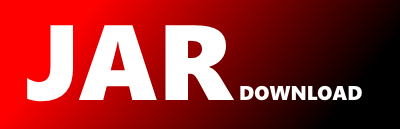
com.pulumi.awsnative.databrew.kotlin.outputs.RecipeParameters.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.databrew.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property aggregateFunction
* @property base
* @property caseStatement
* @property categoryMap
* @property charsToRemove
* @property collapseConsecutiveWhitespace
* @property columnDataType
* @property columnRange
* @property count
* @property customCharacters
* @property customStopWords
* @property customValue
* @property datasetsColumns
* @property dateAddValue
* @property dateTimeFormat
* @property dateTimeParameters
* @property deleteOtherRows
* @property delimiter
* @property endPattern
* @property endPosition
* @property endValue
* @property expandContractions
* @property exponent
* @property falseString
* @property groupByAggFunctionOptions
* @property groupByColumns
* @property hiddenColumns
* @property ignoreCase
* @property includeInSplit
* @property input Input
* @property interval
* @property isText
* @property joinKeys
* @property joinType
* @property leftColumns
* @property limit
* @property lowerBound
* @property mapType
* @property modeType
* @property multiLine
* @property numRows
* @property numRowsAfter
* @property numRowsBefore
* @property orderByColumn
* @property orderByColumns
* @property other
* @property pattern
* @property patternOption1
* @property patternOption2
* @property patternOptions
* @property period
* @property position
* @property removeAllPunctuation
* @property removeAllQuotes
* @property removeAllWhitespace
* @property removeCustomCharacters
* @property removeCustomValue
* @property removeLeadingAndTrailingPunctuation
* @property removeLeadingAndTrailingQuotes
* @property removeLeadingAndTrailingWhitespace
* @property removeLetters
* @property removeNumbers
* @property removeSourceColumn
* @property removeSpecialCharacters
* @property rightColumns
* @property sampleSize
* @property sampleType
* @property secondInput
* @property secondaryInputs
* @property sheetIndexes
* @property sheetNames
* @property sourceColumn
* @property sourceColumn1
* @property sourceColumn2
* @property sourceColumns
* @property startColumnIndex
* @property startPattern
* @property startPosition
* @property startValue
* @property stemmingMode
* @property stepCount
* @property stepIndex
* @property stopWordsMode
* @property strategy
* @property targetColumn
* @property targetColumnNames
* @property targetDateFormat
* @property targetIndex
* @property timeZone
* @property tokenizerPattern
* @property trueString
* @property udfLang
* @property units
* @property unpivotColumn
* @property upperBound
* @property useNewDataFrame
* @property value
* @property value1
* @property value2
* @property valueColumn
* @property viewFrame
*/
public data class RecipeParameters(
public val aggregateFunction: String? = null,
public val base: String? = null,
public val caseStatement: String? = null,
public val categoryMap: String? = null,
public val charsToRemove: String? = null,
public val collapseConsecutiveWhitespace: String? = null,
public val columnDataType: String? = null,
public val columnRange: String? = null,
public val count: String? = null,
public val customCharacters: String? = null,
public val customStopWords: String? = null,
public val customValue: String? = null,
public val datasetsColumns: String? = null,
public val dateAddValue: String? = null,
public val dateTimeFormat: String? = null,
public val dateTimeParameters: String? = null,
public val deleteOtherRows: String? = null,
public val delimiter: String? = null,
public val endPattern: String? = null,
public val endPosition: String? = null,
public val endValue: String? = null,
public val expandContractions: String? = null,
public val exponent: String? = null,
public val falseString: String? = null,
public val groupByAggFunctionOptions: String? = null,
public val groupByColumns: String? = null,
public val hiddenColumns: String? = null,
public val ignoreCase: String? = null,
public val includeInSplit: String? = null,
public val input: RecipeParametersInputProperties? = null,
public val interval: String? = null,
public val isText: String? = null,
public val joinKeys: String? = null,
public val joinType: String? = null,
public val leftColumns: String? = null,
public val limit: String? = null,
public val lowerBound: String? = null,
public val mapType: String? = null,
public val modeType: String? = null,
public val multiLine: Boolean? = null,
public val numRows: String? = null,
public val numRowsAfter: String? = null,
public val numRowsBefore: String? = null,
public val orderByColumn: String? = null,
public val orderByColumns: String? = null,
public val other: String? = null,
public val pattern: String? = null,
public val patternOption1: String? = null,
public val patternOption2: String? = null,
public val patternOptions: String? = null,
public val period: String? = null,
public val position: String? = null,
public val removeAllPunctuation: String? = null,
public val removeAllQuotes: String? = null,
public val removeAllWhitespace: String? = null,
public val removeCustomCharacters: String? = null,
public val removeCustomValue: String? = null,
public val removeLeadingAndTrailingPunctuation: String? = null,
public val removeLeadingAndTrailingQuotes: String? = null,
public val removeLeadingAndTrailingWhitespace: String? = null,
public val removeLetters: String? = null,
public val removeNumbers: String? = null,
public val removeSourceColumn: String? = null,
public val removeSpecialCharacters: String? = null,
public val rightColumns: String? = null,
public val sampleSize: String? = null,
public val sampleType: String? = null,
public val secondInput: String? = null,
public val secondaryInputs: List? = null,
public val sheetIndexes: List? = null,
public val sheetNames: List? = null,
public val sourceColumn: String? = null,
public val sourceColumn1: String? = null,
public val sourceColumn2: String? = null,
public val sourceColumns: String? = null,
public val startColumnIndex: String? = null,
public val startPattern: String? = null,
public val startPosition: String? = null,
public val startValue: String? = null,
public val stemmingMode: String? = null,
public val stepCount: String? = null,
public val stepIndex: String? = null,
public val stopWordsMode: String? = null,
public val strategy: String? = null,
public val targetColumn: String? = null,
public val targetColumnNames: String? = null,
public val targetDateFormat: String? = null,
public val targetIndex: String? = null,
public val timeZone: String? = null,
public val tokenizerPattern: String? = null,
public val trueString: String? = null,
public val udfLang: String? = null,
public val units: String? = null,
public val unpivotColumn: String? = null,
public val upperBound: String? = null,
public val useNewDataFrame: String? = null,
public val `value`: String? = null,
public val value1: String? = null,
public val value2: String? = null,
public val valueColumn: String? = null,
public val viewFrame: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.databrew.outputs.RecipeParameters): RecipeParameters = RecipeParameters(
aggregateFunction = javaType.aggregateFunction().map({ args0 -> args0 }).orElse(null),
base = javaType.base().map({ args0 -> args0 }).orElse(null),
caseStatement = javaType.caseStatement().map({ args0 -> args0 }).orElse(null),
categoryMap = javaType.categoryMap().map({ args0 -> args0 }).orElse(null),
charsToRemove = javaType.charsToRemove().map({ args0 -> args0 }).orElse(null),
collapseConsecutiveWhitespace = javaType.collapseConsecutiveWhitespace().map({ args0 ->
args0
}).orElse(null),
columnDataType = javaType.columnDataType().map({ args0 -> args0 }).orElse(null),
columnRange = javaType.columnRange().map({ args0 -> args0 }).orElse(null),
count = javaType.count().map({ args0 -> args0 }).orElse(null),
customCharacters = javaType.customCharacters().map({ args0 -> args0 }).orElse(null),
customStopWords = javaType.customStopWords().map({ args0 -> args0 }).orElse(null),
customValue = javaType.customValue().map({ args0 -> args0 }).orElse(null),
datasetsColumns = javaType.datasetsColumns().map({ args0 -> args0 }).orElse(null),
dateAddValue = javaType.dateAddValue().map({ args0 -> args0 }).orElse(null),
dateTimeFormat = javaType.dateTimeFormat().map({ args0 -> args0 }).orElse(null),
dateTimeParameters = javaType.dateTimeParameters().map({ args0 -> args0 }).orElse(null),
deleteOtherRows = javaType.deleteOtherRows().map({ args0 -> args0 }).orElse(null),
delimiter = javaType.delimiter().map({ args0 -> args0 }).orElse(null),
endPattern = javaType.endPattern().map({ args0 -> args0 }).orElse(null),
endPosition = javaType.endPosition().map({ args0 -> args0 }).orElse(null),
endValue = javaType.endValue().map({ args0 -> args0 }).orElse(null),
expandContractions = javaType.expandContractions().map({ args0 -> args0 }).orElse(null),
exponent = javaType.exponent().map({ args0 -> args0 }).orElse(null),
falseString = javaType.falseString().map({ args0 -> args0 }).orElse(null),
groupByAggFunctionOptions = javaType.groupByAggFunctionOptions().map({ args0 ->
args0
}).orElse(null),
groupByColumns = javaType.groupByColumns().map({ args0 -> args0 }).orElse(null),
hiddenColumns = javaType.hiddenColumns().map({ args0 -> args0 }).orElse(null),
ignoreCase = javaType.ignoreCase().map({ args0 -> args0 }).orElse(null),
includeInSplit = javaType.includeInSplit().map({ args0 -> args0 }).orElse(null),
input = javaType.input().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.databrew.kotlin.outputs.RecipeParametersInputProperties.Companion.toKotlin(args0)
})
}).orElse(null),
interval = javaType.interval().map({ args0 -> args0 }).orElse(null),
isText = javaType.isText().map({ args0 -> args0 }).orElse(null),
joinKeys = javaType.joinKeys().map({ args0 -> args0 }).orElse(null),
joinType = javaType.joinType().map({ args0 -> args0 }).orElse(null),
leftColumns = javaType.leftColumns().map({ args0 -> args0 }).orElse(null),
limit = javaType.limit().map({ args0 -> args0 }).orElse(null),
lowerBound = javaType.lowerBound().map({ args0 -> args0 }).orElse(null),
mapType = javaType.mapType().map({ args0 -> args0 }).orElse(null),
modeType = javaType.modeType().map({ args0 -> args0 }).orElse(null),
multiLine = javaType.multiLine().map({ args0 -> args0 }).orElse(null),
numRows = javaType.numRows().map({ args0 -> args0 }).orElse(null),
numRowsAfter = javaType.numRowsAfter().map({ args0 -> args0 }).orElse(null),
numRowsBefore = javaType.numRowsBefore().map({ args0 -> args0 }).orElse(null),
orderByColumn = javaType.orderByColumn().map({ args0 -> args0 }).orElse(null),
orderByColumns = javaType.orderByColumns().map({ args0 -> args0 }).orElse(null),
other = javaType.other().map({ args0 -> args0 }).orElse(null),
pattern = javaType.pattern().map({ args0 -> args0 }).orElse(null),
patternOption1 = javaType.patternOption1().map({ args0 -> args0 }).orElse(null),
patternOption2 = javaType.patternOption2().map({ args0 -> args0 }).orElse(null),
patternOptions = javaType.patternOptions().map({ args0 -> args0 }).orElse(null),
period = javaType.period().map({ args0 -> args0 }).orElse(null),
position = javaType.position().map({ args0 -> args0 }).orElse(null),
removeAllPunctuation = javaType.removeAllPunctuation().map({ args0 -> args0 }).orElse(null),
removeAllQuotes = javaType.removeAllQuotes().map({ args0 -> args0 }).orElse(null),
removeAllWhitespace = javaType.removeAllWhitespace().map({ args0 -> args0 }).orElse(null),
removeCustomCharacters = javaType.removeCustomCharacters().map({ args0 -> args0 }).orElse(null),
removeCustomValue = javaType.removeCustomValue().map({ args0 -> args0 }).orElse(null),
removeLeadingAndTrailingPunctuation = javaType.removeLeadingAndTrailingPunctuation().map({ args0 ->
args0
}).orElse(null),
removeLeadingAndTrailingQuotes = javaType.removeLeadingAndTrailingQuotes().map({ args0 ->
args0
}).orElse(null),
removeLeadingAndTrailingWhitespace = javaType.removeLeadingAndTrailingWhitespace().map({ args0 ->
args0
}).orElse(null),
removeLetters = javaType.removeLetters().map({ args0 -> args0 }).orElse(null),
removeNumbers = javaType.removeNumbers().map({ args0 -> args0 }).orElse(null),
removeSourceColumn = javaType.removeSourceColumn().map({ args0 -> args0 }).orElse(null),
removeSpecialCharacters = javaType.removeSpecialCharacters().map({ args0 -> args0 }).orElse(null),
rightColumns = javaType.rightColumns().map({ args0 -> args0 }).orElse(null),
sampleSize = javaType.sampleSize().map({ args0 -> args0 }).orElse(null),
sampleType = javaType.sampleType().map({ args0 -> args0 }).orElse(null),
secondInput = javaType.secondInput().map({ args0 -> args0 }).orElse(null),
secondaryInputs = javaType.secondaryInputs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.databrew.kotlin.outputs.RecipeSecondaryInput.Companion.toKotlin(args0)
})
}),
sheetIndexes = javaType.sheetIndexes().map({ args0 -> args0 }),
sheetNames = javaType.sheetNames().map({ args0 -> args0 }),
sourceColumn = javaType.sourceColumn().map({ args0 -> args0 }).orElse(null),
sourceColumn1 = javaType.sourceColumn1().map({ args0 -> args0 }).orElse(null),
sourceColumn2 = javaType.sourceColumn2().map({ args0 -> args0 }).orElse(null),
sourceColumns = javaType.sourceColumns().map({ args0 -> args0 }).orElse(null),
startColumnIndex = javaType.startColumnIndex().map({ args0 -> args0 }).orElse(null),
startPattern = javaType.startPattern().map({ args0 -> args0 }).orElse(null),
startPosition = javaType.startPosition().map({ args0 -> args0 }).orElse(null),
startValue = javaType.startValue().map({ args0 -> args0 }).orElse(null),
stemmingMode = javaType.stemmingMode().map({ args0 -> args0 }).orElse(null),
stepCount = javaType.stepCount().map({ args0 -> args0 }).orElse(null),
stepIndex = javaType.stepIndex().map({ args0 -> args0 }).orElse(null),
stopWordsMode = javaType.stopWordsMode().map({ args0 -> args0 }).orElse(null),
strategy = javaType.strategy().map({ args0 -> args0 }).orElse(null),
targetColumn = javaType.targetColumn().map({ args0 -> args0 }).orElse(null),
targetColumnNames = javaType.targetColumnNames().map({ args0 -> args0 }).orElse(null),
targetDateFormat = javaType.targetDateFormat().map({ args0 -> args0 }).orElse(null),
targetIndex = javaType.targetIndex().map({ args0 -> args0 }).orElse(null),
timeZone = javaType.timeZone().map({ args0 -> args0 }).orElse(null),
tokenizerPattern = javaType.tokenizerPattern().map({ args0 -> args0 }).orElse(null),
trueString = javaType.trueString().map({ args0 -> args0 }).orElse(null),
udfLang = javaType.udfLang().map({ args0 -> args0 }).orElse(null),
units = javaType.units().map({ args0 -> args0 }).orElse(null),
unpivotColumn = javaType.unpivotColumn().map({ args0 -> args0 }).orElse(null),
upperBound = javaType.upperBound().map({ args0 -> args0 }).orElse(null),
useNewDataFrame = javaType.useNewDataFrame().map({ args0 -> args0 }).orElse(null),
`value` = javaType.`value`().map({ args0 -> args0 }).orElse(null),
value1 = javaType.value1().map({ args0 -> args0 }).orElse(null),
value2 = javaType.value2().map({ args0 -> args0 }).orElse(null),
valueColumn = javaType.valueColumn().map({ args0 -> args0 }).orElse(null),
viewFrame = javaType.viewFrame().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy