partitionColumns(partitionColumns?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [JobOutputArgs].
*/
@PulumiTagMarker
public class JobOutputArgsBuilder internal constructor() {
private var compressionFormat: Output? = null
private var format: Output? = null
private var formatOptions: Output? = null
private var location: Output? = null
private var maxOutputFiles: Output? = null
private var overwrite: Output? = null
private var partitionColumns: Output>? = null
/**
* @param value The compression algorithm used to compress the output text of the job.
*/
@JvmName("kacdjmqjsuvfggsk")
public suspend fun compressionFormat(`value`: Output) {
this.compressionFormat = value
}
/**
* @param value The data format of the output of the job.
*/
@JvmName("ehoxbtlrribdywnf")
public suspend fun format(`value`: Output) {
this.format = value
}
/**
* @param value Represents options that define how DataBrew formats job output files.
*/
@JvmName("huwwricjrgdyycem")
public suspend fun formatOptions(`value`: Output) {
this.formatOptions = value
}
/**
* @param value The location in Amazon S3 where the job writes its output.
*/
@JvmName("twmpeoksktfcfqqk")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The maximum number of files to be generated by the job and written to the output folder.
*/
@JvmName("xtyxtpbytknlinly")
public suspend fun maxOutputFiles(`value`: Output) {
this.maxOutputFiles = value
}
/**
* @param value A value that, if true, means that any data in the location specified for output is overwritten with new output.
*/
@JvmName("lcaballaopkatppv")
public suspend fun overwrite(`value`: Output) {
this.overwrite = value
}
/**
* @param value The names of one or more partition columns for the output of the job.
*/
@JvmName("dkfdeghcridpabmg")
public suspend fun partitionColumns(`value`: Output>) {
this.partitionColumns = value
}
@JvmName("assjkvxvspkjfnjw")
public suspend fun partitionColumns(vararg values: Output) {
this.partitionColumns = Output.all(values.asList())
}
/**
* @param values The names of one or more partition columns for the output of the job.
*/
@JvmName("pfrujpbklmgnrktg")
public suspend fun partitionColumns(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy